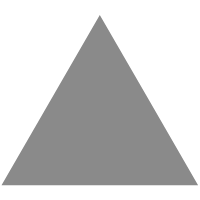
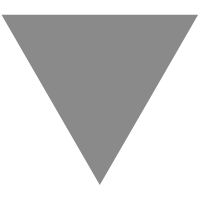
Take a journey with your own map service by AWS Amplify and Amazon Location Serv...
source link: https://dev.to/aws-builders/take-a-journey-with-your-own-map-service-by-aws-amplify-and-amazon-location-service-1nj6
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Amazon Location Service is Generally Available on June 1st, 2021.
https://aws.amazon.com/jp/blogs/aws/amazon-location-service-is-now-generally-available-with-new-routing-and-satellite-imagery-capabilities/
So, we can make our own map service which used Amazon Location Service.
This post shows how to make your own map service by AWS Amplify and Amazon Location Service.
We can search the location and display it on the map.
1. Setup
- Vue CLI (+ Vuetify)
- Amplify CLI
1.1. Vue CLI
Install "Vue CLI."
Vue CLI
$ vue -V
@vue/cli 4.5.13
1.2. Amplify CLI
Install "Amplify CLI."
AWS Amplify
$ amplify -v
Scanning for plugins...
Plugin scan successful
4.52.0
1.3. Create Vue project
Create a Vue project by Vue CLI
.
1.4. Setup Amplify project
Setup Amplify project.
amplify initAnd don't forget to add UI Components.
$ yarn add aws-amplify @aws-amplify/ui-vue
$
1.5. Add other packages
This time we use "MapLibre GL JS," so we need to add these packages.
See the official document for the detail.
$ yarn add aws-sdk @benchmark-urbanism/vue-mapbox-map maplibre-gl
1.6. Edit main.js
Then, we edit src/main.js
like below.
import Vue from 'vue'
import App from './App.vue'
import vuetify from './plugins/vuetify'
import '@aws-amplify/ui-vue'
import Amplify from 'aws-amplify'
import awsconfig from './aws-exports'
Amplify.configure(awsconfig)
import 'maplibre-gl/dist/maplibre-gl.css'
Vue.config.productionTip = false
new Vue({
vuetify,
render: (h) => h(App),
}).$mount('#app')
2. Add Auth
Add Authentication.
amplify add authThen, Push project.
amplify push3. Create a Map and a Search index
3.1. Create a Map
Next, we open the AWS console by the browser and move to "Amazon Location Service."
- Click the "Maps" menu on the left panel.
- And click "Create map" in the My maps area.
- Input the map name "SampleMap" in the Name field.
- Select the map in the Maps area. In this case, we select "Esri Light."
- Select "Pricing plan use-case." In this case, we select "Yes" to the first question.
- Push "Create map" at the bottom of the page.
3.2. Create a Search index
Continuously, we create a Place index.
- Click the "Search indexes" menu on the left panel.
- And click "Create place index" in the My maps area.
- Input the map name "SamplePlaceIndex" in the Name field.
- Select the data provider in the Data provider area. In this case, we select "Esri."
- Select the data storage option in the Data storage options area. In this case, we select "No, single use only."
- Select "Pricing plan use-case." In this case, we select "Yes" to the first question.
- Push "Create place index" at the bottom of the page.
4. Set IAM Role
We have already added the authentication function by Amplify.
However, the IAM role is incomplete. Unable to access previously created location services resources.
Let's add it.
- Go to IAM Services and click the Roles menu on the left panel.
- Input "amplify-amplifyamazonlocatio" in the search field.
- Click the role that name ends with "-authRole".
- Click "Add inline policy".
- Select the JSON tab and input below JSON data. (Set your ARN in [MAP_ARN] and [PLACE_INDEX_ARN].)
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "VisualEditor0",
"Effect": "Allow",
"Action": [
"geo:GetMapGlyphs",
"geo:GetMapSprites",
"geo:GetMapStyleDescriptor",
"geo:GetMapTile"
],
"Resource": [MAP_ARN]
},
{
"Sid": "VisualEditor1",
"Effect": "Allow",
"Action": "geo:SearchPlaceIndexForText",
"Resource": [PLACE_INDEX_ARN]
}
]
}
5. Implement
OK, we can implement a search place and show it on the map.
Please see the repository for code details.
5.1. Search the location
"Search the location" implementation is like below.
Only use searchPlaceIndexForText
, we can get the place information.
search: function () {
if (!this.place || this.place.length === 0) {
return
}
const params = {
IndexName: 'SamplePlaceIndex',
Text: this.place,
}
this.client.searchPlaceIndexForText(params, (err, data) => {
if (err) {
console.error(err)
return
}
if (data) {
console.log(data)
if (data.Results && data.Results.length > 0) {
this.lng = data.Results[0].Place.Geometry.Point[0]
this.lat = data.Results[0].Place.Geometry.Point[1]
}
}
})
},
5.2. Display the location on the map
"Display the location on the map" implementation is like below.mapInstance
is the object rendering the map.transformRequest
make a Signed URL for access to the Map resource previously created.
mounted: async function () {
this.credentials = await Auth.currentCredentials()
this.mapInstance = new maplibregl.Map({
container: 'map',
style: this.mapName,
center: [this.scene.lng, this.scene.lat],
zoom: this.scene.zoom,
transformRequest: this.transformRequest,
})
this.mapInstance.addControl(new maplibregl.NavigationControl(), 'top-left')
this.mapInstance.on('load', function () {
this.resize()
})
},
(snip)
transformRequest: function (url, resourceType) {
if (resourceType === 'Style' && !url.includes('://')) {
url = `https://maps.geo.${awsconfig.aws_project_region}.amazonaws.com/maps/v0/maps/${url}/style-descriptor`
}
if (url.includes('amazonaws.com')) {
return {
url: Signer.signUrl(url, {
access_key: this.credentials.accessKeyId,
secret_key: this.credentials.secretAccessKey,
session_token: this.credentials.sessionToken,
}),
}
}
return { url }
},
6. Take a journey
We arrive at the final section.
Let's get to the journey!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK