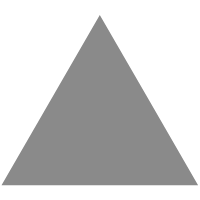
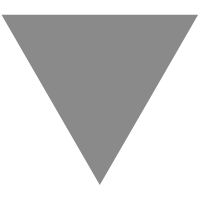
Python Lists
source link: https://rowelldionicio.com/python-day-2-lists/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Python Day 2: Lists
September 10, 2018 By Rowell Leave a Comment
Lists look like they’ll be useful when I get to a point where I can write scripts. Python Lists can hold multiple values in an ordered sequence.
Within the list are items which are ordered by an index. The index starts with 0. Each item is separated by a comma and any strings must have quotes around them.
To assign a list to a variable and retrieve an item from the list the syntax looks like:
[python]
groceries = ['oranges', 'spinach', 'eggs', 'milk']
groceries[0]
'oranges'
[/python]
As I said earlier, the first value in the list starts with 0. It’s possible to go in reverse by using negative indexes:
[python]
groceries = ['oranges', 'spinach', 'eggs', 'milk']
groceries[-1]
'milk'
[/python]
What if you wanted to access multiple values within a list? That’s done by slicing. A slice starts with the first integer, a colon, and the second integer is where the slice ends, but not including that item. Take a look:
[python]
groceries[0:3]
['oranges', 'spinach', 'eggs']
[/python]
Modifying items within a list is possible. Let’s say you wanted to change oranges to apples in the groceries list. We need to modify index 0 within the list.
[python]
groceries[0]
'oranges'
groceries[0] = 'apples'
groceries[0]
'apples'
[/python]
Removing items from a Python list is fairly simple as well by using the del statement. We must reference the index of the item we want to remove.:
[python]
groceries
['apples', 'spinach', 'eggs', 'milk']
del groceries[0]
groceries
['spinach', 'eggs', 'milk']
[/python]
The items in the list move up in their index values which means spinach is now at index 0.
Adding items to the Python list is done by appending it using what’s called a method. Methods are a little different when it comes to lists because we call on the method on a value:
[python]
groceries
['spinach', 'eggs', 'milk']
groceries.append('yogurt')
groceries
['spinach', 'eggs', 'milk', 'yogurt']
[/python]
By appending to the list, the new item we are adding gets added to the end of the list.
It is possible to add items to the list at a specific index. The index value must be passed, with the new item, using the insert method:
[python]
groceries
['spinach', 'eggs', 'milk', 'yogurt']
groceries.insert(0, 'pears')
groceries
['pears', 'spinach', 'eggs', 'milk', 'yogurt']
[/python]
There’s quite a lot you can do with lists. They are very flexible and can be modified with ease. That’s all I’ve done with Day 2 of my Python learning exercise. The next Day will include more Python List work.
On to more practicing…
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK