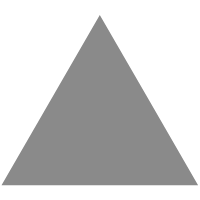
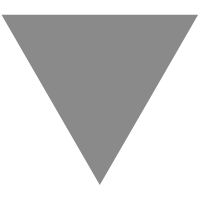
C# tip: String.IsNullOrEmpty or String.IsNullOrWhiteSpace?
source link: https://www.code4it.dev/csharptips/string-isnullorempty-isnullorwhitespace
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
C# tip: String.IsNullOrEmpty or String.IsNullOrWhiteSpace?

Imagine this: you have created a method that creates a new user in your system, like this:
void CreateUser(string username)
{
if (string.IsNullOrEmpty(username))
throw new ArgumentException("Username cannot be empty");
CreateUserOnDb(username);
}
void CreateUserOnDb(string username)
{
Console.WriteLine("Created");
}
It looks quite safe, right? Is the first check enough?
Let's try it: CreateUser("Loki")
prints Created, while CreateUser(null)
and CreateUser("")
throw an exception.
What about CreateUser(" ")
?
Unfortunately, it prints Created: this happens because the string is not actually empty, but it is composed of invisible characters.
The same happens with escaped characters too!
To avoid it, you can replace String.IsNullOrEmpty
with String.IsNullOrWhiteSpace
: this method performs its checks on invisible characters too.
So we have:
String.IsNullOrEmpty(""); //True
String.IsNullOrEmpty(null); //True
String.IsNullOrEmpty(" "); //False
String.IsNullOrEmpty("\n"); //False
String.IsNullOrEmpty("\t"); //False
String.IsNullOrEmpty("hello"); //False
but also
String.IsNullOrWhiteSpace("");//True
String.IsNullOrWhiteSpace(null);//True
String.IsNullOrWhiteSpace(" ");//True
String.IsNullOrWhiteSpace("\n");//True
String.IsNullOrWhiteSpace("\t");//True
String.IsNullOrWhiteSpace("hello");//False
As you can see, the two methods behave in a different way.
If we want to see the results in a tabular way, we have:
value IsNullOrEmpty IsNullOrWhiteSpace"Hello"
false
false
""
true
true
null
true
true
" "
false
true
"\n"
false
true
"\t"
false
true
Conclusion
Do you have to replace all String.IsNullOrEmpty
with String.IsNullOrWhiteSpace
? Probably yes, unless you have a specific reason to consider the latest three values in the table as valid characters.
More on this topic can be found here
👉 Let's discuss about it on Twitter or on the comment section below.
Published under CSharp, CSharp Tip on 06 July, 2021.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK