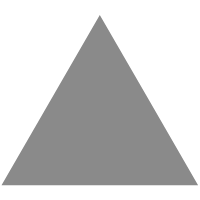
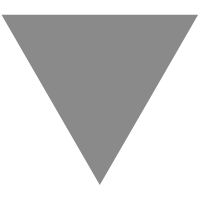
JavaScript – Base64 Data URLs Outside of the Latin1 Range
source link: https://cwestblog.com/2021/06/30/javascript-base64-data-urls-outside-of-latin1-range/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
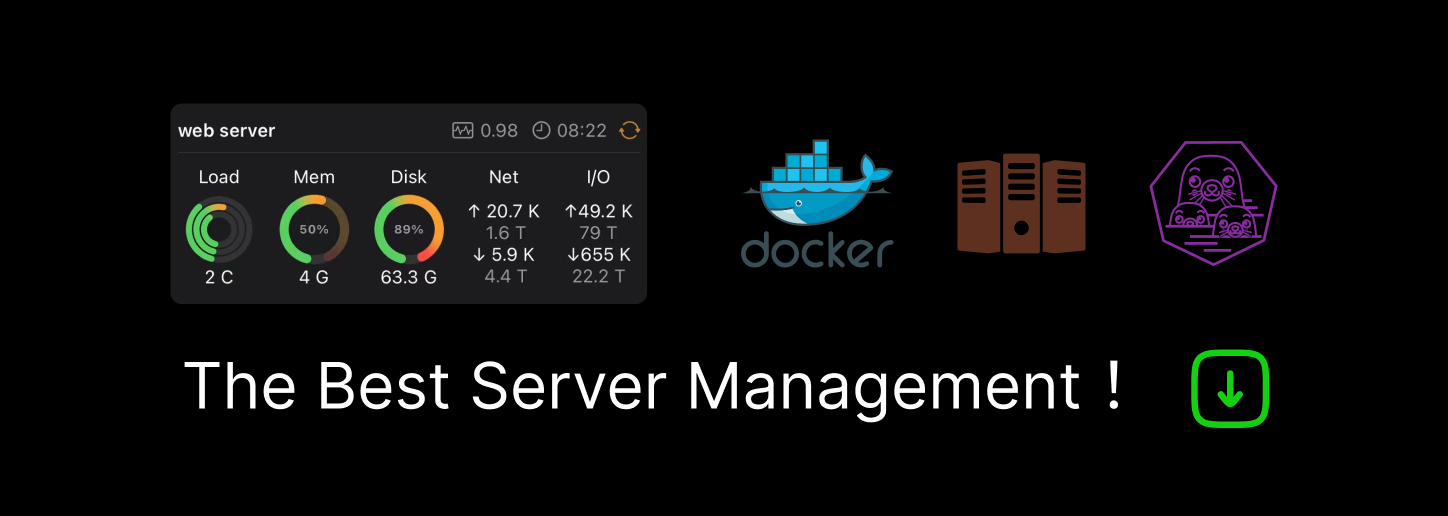
It is often handy to create downloadable content on the fly using JavaScript. Sometimes you may want to do so while also encoding the contents as base64. That is where the btoa()
function comes in handy. Here is an example of doing just that:
let
content =
'Hello JS world!!!'
;
Object.assign(document.createElement(
'a'
), {
href:
'data:text/plain;charset=utf-8;base64,'
+ btoa(content),
download:
'example-'
+ (+
new
Date()) +
'.txt'
}).click();
As you can see by clicking here, the above code does in fact make it possible to download an example text file with the message “Hello JS world!!!”.
I’m Getting An Outside of Latin1 Range Error!
If you are getting this error you are undoubtedly trying to use this same method with special characters that are outside of the \u0000
to \u00ff
. The following is an example of code that will not work using the same method as outlined above:
let
content =
'Hello JS world!!! This has been a very interesting\u2026'
;
Object.assign(document.createElement(
'a'
), {
href:
'data:text/plain;charset=utf-8;base64,'
+ btoa(content),
download:
'example-'
+ (+
new
Date()) +
'.txt'
}).click();
By clicking here you will see the error that is produced when trying to run the above code. In order to get this code to work correctly you can turn all of the characters into a Latin1 range string that when interpreted as UTF-8 will still produce the expected result. The 2 functions that you can use together are encodeURIComponent()
followed by unescape()
. Here is the working code:
let
content =
'Hello JS world!!! This has been a very interesting\u2026'
;
Object.assign(document.createElement(
'a'
), {
href:
'data:text/plain;charset=utf-8;base64,'
+ btoa(unescape(encodeURIComponent(content))),
download:
'example-'
+ (+
new
Date()) +
'.txt'
}).click();
Click here if you want to see if this really works.
Final Words
I have found that simply making the data URL without base64 seems to be easiest option:
let
content =
'Hello JS world!!! This has been a very interesting\u2026'
;
Object.assign(document.createElement(
'a'
), {
href:
'data:text/plain;charset=utf-8,'
+ encodeURIComponent(content),
download:
'example-'
+ (+
new
Date()) +
'.txt'
}).click();
Click here to see the above code in action. As you can see we only need to use the encodeURIComponent()
function in the case that we are not specifying base64 encoding of the data URL. For this reason it seems that the best option is to simply encode the contents once without having to use base64 (via btoa()
) at all.
Let me know what you think and what you have found has worked and/or has not worked. As all always, happy coding!!!
Recommend
-
13
Base64 Encoding and Decoding in JavaScript in Adobe AIR Thursday, June 12, 2008 I have been working on a JavaScript AIR app for the past week or so while on the on AIR Tour in Europe, and ran into the need to Base64 enc...
-
10
XML document read as Latin1 but half converted to UTF-8 advertisements I'm hitting my head off a brick wall with a bizarre problem that I ...
-
10
Introduction In JavaScript, it is possible to use Base64 to encode and decode strings. In this article, you will be introduced to the btoa and atob...
-
9
The Base16, Base32, and Base64 Data Encodings Network Working Group S. Josefsson Request for Comments: 4648 SJD Obsoletes:
-
1
Remove BST keys outside the given rangeSkip to content
-
11
How to get URLs in JavaScript for uploaded files in MediaWiki
-
5
A Uniform Resource Locator (URL) is what leads you to a page or file on the internet. URLs serve as the addresses of things on the internet.All valid URLs follow certain patterns. So if you know those patterns, you can determine whether a U...
-
9
@maratMaratHi guys, I'm Marat, a software developer with expertise i...
-
7
A Handy Guide to Parsing URLs in JavaScriptA Handy Guide to Parsing URLs in JavaScriptMarch 1st 2023 New Story7 min...
-
7
How To Parse URLs in JavaScript In this article, readers will do a...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK