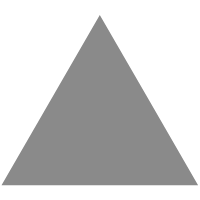
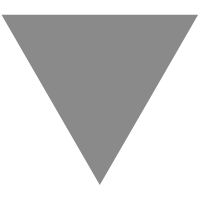
Xamarin.Forms lightweight bottom sheet modal
source link: https://xamarinhowto.com/xamarin-forms-lightweight-bottom-sheet-modal/?utm_campaign=xamarin-forms-lightweight-bottom-sheet-modal
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Xamarin.Forms lightweight bottom sheet modal
Bottom sheets are becoming increasingly more popular in modern apps including Facebook, Instagram and Microsoft Teams. A bottom sheet comes in three main forms including:
- Standard – the sheet remains visible while users can interact with the rest of the content on the screen.
- Modal – the sheet must be dismissed to interact with the rest of the content on the screen.
- Expanding – the sheet is able to be resized with ‘snap’ points often a low, middle and fullscreen view. The underlying content is also able to be interacted with by the user.
In this Xamarin how-to post, I will show you how you can create a control to display a modal bottom sheet.
The criteria:
- Pure Xamarin.Forms (no custom renderers)
- Change sheet content through binding
- Open sheet via button
- Close sheet by either swiping down or tapping outside of the sheet’s content
- Smooth + efficient
Start off by creating a blank ContentView class. We create the PanGesture on the ContentView rather than the frame itself. This is to stop jerkiness on Android due to the way that the OS handles translations.
The Control’s .XAML
The Control’s Code Behind
Consuming the Control
I know this looks like a lot of code but it is quite simple. The bottom sheet control uses a pan gesture recognizer and a couple simple animations (FadeTo and TranslateTo) to give the desired look and feel. This bottom sheet is very lightweight and takes a little performance hit. The content inside the frame could very easily be changed to collection view to be used as a toolbar / menu with the bindings set up accordingly. I hope this control can be used in your next project!
Full source code is available here
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK