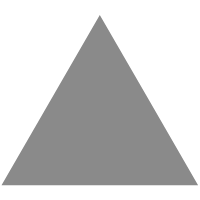
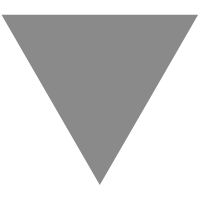
From Junior to Master in Kotlin — 2 (Safe null)
source link: https://medium.com/google-developer-experts/from-junior-to-master-in-kotlin-2-safe-null-188839da41ab
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
From Junior to Master in Kotlin — 2 (Safe null)
Kotlin by default does not allow null initializations unless you tell it otherwise. Personally, I love that Kotlin “hates” nulls, it forces me to initialize and implement proper strategies to handle nulls if we have to.
One of the most common mistakes in many programming languages is when we try to access a member of a null reference, which will result in a null pointer exception or NPE for short.
Let me show you how to declare a variable not null and how Kotlin throws an error when I assign a null to the variable:

You run the code:

So good, a compilation error comes in. To be honest, I prefer this kind of error instead of runtime exceptions, when the app is running and crashes due to NPE it seems that the app wasn’t developed carefully (my opinion).
If you need that you code allows nulls (You are weird but we love you :D, it is a joke) you could do the following:
var name: String? = “Gerardo” // can be set null
print(name) //Prints Gerardoname = null // ok
print(name) //Prints null
But if you want to access the same property on `name`, that wouldn’t be safe, and the compiler reports an error:
val length = name.length // error: variable ‘name’ could be null
But what if you need to access properties or methods of your variables that could be null? There are a few ways of doing that.
Safe calls
The safe call operator is ?
. See the following example:
val name= "Gerardo"
val lastName: String? = null
println(name?.length) // Unnecessary safe call
println(lastName?.length)
The safe operator first checks if the value isn’t null so allows the execution, otherwirse returns a null. Bye bye Null pointers exceptions… You were History. So about the example, the compiler will return a null when the lastName variable tries to access length property and won’t throw an error. Yessss, saaaafeeeee cooodeeeee.
The !! operator
Kotlin respects all developers decisions, good or bad, but repects all. So, if you for some reason love the NPE, Kotlin allows it, but I would like very good why I need a NPE… If you want to allow NPE, use !!
val lastNameTemp= lastName!!.length
If lastName is null then a ungly NPE will be thrown.
Elvis operator
Another option could be use the elvis operator ?:
as following:
val l = lastName?.length ?: "Falcon"
If the expression to the left of ?:
is not null
, the operator returns it, otherwise will use the default value that you chose, in this context it is “Falcon”. No bad.
Conclusion
Enough info by today. In other posts I will talk about other advanced choices such as lazy loading and lateinit… Thank you for following me and sharing it with your friends.
Long Live Kotlin.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK