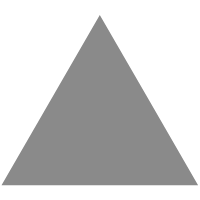
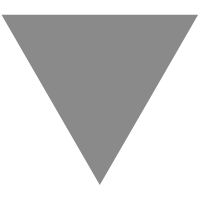
Python Argparse for command-line arguments - Knoldus Blogs
source link: https://blog.knoldus.com/python-argparse-parser-for-command-line-options-arguments-and-sub-commands/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Python Argparse: Parser for command-line options, arguments and sub-commands
Python Argparse Concept:
When you run the “ls” command without any options, it will display all the files and directories in the current directory.
If you want to run the “ls” command on a different directory from the current directory, you would type “ls directory_name”. The “directory_name” is a “positional argument”, which means that the program knows what to do with the value.
If you want to get more information about files we can use the “-l” switch. The “-l” is knowns as an “optional argument”.
Using the python argparse module we can create custom positional and optional arguments.
Python Argparse
The argparse module makes it easy to write user-friendly command-line interfaces. The program defines what arguments it requires, and argparse will figure out how to parse those out of sys.argv. The argparse module also automatically generates help and usage messages and issues errors when users give the program invalid arguments.
Parsing command-line arguments is a very common task, which Python scripts do and behave according to the passed values.
The argparse module helps to build logic simply so that users can build scripts that require arguments and behave accordingly. It handles how to parse the arguments collected in sys.argv list and automatically generates help and issues error messages when invalid options are given.
In this blog, I am going to show you how you can parse the command line arguments to your python script using the python argparse module.
Let’s start with argparse:
To start using the argparse module, we first have to import it and then Set up the parser object using ArgumentParser() function in the argparse module.
import argparse parser = argparse.ArgumentParser() parser.parse_args()
Save the code into the pythonTest.py
Run the code with the –help option :
python pythonTest.py --help
usage: pythonTest.py [-h]
optional arguments:
-h, --help show this help message and exit
Now we can see that we didn’t specify any help arguments in our script, it’s still giving us a nice help message. The –help parameter is available by default.
Now let us define an argument which is mandatory for the script to run and if not given script should throw an error. Here we define the argument using add_argument() function in the argparse module.
import argparse parser = argparse.ArgumentParser() parser.add_argument("echo") args = parser.parse_args() print(args.echo)
Update the code into the pythonTest.py
Now, you have successfully created your first command line argument with argparse.
-Run the code with –help option:
python pythonTest.py --help
Output:
usage: pythonTest.py [-h] echo
positional arguments:
echo
optional arguments:
-h, --help show this help message and exit
We can see that our echo option in the positional arguments.
-Run the code without option:
python pythonTest.py Output: usage: pythonTest.py [-h] echo pythonTest.py: error: too few arguments
Now, we can see that it gives an error if we do not provide the echo argument value.
-Run the code with echo value:
python pythonTest.py helloWorld!
Output:
helloWorld!
It works fine, we can see the output
So far we have been playing with positional arguments. Let us have a look at how to add optional arguments:
import argparse parser = argparse.ArgumentParser() parser.add_argument("--verbosity", help="increase output verbosity") args = parser.parse_args() if args.verbosity: print "verbosity turned on"
In the above code, we use ‘–verbosity’ as an optional argument, It means that if you do not specify ‘–verbosity’ so it displays noting otherwise it displays something.
-Run the code:
$ python pythonTest.py --verbosity 1 Output: verbosity turned on
$ python pythonTest.py --help Output: usage: pythonTest.py [-h] [--verbosity VERBOSITY] optional arguments: -h, --help show this help message and exit --verbosity VERBOSITY increase output verbosity
Some important points:
-we can give a description of your parser in ArgumentParser() method
parser=argparse.ArgumentParser(description="sample argument parser")
-We can assign default value to an argument in add_argument() method
parser.add_argument("echo", nargs='?', default="HelloWorld")
#Here nargs is the number of command-line arguments that should be consumed.'?'. One argument will be consumed from the command line if possible, and produced as a single item. If no command-line argument is present, the value from default will be produced.
By default, all arguments are treated as strings. To explicitly mention type of argument, use type parameter in the add_argument() method. All Python data types are valid values of type.
parser.add_argument("intValue", type=int)
If it is desired that an argument should value only from a defined list, it is defined as choices parameter.
parser.add_argument("sub", choices=['Physics', 'Maths', 'Biology'])
To get more help about our argument, we can provide the help message.
parser.add_argument("echo", help="echo the string you use here")
Example: Combining Positional and Optional arguments
import argparse
parser = argparse.ArgumentParser()
parser.add_argument("square", type=int,help="display a square of a given number")
parser.add_argument("-v", "--verbose",action="store_true",help="increase output verbosity")
args = parser.parse_args()
answer = args.square**2
if args.verbose:
print "the square of {} equals {}".format(args.square, answer)
else:
print answer
In the above example, we have used both positional and optional arguments,
square is a positional argument which means that we must require the int value to calculate the square.
-v, –verbose is an optional argument which means it is optional, if you specify the -v or –verbose then you will display some message otherwise it will display nothing but will work fine.
Outputs of the above example :
$ python pythonTest.py Output: usage: pythonTest.py [-h] [-v] square pythonTest.py: error: the following arguments are required: square
$ python pythonTest.py 4 Output: 16
$ python pythonTest.py 4 --verbose Output:the square of 4 equals 16
We can see that if we do not give the value of positional arguments then it will give an error but optional argument is optional.
That’s all for now, I will follow it up with more knowledge on this topic next time.
References:
Thank you for sticking to the end. If you like this blog, please do show your appreciation by giving thumbs ups and share this blog and give me suggestions on how I can improve my future posts to suit your needs. Follow me to get updates on different technologies
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK