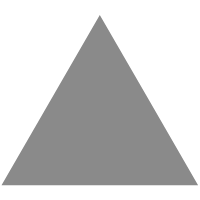
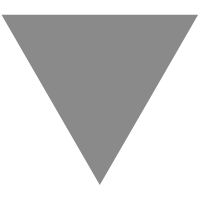
web.js 使用教程第二篇(version:1.3.5)- 合约交互,发送ETH,发送ERC20代币
source link: https://learnblockchain.cn/article/2369
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
web.js 使用教程第二篇(version:1.3.5)- 合约交互,发送ETH,发送ERC20代币
vue项目中使用web3.js 学会如何用最新的web3.js版本
web3.js API参考文档:API文档
在web3.js 大于1.0的版本中,也就是目前的通过metamask获取web对象的方法
构建web3对象
//在和以太坊兼容的浏览器中使用 web3.js 时,当前环境的原生 provider 会被浏览器设置
//例如安装了MetaMask,它在浏览器中注入了window.ethereum下的提供者对象,我们就可以通过window.ethereum来初始化web3对象
var web3Provider;
if (window.ethereum) {
web3Provider = window.ethereum;
try {
// 请求用户授权
await window.ethereum.enable();
} catch (error) {
//用户不授权时,这里处理异常情况,同时可以设置重试等机制
console.log(error)
}
} else if (window.web3) {// 老版 MetaMask Legacy dapp browsers...
web3Provider = window.web3.currentProvider;
} else {
// 这里处理连接在不支持dapp的地方打开的情况
web3Provider = new Web3.providers.HttpProvider('https://eth-testnet.tokenlon.im');
console.log("Non-Ethereum browser detected. You should consider trying MetaMask!")
//这种情况下表示用户在不支持dapp的浏览器打开,无法初始化web3
//这里一般的处理逻辑是:使用第一篇中的那种自己初始化,获得区块上的基础数据,但是没法获取用户的账户信息
//或者直接提示错误,不进行任何处理
}
this.web3 = new Web3(web3Provider);
初始化后就可以通过web3对象进行相关操作
获取账户地址
console.log("获取账户地址Coinbase", this.web3.eth.getCoinbase());
获取metamask中连接的所有账户
console.log(this.web3.eth.getAccounts());
获取网络ID,用来区分在测试网还是正式网
console.log("获取链 ID",this.web3.eth.net.getId())
console.log("ChainId获取链 ID",this.web3.eth.getChainId())this.web3.eth.net.getId((err, netID) => {
// Main Network: 1 表示主网
// Ropsten Test Network: 3 //测试网Ropsten
// Kovan Test Network: 42 //测试网Kovan
// Rinkeby Test Network: 4 //测试网Rinkeby
console.log(netID)});
查询当前区块高度
console.log("查询当前区块高度", this.web3.eth.getBlockNumber())
this.web3.eth.getBlockNumber().then(console.log);
查询某个地址的余额,单位是wei
this.web3.eth.getBalance("0xFedC0F66dDba0a7EE51F47b894Db35b222b0EF42").then(console.log);
获取当前metamask账户地址的eth余额
this.web3.eth.getCoinbase((err, coinbase) =>{
this.web3.eth.getBalance(coinbase).then(console.log);
})
通过hash查询交易
console.log("查询交易",this.web3.eth.getTransaction('0x0f77813b2f252b0b189824bcac09d19d0d8e0a3ae506dfbb0a90906d115d4d2c'))
查询交易Receipt,一般通过这里的status来判断交易是否成功
console.log("查询交易receipt",this.web3.eth.getTransactionReceipt('0x0f77813b2f252b0b189824bcac09d19d0d8e0a3ae506dfbb0a90906d115d4d2c'))
发送ETH相关
发送交易ETH
说明:from是你metamask里的账户地址
//发送交易https://learnblockchain.cn/docs/web3.js/web3-eth.html#sendtransaction
this.web3.eth.sendTransaction({
from:'0xFedC0F66dDba0a7EE51F47b894Db35b222b0EF42',//如果from为空,默认取this.web3.eth.defaultAccount
to:'0x96B4250b8F769Ed413BFB1bb38c5d28C54f81618',
value:10000000000000000, //发送的金额,这里是0.01 ether
gas: 21000 //一个固定值,可选
})
发送一笔eth转账(使用回调)
this.web3.eth.sendTransaction({
from:'0xFedC0F66dDba0a7EE51F47b894Db35b222b0EF42',
to:'0x96B4250b8F769Ed413BFB1bb38c5d28C54f81618',
value:10000000000000000,
gas: 21000 //一个固定值
}, function(error, hash){
if (error){
console.log("发送交易失败", error)
}else {
console.log("发送交易成功,hash:", hash)
}
});
发送一笔eth转账(使用 promise)
this.web3.eth.sendTransaction({
from:'0xFedC0F66dDba0a7EE51F47b894Db35b222b0EF42',
to:'0x96B4250b8F769Ed413BFB1bb38c5d28C54f81618',
value:10000000000000000
}).then(function (receipt) {
//待区块确认后会回调,通过receipt判断交易是否成功
console.log(receipt)
console.log("交易状态:", receipt.status)
});
使用事件发生器
this.web3.eth.sendTransaction({
from:'0xFedC0F66dDba0a7EE51F47b894Db35b222b0EF42',
to:'0x96B4250b8F769Ed413BFB1bb38c5d28C54f81618',
value: '10000000000000000'
}).on('transactionHash', function(hash){
console.log("发送成功,获取交易hash:",hash)
}).on('receipt', function(receipt){
console.log("链上结果返回,返回数据:",receipt)
}).on('confirmation', function(confirmationNumber, receipt){
console.log("链上confirmation结果返回,确认数:",confirmationNumber)
console.log("链上confirmation结果返回,返回数据:",receipt)
}).on('error', console.error); // 如果是 out of gas 错误, 第二个参数为交易收据
ERC20相关
获取erc20代币余额
导入的ABI文件,这个就是区块浏览器https://kovan.etherscan.io/address/0x78616d23e97967ee072f21e82864f55826f674bb#code里的Contract ABI的内容
新建一个WZGLTokenABI.json,里面的内容就是Contract ABI
获取erc20代币余额
//导入erc20代币合约的ABI文件
import WZGLTokenABI from '../ABI/WZGLTokenABI.json'
//发送代币的地址
var from = "0xFedC0F66dDba0a7EE51F47b894Db35b222b0EF42";
//接收代币的地址
var to = "0x96B4250b8F769Ed413BFB1bb38c5d28C54f81618";
//erc20代币合约地址: 我自己在kovan发行的一个代币0x78616D23E97967eE072f21e82864F55826F674Bb
var contractAddress = "0x78616D23E97967eE072f21e82864F55826F674Bb";
//WZGLTokenABI在前面已经导入
console.log(WZGLTokenABI)
var WZGLToken = new this.web3.eth.Contract(WZGLTokenABI, contractAddress,{
from: '0xFedC0F66dDba0a7EE51F47b894Db35b222b0EF42'
});
//获取代币余额
WZGLToken.methods.balanceOf(from).call({
from: from
}, function call(error, result){
if (!error) {
console.log("balanceOf", result)
} else {
console.log(error)
}
});
发送erc20代币
//发送代币的地址
var from = "0xFedC0F66dDba0a7EE51F47b894Db35b222b0EF42";
//接收代币的地址
var to = "0x96B4250b8F769Ed413BFB1bb38c5d28C54f81618";
//erc20代币合约地址: 我自己在kovan发行的一个代币0x78616D23E97967eE072f21e82864F55826F674Bb
var contractAddress = "0x78616D23E97967eE072f21e82864F55826F674Bb";
//WZGLTokenABI在前面已经导入
console.log(WZGLTokenABI)
var WZGLToken = new this.web3.eth.Contract(WZGLTokenABI, contractAddress,{
from: from
});
//发送一个代币
WZGLToken.methods.transfer(to, '1000000000000000000').send({
from: from
}, function(error, transactionHash){
if (!error) {
console.log("交易hash:", transactionHash)
} else {
console.log(error)
}
}).then(function (receipt) {//监听后续的交易情况
console.log(receipt)
console.log("交易状态:", receipt.status)
});
和其他的Dapp合约交互的逻辑和这个erc20转账是类似的
通过ABI和合约地址参数化合约对象,然后通过合约对象进行合约调用
var myContract = new web3.eth.Contract(abi, 合约地址);
//toPay是合约里的一个方法
myContract.methods.toPay().send({from: '0x...'});
具体的可以参考文档:https://learnblockchain.cn/docs/web3.js/web3-eth-contract.html#methods-mymethod-send
本文参与登链社区写作激励计划 ,好文好收益,欢迎正在阅读的你也加入。
- 发表于 14小时前
- 阅读 ( 35 )
- 学分 ( 0 )
- 分类:DApp
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK