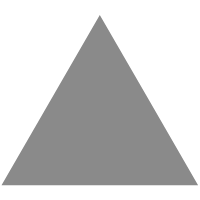
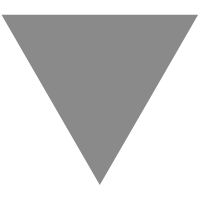
Find third number such that sum of all three number becomes prime
source link: https://www.geeksforgeeks.org/find-third-number-such-that-sum-of-all-three-number-becomes-prime/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Find third number such that sum of all three number becomes prime
- Last Updated : 18 Mar, 2021
Given two numbers A and B. The task is to find the smallest positive integer greater than or equal to 1 such that the sum of all three numbers become a prime number.
Examples:
Input: A = 2, B = 3
Output: 2
Explaination:
The third number is 2 because if we add all three number the
sum obtained is 7 which is a prime number.
Input: A = 5, B = 3
Output: 3
Approach:
- First of all store the sum of given 2 number in sum variable.
- Now, check if bitwise and (&) of sum and 1 is equal to 1 or not (checking if the number is odd or not).
- If it is equal to 1 then assign 2 to variable temp and go to step 4.
- Else check sum value of sum and temp variable whether it is prime number or not. If prime, then print the value of temp variable else add 2 to temp variable untill it is less than a prime value.
Below is the implementation of the above approach:
- Python3
- Javascript
// C++ implementation of the above approach
#include <bits/stdc++.h>
using
namespace
std;
// Function that will check
// whether number is prime or not
bool
prime(
int
n)
{
for
(
int
i = 2; i * i <= n; i++) {
if
(n % i == 0)
return
false
;
}
return
true
;
}
// Function to print the 3rd number
void
thirdNumber(
int
a,
int
b)
{
int
sum = 0, temp = 0;
sum = a + b;
temp = 1;
// If the sum is odd
if
(sum & 1) {
temp = 2;
}
// If sum is not prime
while
(!prime(sum + temp)) {
temp += 2;
}
cout << temp;
}
// Driver code
int
main()
{
int
a = 3, b = 5;
thirdNumber(a, b);
return
0;
}
3
Attention reader! Don’t stop learning now. Get hold of all the important DSA concepts with the DSA Self Paced Course at a student-friendly price and become industry ready. To complete your preparation from learning a language to DS Algo and many more, please refer Complete Interview Preparation Course.
In case you wish to attend live classes with experts, please refer DSA Live Classes for Working Professionals and Competitive Programming Live for Students.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK