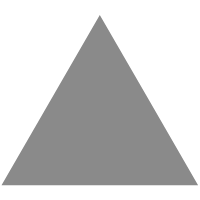
0
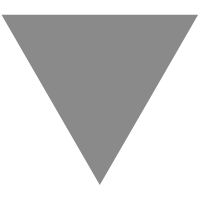
boost::algorithm::equal() in C++ library
source link: https://www.geeksforgeeks.org/boostalgorithmequal-in-c-library/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Improve Article
boost::algorithm::equal() in C++ library
- Last Updated : 17 Jun, 2019
The equal() function in C++ boost library is found under the header ‘boost/algorithm/cxx11/equal.hpp’ tests to see if two sequences contain equal values. It returns a boolean value which determines if both the sequences are same or not.
Syntax:
bool equal ( InputIterator1 first1, InputIterator1 second1, InputIterator2 first2, InputIterator2 second2 ) or bool equal ( InputIterator1 first1, InputIterator1 second1, InputIterator2 first2, InputIterator2 second2, BinaryPredicate pred )
Parameters: The function accepts parameters as described below:
- first1: It specifies the input iterators to the initial positions in the first sequence.
- second1: It specifies the input iterators to the final positions in the first sequence.
- first2: It specifies the input iterators to the initial positions in the second sequence.
- second2: It specifies the input iterators to the final positions in the second sequence.
- p: It specifies the comparison predicate if specified.
Return Value: The function returns true if both the sequence are same, else it returns false.
Note: The above function runs for C++14 and above.
Program-1:
// C++ program to implement the
// above mentioned function
#include <bits/stdc++.h>
#include <boost/algorithm/cxx14/equal.hpp>
using
namespace
std;
// Drivers code
int
main()
{
// Declares the sequences
int
a[] = { 1, 2, 5, 6, 8 };
int
b[] = { 1, 2, 5, 6, 8 };
// Run the function
bool
ans
= boost::algorithm::equal(a, a + 5, b, b + 5);
// Condition to check
if
(ans == 1)
cout <<
"Sequence1 and Sequence2 are equal"
;
else
cout <<
"Sequence1 and Sequence2 are not equal"
;
return
0;
}
Output:Sequence1 and Sequence2 are equal
Program-2:
// C++ program to implement the
// above mentioned function
#include <bits/stdc++.h>
#include <boost/algorithm/cxx14/equal.hpp>
using
namespace
std;
// Drivers code
int
main()
{
// Declares the sequences
int
a[] = { 1, 2, 5, 6, 8 };
int
b[] = { 1, 2, 5 };
// Run the function
bool
ans
= boost::algorithm::equal(a, a + 5, b, b + 5);
// Condition to check
if
(ans == 1)
cout <<
"Sequence1 and Sequence2 are equal"
;
else
cout <<
"Sequence1 and Sequence2 are not equal"
;
return
0;
}
Output:Sequence1 and Sequence2 are not equal
Want to learn from the best curated videos and practice problems, check out the C++ Foundation Course for Basic to Advanced C++ and C++ STL Course for foundation plus STL. To complete your preparation from learning a language to DS Algo and many more, please refer Complete Interview Preparation Course.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK