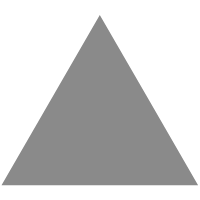
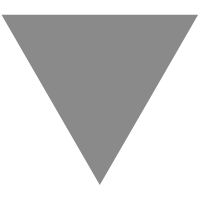
Don't Procrastinate, GO Code!
source link: https://hackernoon.com/dont-procrastinate-go-code
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Don't Procrastinate, GO Code!
@orientsoftwareOrient Software Development Corp.
Orient Software is the leading software company in Vietnam. We provide software solutions to businesses worldwide.
The Go programming language focuses on writing code that is easy to read and elegant in structure and design.
The Go Programming Language is a powerful tool for building infrastructure that can run on almost any platform (from servers to desktops).
It's also straightforward to learn and read. So, if you're just getting started, how do you get your first code lines into the universe? This guide helps you start your software development journey.
What is GO?
Go is an open-source programming language designed for building simple, fast, and reliable software. Google created it in 2007 to help solve developers' programming problems with modern software.
It's a compiled language, which means your code will be transformed into instructions that computers can run through their processors on the fly. This makes it great for projects where you need to get things done fast.
GO is a systems language, which means that it's a bit lower level than languages like Java or Python and more focused on solving problems in ways those languages aren't set up for.
You'll need to manage more of the details of your program since you won't be able to rely on the tools and frameworks they provide. Go's goal is simplicity and efficiency in code, so you don't have to worry about memory allocations or interfacing with external packages or services.
Install GO (Using Homebrew Mac)
The easiest way to get started is by installing GO on your mac. You'll want to install the latest version:
Open a Terminal window (which you can find in Applications > Utilities, or by running the command Spotlight). At the prompt type:
$ brew update
$ brew install golang
Write Your First Code
Now that you have your GO set up let's write some code!
Copy and paste the following example into a new text file called "first.go". Use your favorite code editor like VSCode, Sublime Text, or Atom. Paste in the following:
package main
import "fmt"
func main() {
fmt.Println("Hello World!")
}
Now run it:
$ go run first.go
Hello World!
If you want to compile, run the following command:
$ go build first.go
$ ./first
Hello World!
Hello World Web Server Example
Now you have completed the simple console app; it is time to create a webserver example. First, create a file called "webserver.go":
import (
"fmt"
"net/http"
)
func main() {
http.HandleFunc("/", HelloWorldFunc)
http.ListenAndServe(":8008", nil)
}
func HelloWorldFunc(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello World! %s", r.URL.Path[1:])
}
Now run:
$ go run webserver.go
And navigate to http://localhost:8008/:
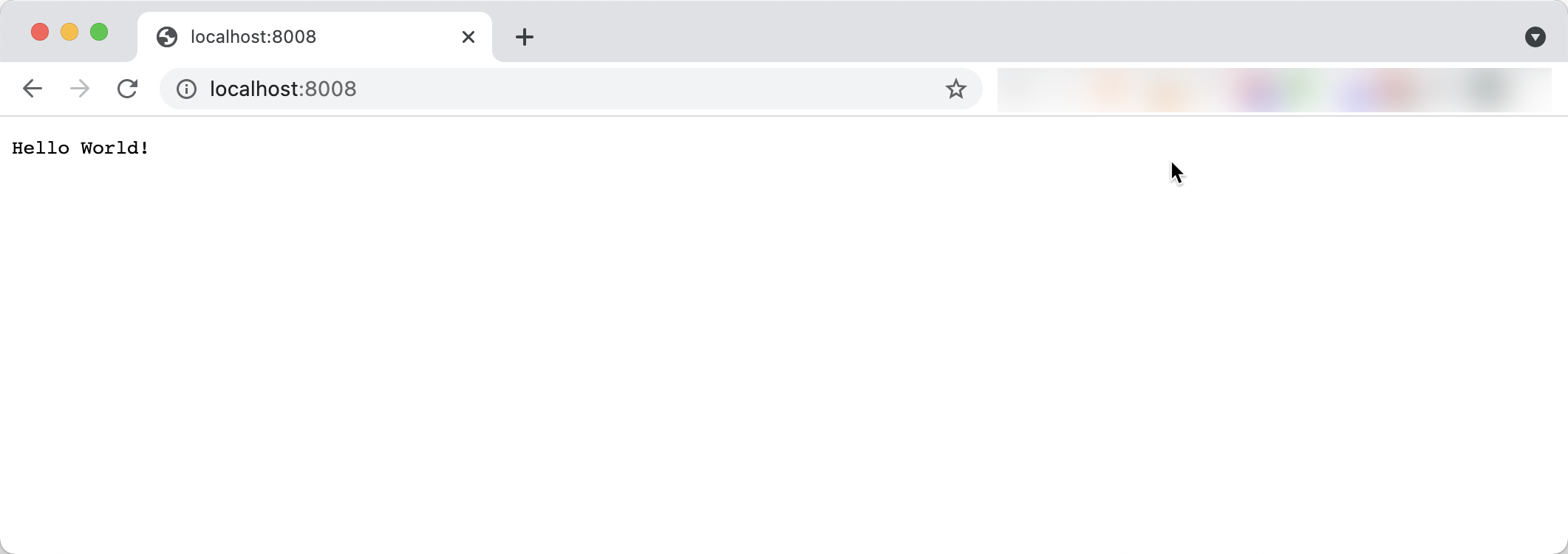
The output of the GO web server application.
Conclusion
Now you have learned how to write some GO code. There are lots of great resources online to help you learn more.
I recommend https://golang.org/doc/ for documentation and the Golang subreddit (https://www.reddit.com/r/golang/) for active community support.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK