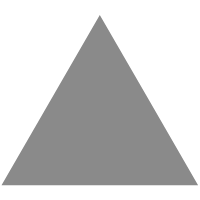
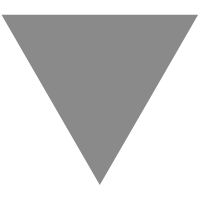
Counting a Non-Generic IEnumerable
source link: https://www.stevefenton.co.uk/2021/06/counting-a-non-generic-ienumerable/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
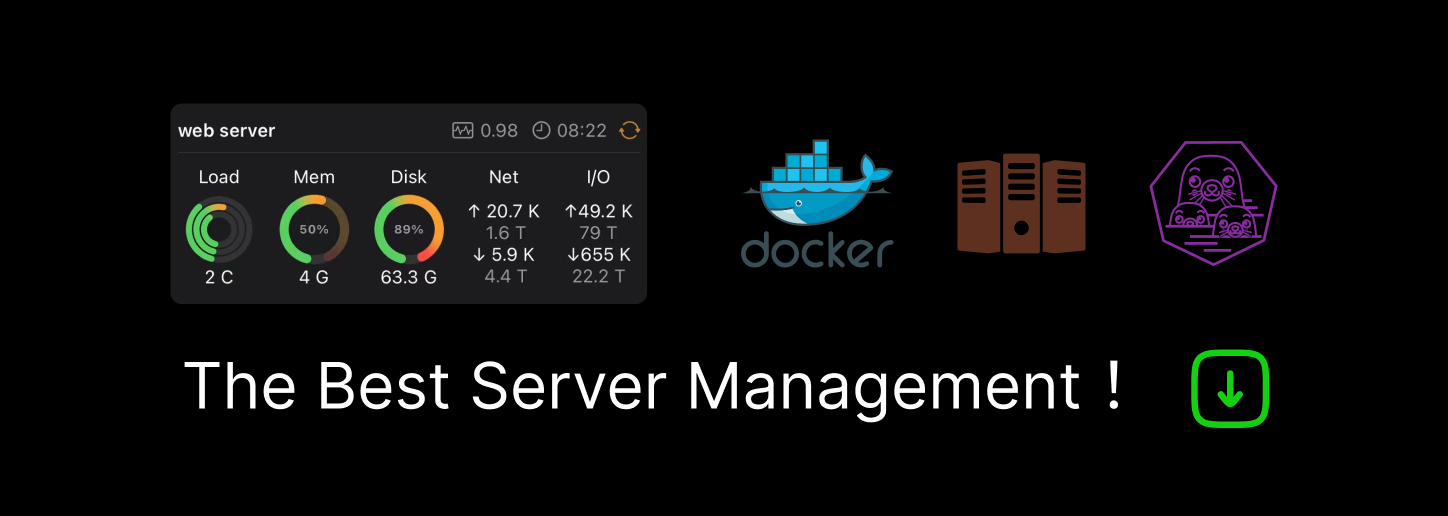
Counting a Non-Generic IEnumerable
You rarely come across them in 2021, but there is such a thing as a non-generic IEnumerable
. They exist in System.Collections
rather than System.Collections.Generic
. Since .NET 2 pretty much everyone is full-on using generics as they are the best thing since curly-braces… but occasionally you find one and even more rarely you need to count it.
Now, you can’t just use System.Linq
to count an IEnumerable
because Linq works on your generics. However, Linq is a useful starting point because it’s basically a whole bunch of cool extension methods. We can create our own extension method to add Count()
to an IEnumerable
, such as the SelectedItems
in a MultiSelectList
.
Here’s the extension that does it, including a helper that allows us to dispose of the enumerator if it is disposable.
public static class EnumerableExtensions { public static int Count(this IEnumerable source) { if (source is ICollection collection) { return collection.Count; } int count = 0; var enumerator = source.GetEnumerator(); DynamicUsing(enumerator, () => { while (enumerator.MoveNext()) { count++; } }); return count; } public static void DynamicUsing(object resource, Action action) { try { action(); } finally { if (resource is IDisposable disposable) { disposable.Dispose(); } } } }
To use this code, we just call it on our IEnumerable
, like this…
int count = multiSelectList.SelectedItems.Count();
Recommend
-
10
Non-Generic Inner Functions Oct 20th, 2020 · Pattern · #generics...
-
10
Linq to Collections: Beyond IEnumerable<T> posted by Craig Gidney on December 18, 2012 The latest version of the .Net...
-
6
Why refactor the argument of List & lt; Term & gt; to IEnumerable & lt; Term & gt; advertisements I have a method that looks l...
-
16
IEnumerator、IEnumerable这两个接口单词相近、含义相关,傻傻分不清楚。 入行多年,一直没有系统性梳理这对李逵李鬼。 最近本人在怼着why神的《其实吧,LRU也就那么回事》,方案1使用数组实现LRU,手写算法涉及这一对接口,借...
-
5
IEnumerable<T> Interface Definition Namespace: System.Collections.Generic Assembly:System.Runti...
-
4
.NET Collections – IEnumerable, IQueryable, ICollection Posted by Code Maze | Updated Date Nov 1, 2021 |
-
2
André Slupik Posted on Mar 3...
-
8
View .NET collections with the new IEnumerable Debugger Visualizer Harshada H April 21st, 2022 While debugging .NET code,...
-
8
IEnumerable Visualizer In Visual Studio 2022Visual Studio 2022 17.2 shipped the other day, and in it was a handy little feature that I can definitely see myself using a lot going forward. That is the IEnumerable Visu...
-
9
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK