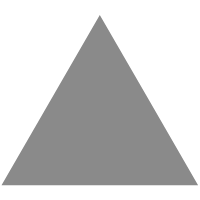
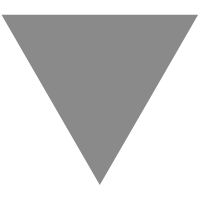
10 个超棒的 JavaScript 简写技巧
source link: https://www.cnblogs.com/frontworld/p/15148751.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
今天我要分享的是10个超棒的JavaScript简写方法,可以加快开发速度,让你的开发工作事半功倍哦。
1. 合并数组
普通写法:
我们通常使用Array
中的concat()
方法合并两个数组。用concat()
方法来合并两个或多个数组,不会更改现有的数组,而是返回一个新的数组。请看一个简单的例子:
let apples = ['🍎', '🍏'];
let fruits = ['🍉', '🍊', '🍇'].concat(apples);
console.log( fruits );
//=> ["🍉", "🍊", "🍇", "🍎", "🍏"]
简写方法:
我们可以通过使用ES6扩展运算符(...
)来减少代码,如下所示:
let apples = ['🍎', '🍏'];
let fruits = ['🍉', '🍊', '🍇', ...apples]; // <-- here
console.log( fruits );
//=> ["🍉", "🍊", "🍇", "🍎", "🍏"]
得到的输出与普通写法相同。
2. 合并数组(在开头位置)
普通写法:
假设我们想将apples
数组中的所有项添加到Fruits
数组的开头,而不是像上一个示例中那样放在末尾。我们可以使用Array.prototype.unshift()
来做到这一点:
let apples = ['🍎', '🍏'];
let fruits = ['🥭', '🍌', '🍒'];
// Add all items from apples onto fruits at start
Array.prototype.unshift.apply(fruits, apples)
console.log( fruits );
//=> ["🍎", "🍏", "🥭", "🍌", "🍒"]
现在红苹果和绿苹果会在开头位置合并而不是末尾。
简写方法:
我们依然可以使用ES6扩展运算符(...
)缩短这段长代码,如下所示:
let apples = ['🍎', '🍏'];
let fruits = [...apples, '🥭', '🍌', '🍒']; // <-- here
console.log( fruits );
//=> ["🍎", "🍏", "🥭", "🍌", "🍒"]
3. 克隆数组
普通写法:
我们可以使用Array
中的slice()
方法轻松克隆数组,如下所示:
let fruits = ['🍉', '🍊', '🍇', '🍎'];
let cloneFruits = fruits.slice();
console.log( cloneFruits );
//=> ["🍉", "🍊", "🍇", "🍎"]
简写方法:
我们可以使用ES6扩展运算符(...
)像这样克隆一个数组:
let fruits = ['🍉', '🍊', '🍇', '🍎'];
let cloneFruits = [...fruits]; // <-- here
console.log( cloneFruits );
//=> ["🍉", "🍊", "🍇", "🍎"]
4. 解构赋值
普通写法:
在处理数组时,我们有时需要将数组“解包”成一堆变量,如下所示:
let apples = ['🍎', '🍏'];
let redApple = apples[0];
let greenApple = apples[1];
console.log( redApple ); //=> 🍎
console.log( greenApple ); //=> 🍏
简写方法:
我们可以通过解构赋值用一行代码实现相同的结果:
let apples = ['🍎', '🍏'];
let [redApple, greenApple] = apples; // <-- here
console.log( redApple ); //=> 🍎
console.log( greenApple ); //=> 🍏
5. 模板字面量
普通写法:
通常,当我们必须向字符串添加表达式时,我们会这样做:
// Display name in between two strings
let name = 'Palash';
console.log('Hello, ' + name + '!');
//=> Hello, Palash!
// Add & Subtract two numbers
let num1 = 20;
let num2 = 10;
console.log('Sum = ' + (num1 + num2) + ' and Subtract = ' + (num1 - num2));
//=> Sum = 30 and Subtract = 10
简写方法:
通过模板字面量,我们可以使用反引号(),这样我们就可以将表达式包装在
${...}`中,然后嵌入到字符串,如下所示:
// Display name in between two strings
let name = 'Palash';
console.log(`Hello, ${name}!`); // <-- No need to use + var + anymore
//=> Hello, Palash!
// Add two numbers
let num1 = 20;
let num2 = 10;
console.log(`Sum = ${num1 + num2} and Subtract = ${num1 - num2}`);
//=> Sum = 30 and Subtract = 10
6. For循环
普通写法:
我们可以使用for
循环像这样循环遍历一个数组:
let fruits = ['🍉', '🍊', '🍇', '🍎'];
// Loop through each fruit
for (let index = 0; index < fruits.length; index++) {
console.log( fruits[index] ); // <-- get the fruit at current index
}
//=> 🍉
//=> 🍊
//=> 🍇
//=> 🍎
简写方法:
我们可以使用for...of
语句实现相同的结果,而代码要少得多,如下所示:
let fruits = ['🍉', '🍊', '🍇', '🍎'];
// Using for...of statement
for (let fruit of fruits) {
console.log( fruit );
}
//=> 🍉
//=> 🍊
//=> 🍇
//=> 🍎
7. 箭头函数
普通写法:
要遍历数组,我们还可以使用Array
中的forEach()
方法。但是需要写很多代码,虽然比最常见的for
循环要少,但仍然比for...of
语句多一点:
let fruits = ['🍉', '🍊', '🍇', '🍎'];
// Using forEach method
fruits.forEach(function(fruit){
console.log( fruit );
});
//=> 🍉
//=> 🍊
//=> 🍇
//=> 🍎
简写方法:
但是使用箭头函数表达式,允许我们用一行编写完整的循环代码,如下所示:
let fruits = ['🍉', '🍊', '🍇', '🍎'];
fruits.forEach(fruit => console.log( fruit )); // <-- Magic ✨
//=> 🍉
//=> 🍊
//=> 🍇
//=> 🍎
大多数时候我使用的是带箭头函数的forEach
循环,这里我把for...of
语句和forEach
循环都展示出来,方便大家根据自己的喜好使用代码。
8. 在数组中查找对象
普通写法:
要通过其中一个属性从对象数组中查找对象的话,我们通常使用for
循环:
let inventory = [
{name: 'Bananas', quantity: 5},
{name: 'Apples', quantity: 10},
{name: 'Grapes', quantity: 2}
];
// Get the object with the name `Apples` inside the array
function getApples(arr, value) {
for (let index = 0; index < arr.length; index++) {
// Check the value of this object property `name` is same as 'Apples'
if (arr[index].name === 'Apples') { //=> 🍎
// A match was found, return this object
return arr[index];
}
}
}
let result = getApples(inventory);
console.log( result )
//=> { name: "Apples", quantity: 10 }
简写方法:
哇!上面我们写了这么多代码来实现这个逻辑。但是使用Array
中的find()
方法和箭头函数=>
,允许我们像这样一行搞定:
// Get the object with the name `Apples` inside the array
function getApples(arr, value) {
return arr.find(obj => obj.name === 'Apples'); // <-- here
}
let result = getApples(inventory);
console.log( result )
//=> { name: "Apples", quantity: 10 }
9. 将字符串转换为整数
普通写法:
parseInt()
函数用于解析字符串并返回整数:
let num = parseInt("10")
console.log( num ) //=> 10
console.log( typeof num ) //=> "number"
简写方法:
我们可以通过在字符串前添加+
前缀来实现相同的结果,如下所示:
let num = +"10";
console.log( num ) //=> 10
console.log( typeof num ) //=> "number"
console.log( +"10" === 10 ) //=> true
10. 短路求值
普通写法:
如果我们必须根据另一个值来设置一个值不是falsy值,一般会使用if-else
语句,就像这样:
function getUserRole(role) {
let userRole;
// If role is not falsy value
// set `userRole` as passed `role` value
if (role) {
userRole = role;
} else {
// else set the `userRole` as USER
userRole = 'USER';
}
return userRole;
}
console.log( getUserRole() ) //=> "USER"
console.log( getUserRole('ADMIN') ) //=> "ADMIN"
简写方法:
但是使用短路求值(||
),我们可以用一行代码执行此操作,如下所示:
function getUserRole(role) {
return role || 'USER'; // <-- here
}
console.log( getUserRole() ) //=> "USER"
console.log( getUserRole('ADMIN') ) //=> "ADMIN"
基本上,expression1 || expression2
被评估为真
表达式。因此,这就意味着如果第一部分为真,则不必费心求值表达式的其余部分。
箭头函数
如果你不需要this
上下文,则在使用箭头函数时代码还可以更短:
let fruits = ['🍉', '🍊', '🍇', '🍎'];
fruits.forEach(console.log);
在数组中查找对象
你可以使用对象解构和箭头函数使代码更精简:
// Get the object with the name `Apples` inside the array
const getApples = array => array.find(({ name }) => name === "Apples");
let result = getApples(inventory);
console.log(result);
//=> { name: "Apples", quantity: 10 }
短路求值替代方案
const getUserRole1 = (role = "USER") => role;
const getUserRole2 = role => role ?? "USER";
const getUserRole3 = role => role ? role : "USER";
最后,我想借用一段话来作结尾:
代码之所以是我们的敌人,是因为我们中的许多程序员写了很多很多的狗屎代码。如果我们没有办法摆脱,那么最好尽全力保持代码简洁。
如果你喜欢写代码——真的,真的很喜欢写代码——你代码写得越少,说明你的爱意越深。
欢迎关注我的公众号:前端新世界
只关注前端技术,每日分享 JS / CSS 技术教程;Vue、React、jQuery等前端开发组件
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK