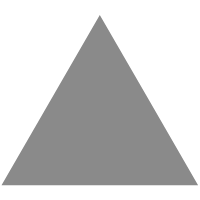
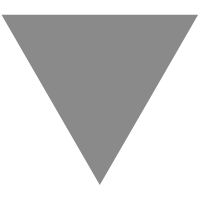
Laravel Unique Validation Rules Examples
source link: https://dev.to/saymontavares/laravel-unique-validation-rules-examples-163g
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
As you know laravel is a popular framework based on the PHP language. It is a Model, View, Controller (MVC) structure and has many in-built features. So today we will learn the unique validation feature in laravel and how you can implement it in laravel 6, laravel 7, and laravel 8.
There are two ways to add unique validation on email, username, or any other fields. First, we will with the database migration and second with form request.
1. Unique Validation with Database Migration
To add the unique validation in laravel migration, you have to tell the laravel that field will be unique. And you can do this by the unique() method. See the migration schema.
Schema::create('users', function (Blueprint $table) {
$table->increments('id');
$table->string('name');
$table->string('username')->unique();
$table->string('email')->unique();
$table->string('password');
$table->rememberToken();
$table->timestamps();
});
we applied a unique validation rule with the unique() method and made a username and email unique.
Now what if you want to handle and present the error more user-friendly then you can use the form request validation.
2. Laravel Form Request Unique Validation
Let’s start with create a form request.
$ php artisan make:request StoreUserRequest
Now, inject the request class into the controller that you just created.
namespace App\Http\Controllers;
use App\Http\Requests\StoreUserRequest;
use App\User;
class UserController extends Controller
{
public function store(StoreUserRequest $request) {
// your code here
}
public function update(UpdateUserRequest $request, User $user) {
// your code here
}
}
Now make a validation rule function in the StoreUserRequest class.
public function rules()
{
return [
'name' => 'required',
'username' => 'required',
'email' => 'required|email|unique:users'
];
}
That’s it!!!
You can also make validation rule for Update case, and Rule class.
I hope you enjoyed the read!
Feel free to follow me on GitHub, LinkedIn and DEV for more!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK