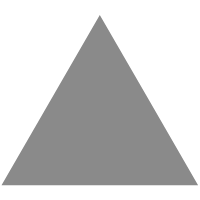
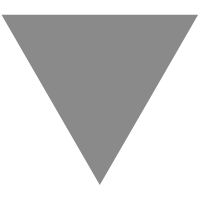
Getting Started with Django
source link: https://blog.knoldus.com/getting-started-with-django/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Getting Started with Django
Reading Time: 4 minutes
Django is a very popular open-source web framework and is easy to pick up for the python beginners and pros. Used by companies such as Mozilla, Instagram, and Spotify; Django is a relevant framework that can help solve a number of problems. Django follows the model-template-view architectural pattern. This differs from the MVC pattern in that Django handles the code that handles interactions between the model and the view. Let’s get into how we can setup Django.
Installation
To install Django we’ll need python and pip. You can follow these steps to install python3, pip3, and django:
sudo apt-get update
sudo apt-get install python3.6
sudo apt install python3-pip
python3 -m pip install Django
Let’s verify Django is installed correctly:
python3 -m django --version
Creating a Simple Web App
Now we’re ready to create our Django app:
django-admin startproject django_starter_app
You’ll be met with a new directory created for you with the following structure:
Basic Django project file structure
- The inner directory is our actual Python package for the project.
- __init__.py: An empty file that tells python that this directory should be considered a package.
- settings.py: Contains various default configuration options for your project. You’re more than welcome to add in your own settings or create global variables here too.
- urls.py: Is where you define routes to your views. We’ll cover more on this in the next section.
- wsgi.py: The entrypoint for WSGI compatible web servers to serve requests to your project.
- manage.py: Command-line program that helps us interact with our Django project. We can use this to start our application from the terminal and run migrations.
Now that we have our Django project setup, and a basic understanding of what the django-admin command created for us, we’ll have to create an app to run on the server. Be sure this run this in the same directory as the manage.py file:
python manage.py startapp myapp
- Our overall structure with the Django project and the app in it should look like the image to the left.
- The newly created views.py is where we’ll be writing our code to handle requests to our server.
Creating a View
In views.py let’s create a function to return an HTTP response from the server. The request will be the first argument to any view, and we can define other URI parameters following it. In the view below I’ve created a path param called name to be expected.
Now we have a server that has a function that could return an HTTP response, but how do we wire this into the Django server? Let’s find out how to route requests to our new view.
Creating a URL
Within our app we’ll have to create a new urls.py file to keep track of the routes we create inside of it. So under django_starter_app/myapp add a file named urls.py. I’m going to define the URL as below:
The first argument pulls the name out of the url, and passes it to our view. The seconds defines the function that the url will form communication with.
Now we have our URL set with the app, we need to configure our server to connect to it. We can define a path for our entire app in the server at the original urls.py that was created for in the directory: django_starter_app/django_starter_app.
Now we have our simple application configured let’s run our server. In the root directory for the Django project run the following manage.py command:
python3 manage.py runserver
We can can visit our application at localhost:8000/myapp/{name}:
And that’s it! The basic flow of django is very easy to understand. It’s easy to implement a service layer and other familiar concepts to handle the logic in your views to keep your code clean and readable.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK