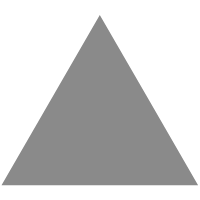
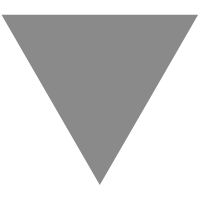
The Power of Axios
source link: https://blog.bitsrc.io/the-power-of-axios-cf45e085d924
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
The Power of Axios
Get to know the features of Axios and how to use them in practice

Axios is a powerful promise-based HTTP client used to make requests to external servers from the browser. If you are a front-end developer, you might already be using this technology.
However, Axios provides a range of advanced features not known by many. Therefore, this article will focus on these features to increase your awareness of its true potential.
- Request and response interception
- Make concurrent requests
- Protection against XSRF
- Transforming data
- Support for upload progress
- Response timeout
- Ability to cancel requests
Request and response interception
This particular feature of Axios is widely spoken about. Therefore, I won’t be doing a detailed explanation. In a nutshell, interceptors allow centrally examining HTTP requests in the browser.
A request interceptor would look like follows.
// declare a request interceptor
axios.interceptors.request.use(config => {
// perform a task before the request is sent
console.log('Request was sent');
return config;
}, error => {
return Promise.reject(error);
});
// GET request
axios.get('https://mysite.com/user')
.then(response => {
console.log('This is printed after ');
});
Whenever a request is sent, the “Request was sent” log will be printed on the console. This is the place where you can perform any operation before any HTTP requests are sent to a server. Similar to this, there are response interceptors as well.
Making concurrent requests
If you need to make multiple network requests at the same time, instead of making them in a sequence, Axios provides a method called Axios.all()
.
Let’s have a look at an example.
class Customer extends Component {
constructor(props) {
super(props); this.state = {
activeCustomer: {
data: '',
permissions: '',
}
}
} getCustomerData = () => axios.get(`${URL}/profile/${this.props.activeCustomerId}`);
getPermissions = () => axios.get(`${URL}/permissions/${this.props.activeCustomerId}`);
async componentDidMount() {
try {
const [customerData, customerPermissions] = await axios.all([ this.getCustomerData(), this.getPermissions() ]);
this.setState(
activeCustomer: {
data: customerData.data,
permissions: customerPermissions.data
}
);
}
catch (error) {
console.log(error);
}
}
}
It allows performing actions after all the concurrent HTTP requests complete.
Tip: Share your reusable components between projects using Bit (Github).
Bit makes it simple to share, document, and reuse independent components between projects. Use it to maximize code reuse, keep a consistent design, collaborate as a team, speed delivery, and build apps that scale.
Bit supports Node, TypeScript, React, Vue, Angular, and more.
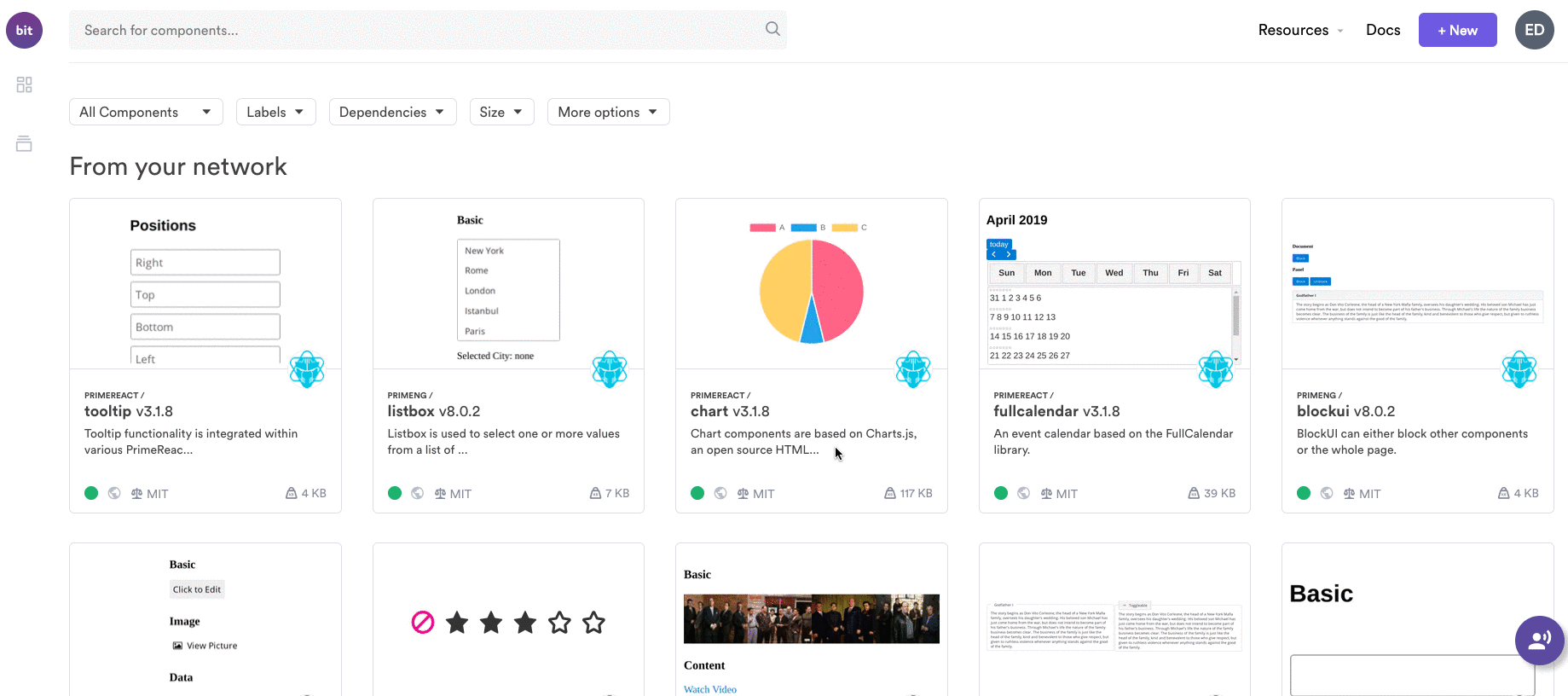
Protection against XSRF
Cross-site request forgery (known as XSRF) is an attacking method for web-hosted applications. When making an XSRF, the attacker disguises as a trusted user to influence the interaction between the application and the browser.
Axios provides a method to avoid such attacks with additional protection while making requests by embedding additional authentication to the request. Using it will help the server to identify the requests from unauthorized locations and blocks them.
The method to add this to Axios is as follows.
const options = {
method: 'post',
url: '/signup',
xsrfCookieName: 'XSRF-TOKEN',
xsrfHeaderName: 'X-XSRF-TOKEN',
};
// send the request
axios(options);
Transforming data
Before a request is made or when a response is received, Axios provides a mechanism to manipulate the data. This is done via two configuration options;
transformRequest
transformResponse
Any functions passed to transformRequest
are applied to PUT, POST, and PATCH requests. transformResponse
is applied to response data before it is passed to the then()
callback.
By default, Axios will convert data to and from JSON in these methods. However, you can customize this by overriding it with your own data transformations.
const options = {
transformRequest: [
(data, headers) => {
// do something with data
return data;
}
]
}
Support for upload progress
Axios allows you to monitor the POST request progress when required. This can be useful when uploading large files to the server. In order to achieve this, you can pass in a request config to Axios.
The request config would look as follows.
const config = {
onUploadProgress: progressEvent => {
console.log(progressEvent.loaded);
}
}
This config object needs to be passed to Axios. The onUploadProgress
function will be called whenever the upload progress changes.
axios.put(`${URL}/upload/image.png`, data, config)
You can even track this on the UI by implementing a progress bar with the use of this.
Response timeout
If a timeout is not set for an HTTP request, any remote end can keep the request waiting for a longer period. With limited resources at hand, this would become a waste. In Axios, the default timeout is set to 0. However, Axios allows you to set a custom timeout when required.
One way to add a timeout is to pass it to the config object.
axios
.post('http://mysite.com/user', { name: 'John' }, { timeout: 2 })
.then(response => {
console.log(response);
})
.catch(error => {
console.log(error);
});
A timeout of 2ms has been set in the above request.
Ability to cancel requests
There can be scenarios where you need to cancel a request that is already sent. Axios allows you to do this using a cancel token. The CancelToken.source
factory provides two main requirements for canceling a request; the cancel token and the cancel()
function. The cancel token can be passed as a config to the axios.get()
function and call the cancel()
method whenever you need to cancel the previous Axios request.
const CancelToken = axios.CancelToken;// create the source
this.source = CancelToken.source();try {
const { data } = await axios.get(`${URL}/posts`, {
cancelToken: this.source.token
});
this.setState({
posts: data
})
} catch (err) {
// check if the request was cancelled
if(axios.isCancel(err)) {
console.log(err.message);
}
console.log(err.message)
}
This feature’s practical usage would be where a user types in a search keyword to retrieve a filtered response.
However, before the response is received, if the user types in another search term, the previous request can be canceled as the response would not be required anymore.
One way to add a timeout is to pass it to the config object.
Summary
As we explored throughout this article, Axios has many advanced features that can be useful for our applications. Most of these features aren’t available in other similar APIs, such as fetch
.
Further, Axios can be used in both the browser and Node.js. This will share the JavaScript code between the browser and the backend or doing a server-side rendering of your front-end applications.
Thanks for reading!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK