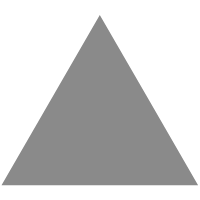
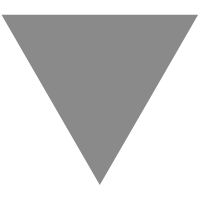
Find a specific char in an array of characters
source link: https://www.codesd.com/item/find-a-specific-char-in-an-array-of-characters.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Find a specific char in an array of characters
I am doing an application where I want to find a specific char in an array of chars. In other words, I have the following char array:
char[] charArray= new char[] {'\uE001', '\uE002', '\uE003', '\uE004', '\uE005', '\uE006', '\uE007', '\uE008', '\uE009'};
At some point, I want to check if the character '\uE002'
exists in the charArray
. My method was to make a loop on every character in the charArray
and find if it exists.
for (int z = 0 ; z < charArray; z ++) {
if (charArray[z] == myChar) {
//Do the work
}
}
Is there any other solution than making a char array and finding the character by looping every single char?
One option is to pre-sort charArray
and use Arrays.binarySearch(charArray, myChar)
. A non-negative return value will indicate that myChar
is present in charArray
.
char[] charArray = new char[] {'\uE001', '\uE002', '\uE003', '\uE004', '\uE005', '\uE006', '\uE007', '\uE008', '\uE009'};
Arrays.sort(charArray); // can be omitted if you know that the values are already sorted
...
if (Arrays.binarySearch(charArray, myChar) >= 0) {
// Do the work
}
edit An alternative that avoids using the Arrays
module is to put the characters into a string (at initialization time) and then use String.indexOf():
String chars = "\uE001...";
...
if (chars.indexOf(myChar) >= 0) {
// Do the work
}
This is not hugely different to what you're doing already, except that it requires less code.
If n
is the size of charArray
, the first solution is O(log n)
whereas the second one is O(n)
.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK