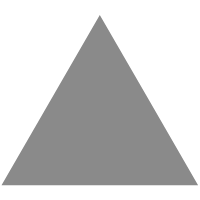
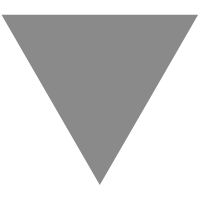
Getting Started with Appium for Android Java on Windows in 10 Minutes
source link: https://www.automatetheplanet.com/getting-started-with-appium-for-android-java-on-windows-in-10-minutes/?utm_campaign=getting-started-with-appium-for-android-java-on-windows-in-10-minutes
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Getting Started with Appium for Android Java on Windows in 10 Minutes
This is the first article from the new series dedicated to the mobile testing using Appium test automation framework. Here, I am going to show you how to configure your machine to test Android applications- prerequisite installations and setup of emulators. After that, you will find how to start your application on the emulator and perform actions on it.
What Is Appium?
Appium is an open source test automation framework for use with native, hybrid and mobile web apps. It drives iOS, Android, and Windows apps using the WebDriver protocol. It is the "standard" for mobile test automation.
Machine Setup
1. Install Java Development Kit (JDK) version 7 or above
(version 8 recommended in order for UI Automation Viewer to work)
2. Set JAVA_HOME environmental variable to where Java JDK is installed
Open in explorer – Control Panel\System and Security\System then click Advanced system settings. Click Environmental Variables.
3. Add Java JDK bin folder to the end of Path environmental variable
4. Install the Android Studio to install the Android SDK at its default location if it is not already installed: C:\Program Files (x86)\Android\android-sdk – follow the following guide
5. Create a virtual device with the Android Virtual Device Manager
6. Install Node.js
7. Install Appium from the command line (skip if you install Appium Desktop)
npm install -g appium
8. Install Appium Desktop (optional)
Find Android App Info
Install APK to Virtual Device
ADB, Android Debug Bridge, is a command-line utility included with Google's Android SDK. ADB can control your device over USB from a computer, copy files back and forth, install and uninstall apps, run shell commands, and more.
First, start the ADB shell using the command – adb shell.
Before automating your app, you may need to expect it and find some info about it. So, you need to install it on your virtual device. To do so, open the command line and execute the following command.
adb install pathToYourApk/yourTestApp.apk
To find the app package and current activity. Open your application on the virtual device and navigate to the desired view. Then open adb shell and use the following command.
dumpsys window windows | grep -E 'mCurrentFocus|mFocusedApp'
Start Android App in Emulator
You need to make sure that the Appium server is started and listening on port 4723.
After the driver is initialised we closed if the app is open. Then before each test, we launch the app and open the desired activity.
Start Appium Service
Instead of starting Appium server manually, we can start it from code.
Get Path to Test App
The apk file is copied from the Resources folder to the compiled binaries. This is how we get the path.
Initialize Desired Capabilities
You need to set the device name to the name of your emulator. In the previous section, I showed you how to find the app package and app activity. If you want to test a native or hybrid app, you have to set the app path. And lastly, add the platform name and version.
Once you have initialised the desired capabilities properly you pass them to the AndroidDriver constructor. If you don't want to start Appium server from code, there is a constructor for passing URL.
Find Android Locators
Using the Android SDK UI Automator Viewer, you can find the elements you are looking for. You can find it in the folder C:\Program Files (x86)\Android\android-sdk\tools\bin. Launch the following file – uiautomatorviewer.bat. Then click on the Device Screenshot and an image of the test app will appear.
Find Android Locators with Appium Desktop
Appium provides you with a neat tool that allows you to find the elements you're looking for. With Appium Desktop you can find any item and its locators by either clicking the element on the screenshot image or locating it in the source tree.
After launching Appium Desktop and starting a session, you can locate any element in the source.
Locating Elements with Appium
- By ID
- By Class
- By XPath
- By AndroidUIAutomator
Locate Elements inside Parent
Gesture Actions in Appium
Swipe
Online Training
NONFUNCTIONAL
START: 16 June 2021
Enterprise Test Automation Framework
LEVEL: 3 (Master Class)
After discussing the core characteristics, we will start writing the core feature piece by piece.
We will continuously elaborate on why we design the code the way it is and look into different designs and compare them. You will have exercises to finish a particular part or extend it further along with discussing design patterns and best practices in programming.
Duration: 30 hours
4 hour per day
-20% coupon code:
BELLATRIX20
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK