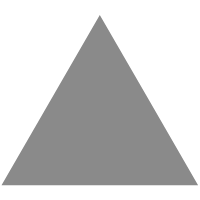
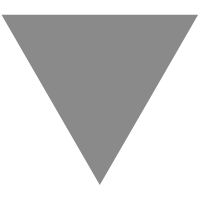
The JavaScript console cheat sheet
source link: https://dev.to/mrwolferinc/the-javascript-console-cheat-sheet-4o76
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
This cheat sheet provides a quick overview of all the methods of the JavaScript console
object. Please note that non-standard methods are not documented.
Basic methods
This section provides information on the basic methods.
console.clear
Clears the console.
Syntax
console.clear();
Parameters
console.debug
Outputs a message to the web console at the "debug" log level.
Syntax
console.debug(obj1 [, obj2, ..., objN]);
console.debug(msg [, subst1, ..., substN]);
Parameters
obj1
... objN
A list of JavaScript objects to output. The string representations of each of these objects are appended together in the order listed and output.
msg
A JavaScript string containing zero or more substitution strings.
subst1
... substN
JavaScript objects with which to replace substitution strings within msg. This gives you additional control over the format of the output.
Example
console.debug('Debug');
console.error
Outputs an error message to the web console.
Syntax
console.error(obj1 [, obj2, ..., objN]);
console.error(msg [, subst1, ..., substN]);
Parameters
obj1
... objN
A list of JavaScript objects to output. The string representations of each of these objects are appended together in the order listed and output.
msg
A JavaScript string containing zero or more substitution strings.
subst1
... substN
JavaScript objects with which to replace substitution strings within msg. This gives you additional control over the format of the output.
Example
console.error('Error');
console.info
Outputs an informational message to the web console.
Syntax
console.info(obj1 [, obj2, ..., objN]);
console.info(msg [, subst1, ..., substN]);
Parameters
obj1
... objN
A list of JavaScript objects to output. The string representations of each of these objects are appended together in the order listed and output.
msg
A JavaScript string containing zero or more substitution strings.
subst1
... substN
JavaScript objects with which to replace substitution strings within msg. This gives you additional control over the format of the output.
Example
console.info('Information');
console.log
Outputs a message to the web console.
Syntax
console.log(obj1 [, obj2, ..., objN]);
console.log(msg [, subst1, ..., substN]);
Parameters
obj1
... objN
A list of JavaScript objects to output. The string representations of each of these objects are appended together in the order listed and output.
msg
A JavaScript string containing zero or more substitution strings.
subst1
... substN
JavaScript objects with which to replace substitution strings within msg. This gives you additional control over the format of the output.
Example
console.log('Message');
console.warn
Outputs a warning message to the web console.
Syntax
console.warn(obj1 [, obj2, ..., objN]);
console.warn(msg [, subst1, ..., substN]);
Parameters
obj1
... objN
A list of JavaScript objects to output. The string representations of each of these objects are appended together in the order listed and output.
msg
A JavaScript string containing zero or more substitution strings.
subst1
... substN
JavaScript objects with which to replace substitution strings within msg. This gives you additional control over the format of the output.
Example
console.warn('Warning');
Advanced methods
This section provides information on the advanced methods.
console.assert
Writes an error message to the web console if the assertion is false.
Syntax
console.assert(assertion, obj1 [, obj2, ..., objN]);
console.assert(assertion, msg [, subst1, ..., substN]);
Parameters
assertion
Any boolean expression. If the assertion is false, the message is written to the console.
obj1
... objN
A list of JavaScript objects to output. The string representations of each of these objects are appended together in the order listed and output.
msg
A JavaScript string containing zero or more substitution strings.
subst1
... substN
JavaScript objects with which to replace substitution strings within msg. This gives you additional control over the format of the output.
Example
for (let num = 2; num <= 5; num++) {
console.log('The number ' + num + ' is even');
console.assert(num % 2 === 0, { number: num, errorMessage: 'The number ' + num + ' is not even' });
}
console.count
Logs the number of times that this particular call to count()
was called.
Syntax
console.count([label]);
Parameters
label
A JavaScript string. If supplied, this method outputs the number of times it has been called with that label. If omitted, count()
behaves as though it was called with the "default" label.
Example
let user = '';
function greet() {
console.count(user);
return 'hi ' + user;
}
user = 'bob';
greet();
user = 'alice';
greet();
greet();
console.count('alice');
console.countReset
Resets the counter used with console.count()
.
Syntax
console.countReset([label]);
Parameters
label
A JavaScript string. If supplied, this method resets the count for that label to 0. If omitted, countReset()
resets the default counter to 0.
Example
let user = '';
function greet() {
console.count(user);
return 'hi ' + user;
}
user = 'bob';
greet();
user = 'alice';
greet();
greet();
console.countReset('bob');
console.count('alice');
console.dir
Displays an interactive list of the properties of a specific JavaScript object.
Syntax
console.dir(object);
Parameters
object
A JavaScript object whose properties should be output.
Example
console.dir(document.location);
console.dirxml
Displays an interactive tree of the descendant elements of the specified XML/HTML element. If it is not possible to display as an element the JavaScript Object view is shown instead.
Syntax
console.dirxml(object);
Parameters
object
A JavaScript object whose properties should be output.
console.group
Creates a new inline group in the web console log. This indents following console messages by an additional level until console.groupEnd()
is called.
Syntax
console.group([label]);
Parameters
label
Label for the group. This does not work with console.groupEnd
.
Example
console.log('This is the outer level');
console.group();
console.log('Level 2');
console.group();
console.log('Level 3');
console.info('More of level 3');
console.groupEnd();
console.log('Back to level 2');
console.groupEnd();
console.log('Back to the outer level');
console.groupCollapsed
Creates a new inline group in the web console. Unlike console.group()
, the new group is created collapsed.
Syntax
console.groupCollapsed([label]);
Parameters
label
Label for the group.
console.groupEnd
Exits the current inline group in the web console.
Syntax
console.groupEnd();
Parameters
console.table
Displays tabular data as a table.
Syntax
console.table(data);
console.table(data, columns);
Parameters
data
The data to display. This must be either an array or an object.
columns
An array containing the names of columns to include in the output.
Example
// An object
console.table({ firstName: 'John', lastName: 'Doe' });
// An array
console.table(['Apples', 'Oranges', 'Bananas']);
// An array of objects
let city1 = { name: 'New York', state: 'New York' };
let city2 = { name: 'Chicago', state: 'Illinois' };
let city3 = { name: 'Los Angeles', state: 'California' };
console.table([city1, city2, city3]);
console.time
Starts a timer that you can use to track how long an operation takes.
Syntax
console.time(label);
Parameters
label
The name to give the new timer. This will identify the timer; use the same name when calling console.timeEnd()
to stop the timer and get the time output to the console.
Example
console.time('answer time');
alert('Click to continue');
console.timeLog('answer time');
alert('Do a bunch of other stuff...');
console.timeEnd('answer time');
console.timeEnd
Stops a timer that was previously started by calling console.time()
.
Syntax
console.timeEnd(label);
Parameters
label
The name of the timer to stop. Once stopped, the elapsed time is automatically outputted to the web console.
console.timeLog
Logs the current value of a timer that was previously started by calling console.time()
to the console.
Syntax
console.timeLog(label);
Parameters
label
The name of the timer to log to the console.
console.trace
Outputs a stack trace to the web console.
Syntax
console.trace( [...any, ...data]);
Parameters
...any, ...data
Zero or more objects to be output to console along with the trace. These are assembled and formatted the same way they would be if passed to the console.log()
method.
Example
function foo() {
function bar() {
console.trace();
}
bar();
}
foo();
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK