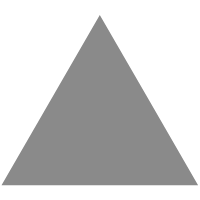
3
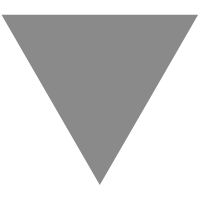
面试官:如何控制多线程执行顺序?
source link: https://blog.csdn.net/zzti_erlie/article/details/84926355
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
面试官:如何控制多线程执行顺序?
先看如下代码:
public class Test {
static Thread thread1 = new Thread(()-> {
System.out.println("thread1");
});
static Thread thread2 = new Thread(()-> {
System.out.println("thread2");
});
static Thread thread3 = new Thread(()-> {
System.out.println("thread3");
});
public static void main(String[] args) throws InterruptedException {
thread1.start();
thread2.start();
thread3.start();
}
}
重复执行多次,发现输出并不是按照线程的启动顺序来执行。因为这个里面涉及到CPU对线程的调度问题。
thread1
thread3
thread2
如何让thread1,thread2,thread3顺序执行呢?
通过join方法去保证多线程顺序执行
public class Test {
static Thread thread1 = new Thread(()-> {
System.out.println("thread1");
});
static Thread thread2 = new Thread(()-> {
System.out.println("thread2");
});
static Thread thread3 = new Thread(()-> {
System.out.println("thread3");
});
public static void main(String[] args) throws InterruptedException {
thread1.start();
thread1.join();
thread2.start();
thread2.join();
thread3.start();
}
}
可以看到输出一直是如下
thread1
thread2
thread3
join是怎么实现这个功能的呢?
join方法让主线程等待子线程结束以后才能继续运行,因此保证了线程的顺序执行
使用单例线程池,用唯一的工作线程执行任务,保证所有任务按照指定顺序执行
ExecutorService executorService = Executors.newSingleThreadExecutor();
这个会把线程放在一个FIFO队列,依次执行线程
public class Test {
static Thread thread1 = new Thread(()-> {
System.out.println("thread1");
});
static Thread thread2 = new Thread(()-> {
System.out.println("thread2");
});
static Thread thread3 = new Thread(()-> {
System.out.println("thread3");
});
static ExecutorService executorService = Executors.newSingleThreadExecutor();
public static void main(String[] args) throws InterruptedException {
executorService.submit(thread1);
executorService.submit(thread2);
executorService.submit(thread3);
executorService.shutdown();
}
}
输出一直为
thread1
thread2
thread3
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK