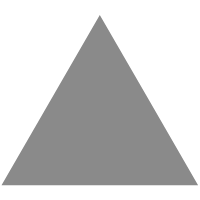
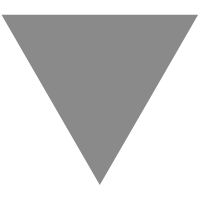
Seg fault error?
source link: https://www.codesd.com/item/seg-fault-error.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Seg fault error?
Does anyone know why I keep getting this seg fault error? I am trying to run a program that locates a substring "from" within "src" and replaces all non-overlapping occurrences of "from" in src with "to" in the output string "dest". Also, could anyone provide me with the proper way to test this case? As I'm not too sure how I could display this with type "void"... (Trying this as an exercise)
void find_replace(char* src, char* from, char* to, char* dest)
{
int count = 0;
int diff = strlen(to) - strlen(from);
int destlength = strlen(src);
dest = malloc(destlength);
for (int i = 0; i < strlen(src); i++)
{
int index = 0;
while (src[i+index] == from[index] && index < strlen(from)){
index++;
}
if (index == strlen(from)) {
for (int j = 0; j < strlen(to); j++) {
dest[i+j+(count * diff)] = to[j];
}
i += strlen(from) - 1;
count++;
}
else {
dest[i + (count * diff)] = src[i];
}
}
return ;
}
Is it sufficient enough to do this for a test?
int main (int argc, char *argv[])
{
char* dest;
find_replace("hello my name is leeho lim", "leeho lim", "(insert name)" dest);
for (int i = 0; i < strlen(dest); i++)
{
printf("%c", dest[i]);
}
printf("\n");
}
The problems happens because you are trying to access an unallocated pointer with
strlen(dest)
In the for in your main program.
The reason for this is that you sent the value of the pointer dest
to the function, not the pointer itself, so when you allocated the memory inside your function, so you didn't actually modify the memory address stored in the pointer outside of it.
When you send the pointer as a parameter of a function, what you are actually doing is sending the memory address stored in that pointer, you are sending the value stored in the pointer, not the pointer itself.
If you want to get the allocated memory address for the string, you either can make the function return it, or you can declare and send dest
as a pointer to a pointer.
EDIT: As the other comment points out, you can also perform the allocation in main()
, instead of doing it inside your function.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK