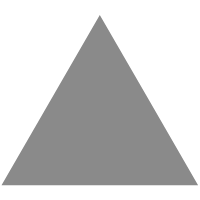
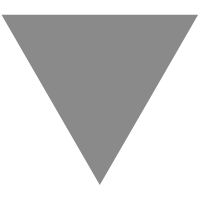
How to Make a Twitter Bot Using Python
source link: https://dev.to/ejach/how-to-make-a-twitter-bot-using-python-2l1k
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
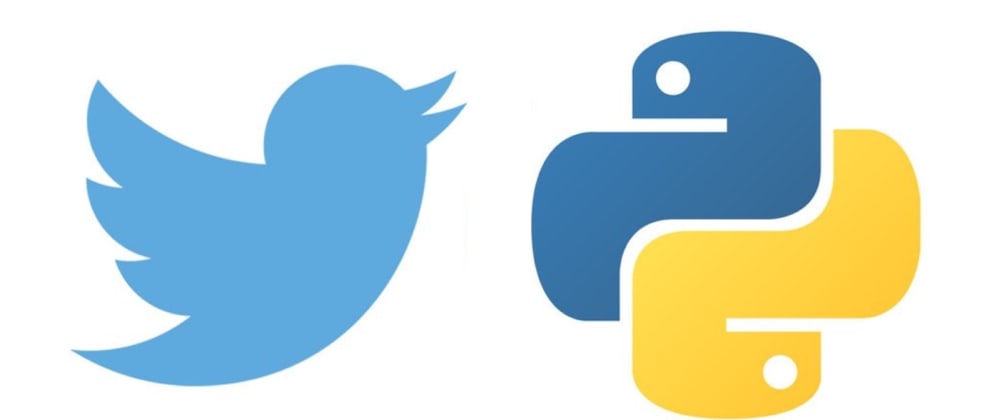
How to Make a Twitter Bot Using Python
Jul 1
・3 min read
Hello!
This tutorial will demonstrate how to create a simple Twitter bot using the Tweepy library in Python.
This tutorial will be separated into four parts:
Prerequisites
Setting up a Twitter Developer Account
To create a Twitter bot you must apply for a Twitter Developer Account detailed in this link.
You must describe the use-cases that you intend to satisfy using their API. This application process can take anywhere from an hour to a day, so be patient!
Once accepted you will get an email detailing how to access the developer portal. Login to the developer portal and follow the steps to create a Twitter app.
Make sure your app has Read + Write + Read and post direct messages
permissions by going to this screen:
Once it is created and the permissions are set, hit the Key 🗝 icon next to your app, and you should see this screen:
Generate and take note of the API key/secret
and the access token/secret
. I recommend putting them in a text document, as you cannot access them again once they are generated.
Next we will go over creating the application.
Creating the Application using Python
We will be creating a Twitter bot that simply tweets Hello world!
The file structure by the end of this tutorial will look like:
├───twitterbot
└───.env # File that contains our keys
└───main.py # Our main application
└───requirements.txt # The packages we will be using
To start out, create an empty directory using:
$ sudo mkdir twitterbot
And cd
into the directory using:
$ cd twitterbot
Create two files called main.py
and requirements.txt
.
In requirements.txt, insert the line:
tweepy==3.10.0
python-dotenv==0.17.1
Then, to install the required packages, run:
$ pip install -r requirements.txt
tweepy
is the library that utilizes the Twitter API, and python-dotenv
is the library that allows environment variables to be utilized in your program.
Second, we will go over where to put the keys/tokens.
Create a file called .env
and paste the following with the keys you generated in the earlier steps:
consumer_key=XXX # Found in the API/Secret section
consumer_secret=XXX # Found in the API/Secret section
access_token=XXX # Found in the Access Token/Secret section
access_token_secret=XXX # Found in the Access Token/Secret section
It is a good practice to utilize environment variables when dealing with sensitive credentials like this. Never put your credentials directly in your code.
Next we will go over how to utilize the credentials in our code. In our main.py
file, add the following:
import os
import tweepy
from dotenv import load_dotenv
# Loads the .env file for the credentials
load_dotenv()
# Credentials set in the .env file
consumer_key = os.environ.get('consumer_key')
consumer_secret = os.environ.get('consumer_secret')
access_token = os.environ.get('access_token')
access_token_secret = os.environ.get('access_token_secret')
We will now implement the tweepy
library to use these credentials and authenticate with Twitter:
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
# Create API object
api = tweepy.API(auth)
# Checks if the credentials entered are correct
def authenticate():
if not api.verify_credentials():
print('Authentication: FAILED')
return False
else:
print('Authentication: OK')
return True
Next, we will implement a function that handles the tweet itself as well as a main
function:
def tweet():
# If authenticate returns true, execute the following
if authenticate():
# Calls the API to tweet the following
api.update_status('Hello World!')
print('Tweet has been sent!')
# If authenticate returns false, print the following
else:
print('Tweet has not been sent.')
if __name__ == "__main__":
tweet()
Running the Application
Now that all of our code has been implemented, you can run the program using python main.py
and you should see the following:
Authentication: OK
Tweet has been sent!
And you should see that your tweet has been sent:
Congratulations! You have successfully created a Twitter bot using Python!
If you need to view the files we created for any reason, check it out on GitHub:
ejach
/
twitterbot
This is an example Twitter bot that uses the tweepy library. It was written for an article that can be read on dev.to.
twitterbot
This is an example Twitter bot that uses the tweepy library. It was written for an article that can be read on dev.to.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK