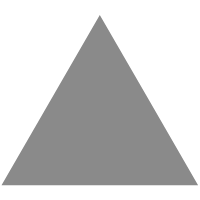
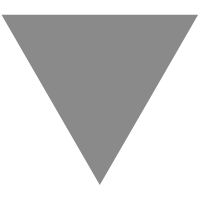
Creating and Debugging Website Routers
source link: https://hackernoon.com/creating-and-debugging-website-routers-hh1g34aj
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Creating and Debugging Website Routers
















@veloVelo by Wix
Velo is a full-stack development platform that empowers you to rapidly build, manage and deploy professional web apps.
Creating a router allows you to take complete control when handling certain incoming requests to your site.




This article takes you through what code you have to write in order to make your router work. To learn more about what a router is and why you would want to create one, see About Routers.




Add a Router
To add a router:




1. Click on the




icon that appears when hovering over the Page Code's Main Pages section in the Velo Sidebar and choose Add a Router.




2. Enter a URL prefix for your router and click Add & Edit Code. All incoming requests with the specified URL prefix will be sent to your router for handling.




When adding a router:




- Your router's
androuter()
functions are added in a routers.js file with sample code for a simple routing scenario. The routers.js file is found in the Code Files's Backend section of the Velo Sidebar.sitemap()
- A new section, Router Pages, is created in the Velo Sidebar for your routers. Router pages are grouped together under a title based on the prefix you chose earlier. One router page is created to start with. For example, if you named your router "myRouter", a page named myRouter-page is added under the title MyRouter Pages (Router).
Sample Scenario
There are four parts to the sample code that's added to the routers.js file:




Import statement




The functionality used to create a router is contained in the Router API. To use this functionality, you need to import it. By default, the
ok()
and notFound()
functions are imported, as well as the WixRouterSitemapEntry
object.



import {ok, notFound, WixRouterSitemapEntry} from "wix-router";
If you want to use more of the functionality from the Router API, you need to add it to the
import
statement.



Sample Data




In the sample scenario, the router uses some static data contained in an object named
peopleData
.



const peopleData = {
"Ash": {
title: "Ash Stowe",
image: "https://static.wixstatic.com/media/b8f383e0fe2b478ea91362b707ef267b.jpg"
},
"Aiden": {
title: "Aiden Johnson",
image: "https://static.wixstatic.com/media/ca3c7ac5427e43928aa5f3f443ae2163.jpg"
},
"Jess": {
title: "Jess White",
image: "https://static.wixstatic.com/media/147fe6f37fe24e83977b4124e41b6d3d.jpg"
},
"Morgan": {
title: "Morgan James",
image: "https://static.wixstatic.com/media/59e1f2f4dbbc4f7c9d6e66e3e125d830.jpg"
}
};
The
peopleData
object contains four keys, each representing a person. Each person contains two properties, a title
that is the person's full name and an image
that is a link to an image of the person.



Although you can use static data with a router, typically you would use data
from your site's database or an external source. We'll see where you would write code to retrieve that data a little later.




Regardless of where you get the data from, since you'll be writing the code that handles it, the data can be structured in any way you like.




Router Function




Remember, all incoming requests with the URL prefix you specified when creating the router are sent to your router for handling. The
router()
function is where you handle those requests.



The
router()
function is named with the following convention:



<router prefix>_Router(request)
So if you named your router myRouter, the code added to the routers.js file should look like:




myRouter_Router(request) { // routing code ...}
The
router()
function has a request parameter which receives a WixRouterRequest
object that contains information about the incoming request. The object has information about the URL used to reach the router, where the request came from, and who the request came from.



Typically, the
router()
function will decide which page to show (if any) and what data to pass to the page. A response is then sent using the forbidden()
, notFound()
, ok()
, redirect()
, or
sendStatus()
functions.



Let's take a look at the sample
router()
function code:



export function myRouter_Router(request) {
// Get item name from URL request
const name = request.path[0];
// Get the item data by name
const data = peopleData[name];
if (data) {
//define SEO tags
const seoData = {
title: data.title,
description: `This is a description of ${data.title} page`,
noIndex: false,
metaTags: [
{"og:title": data.title,
"og:image": data.image,
content: "People Data"
}
]
};
// Render item page
return ok("myRouter-page", data, seoData);
}
// Return 404 if item is not found
return notFound();
}
The
router()
function begins by parsing the request's path to pull out a name value.



const name = request.path[0];
For example, a user might reach this
router()
function by browsing to https://mysite.com/myRouter/Ash
. The received WixRouterRequest
object's path
property will be an array with one element: ["Ash"]
. Now the value of name is "Ash
".



Next, the
router()
function retrieves data based on the name
it received.



const data = peopleData[name];
Continuing our example above, we pull out Ash's information from the
peopleData
object.



So
data
now holds:



{
title: "Ash Stowe",
image: "https://static.wixstatic.com/media/b8f383e0fe2b478ea91362b707ef267b.jpg"
}
If you want to retrieve data from an external source, this is where you place the call to retrieve that data.




After attempting to retrieve the data that corresponds to the incoming request, we check to see if any data was found:




if (data) {
// ...
}
If nothing was found, the
if
is skipped and we return a 404 error using the notFound()
function.



return notFound();
Assuming we found the data we were looking for, we now prepare some header data for our page:




const seoData = {
title: data.title,
description: `This is a description of ${data.title} page`,
noIndex: false,
metaTags: {
"og:title": data.title,
"og:image": data.image
}
};
Here we create a
HeadOptions
object that defines what will be put in the HTML head of the page we respond to the request with. That object is stored in the seoData variable.



The sample code creates a
title
and description
based on the title
property of the retrieved data
object. It also sets noIndex
to false, meaning search engines should index the page. Finally, some metaTags
properties are added as well.



You can create the
HeadOptions
object using any information you want.



You can also add a keywords property to the
HeadOptions
object with a string containing the page's keywords.



Finally, we return a
WixRouterResponse
object using the ok()
function:



return ok("myRouter-page", data, seoData);
Here we route the user to the router page named "
myRouter-page
" and pass it the data
that was retrieved and the seoData
that was built for the page.



Depending on the situation, you can return a number of different responses from the
router()
function. So far we've seen the notFound()
and ok()
functions in use. You can also use the forbidden()
function to return a 403
response, the redirect()
function to return a 301
or 302
response, or the sendStatus()
function to return a response with any status code you choose.



Router Data




To use the data that was returned with a
WixRouterResponse
using the ok()
function, use the wix-
window getRouterData()
function.



For example, we can take the data passed by the sample router code and use it to present a person's information on the myRouter-page page that was created.




First, we need to add a text and image element to serve as placeholders for a person's title and image.




Next, in the page's code we retrieve the router data and set the text and image elements to display the
title
and image
from the data.



import wixWindow from 'wix-window';
$w.onReady(function () {
let data = wixWindow.getRouterData();
$w("#text1").text = data.title;
$w("#image1").src = data.image;
});
If you preview the page, you'll see that the placeholders we put on the page are filled in with information from the data that was passed to the page. You can use the preview widget to see what the page looks like for any of the people in the
peopleData
object that was defined in the routers.js file.



The preview widget gets populated with the
title
values from the WixRouterSitemapEntry
objects that are returned by the router's sitemap()
function.



Sitemap Function




Like the
router()
function, the sitemap()
function is named with the following convention:



<router prefix>_Sitemap(sitemapRequest)
So if you named your router myRouter, the code added to the routers.js file should look like:




myRouter_Sitemap(sitemapRequest) { // routing code ...}
The
sitemap()
function has a sitemapRequest
parameter which receives a WixRouterSitemapEntry
object that contains information about the incoming request. The object has information about the URL used to reach the router, the pages in the router, and who the request came from.



Typically, the
sitemap()
function creates a WixRouterSitemapEntry
object for each item in the data used by the router. So, in our example, we create an entry for each person in the personData
object. You also might want to include entries for other pages, like an index page or a search page.



Let's take a look at the sample
sitemap()
function code:



export function myRouter_SiteMap(sitemapRequest) {
// Convert the data to site map entries
const siteMapEntries = Object.keys(peopleData).map( (name) => {
const data = peopleData[name];
const entry = new WixRouterSitemapEntry(name);
entry.pageName = "myRouter-page"; // The name of the page in the Wix Editor to render
entry.url = `/myRouter/${name}`; // Relative URL of the page
entry.title = data.title; // For better SEO - Help Google
return entry;
} );
// Return the site map entries
return siteMapEntries;
}
The
sitemap()
function takes the keys of the peopleData object and uses the JavaScript map()
function to create an array of WixRouterSitemapEntry
objects, one object for each key. Each entry is given values for the pageName
, url
, and title
properties. Then the array is wrapped in a Promise
and returned.



A sitemap entry can also contain
changeFrequecy
, lastModified
, and priority
properties to give search engines more information about each page.



In a case where you're using a router with external data, in the
sitemap()
function you would retrieve that data and build sitemap entries for each item that was retrieved.



Previously published at https://support.wix.com/en/article/velo-creating-a-router




















Create your free account to unlock your custom reading experience.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK