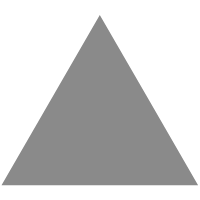
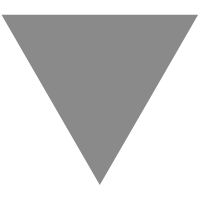
JAVA Socket编程:使用HTTP实现网络通信
source link: https://arminli.com/java-socket-http/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
JAVA Socket编程:使用HTTP实现网络通信
March 22, 2016
服务器功能
提供 HTML,JPG 等 MIME 类型的资源。
客户端功能
访问服务器,获取 HTML 和 JPG 资源,保存到本地磁盘。
预习内容:HTTP通信原理
服务器端代码
TaskThread.java
package httpServer;
import java.io.BufferedReader; import java.io.DataOutputStream; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStreamReader; import java.io.PrintStream; import java.net.Socket;
public class TaskThread extends Thread{ private Socket s; public TaskThread(Socket s){ this.s = s; } public void run(){ BufferedReader reader; PrintStream writer; FileInputStream in; DataOutputStream os; String firstLineOfRequest; try { reader = new BufferedReader(new InputStreamReader(s.getInputStream())); firstLineOfRequest = reader.readLine(); //System.out.println(firstLineOfRequest); String uri = firstLineOfRequest.split(” “)[1]; writer = new PrintStream(s.getOutputStream()); File file = new File(“D:/http”+uri); if(file.exists()){ writer.println(“HTTP/1.1 200 OK”);//返回应答消息,并结束应答 if(uri.endsWith(“.html”)) { writer.println(“Content-Type:text/html”); } else if(uri.endsWith(“.jpg”)) { writer.println(“Content-Type:image/jpeg”); }else { writer.println(“Content-Type:application/octet-stream”); } in = new FileInputStream(“D:/http”+uri); //发送响应头 writer.println(“Content-Lenth:“+in.available()); writer.println(); writer.flush(); //发送响应体 os = new DataOutputStream(s.getOutputStream()); byte[]b=new byte[1024]; int len = 0; len = in.read(b); while(len!=-1) { os.write(b,0,len); len=in.read(b); } os.flush(); writer.close(); }else{ //发送响应头 writer.println(“HTTP/1.1 404 Not Found”); writer.println(“Content-Type:text/plain”); writer.println(“Content-Length:7”); writer.println(); //发送响应体 writer.print(“访问内容不存在”); writer.flush(); writer.close(); } } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
server.java
package httpServer;
import java.io.IOException; import java.net.ServerSocket; import java.net.Socket;
public class server { public static void main(String[]args) { ServerSocket ss=null; try { ss=new ServerSocket(8888); } catch (IOException e) { // TODO 自动生成的 catch 块 e.printStackTrace(); } while(true) { try { Socket s = ss.accept(); TaskThread t = new TaskThread(s); t.start(); } catch (IOException e) { // TODO 自动生成的 catch 块 e.printStackTrace(); } } } }
服务器端写完后可以启动后在浏览器中测试一下如:
输入 http://localhost:8888/login.html
输入 http://localhost:8888/ios.jpg
客户端代码
Client.java
package httpClient;
import java.io.BufferedReader; import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.io.PrintStream; import java.net.Socket; import java.net.UnknownHostException; import java.util.Scanner;
public class Client {
public static void main(String[] args) throws UnknownHostException, IOException {
// TODO Auto-generated method stub
Scanner scanner= new Scanner(System.in);
//连接服务器
Socket s = new Socket("localhost", 8888);
//发送请求头
PrintStream writer = new PrintStream(s.getOutputStream());
System.out.println("请输入filename:");
String filename = scanner.nextLine();
writer.println("GET /"+filename+" HTTP/1.1");
writer.println("Host:localhost");
writer.println("connetion:keep-alive");
writer.println();
writer.flush();
//接受响应状态
InputStream in=s.getInputStream();
BufferedReader reader=new BufferedReader(new InputStreamReader(in));
String firstLineOfResponse = reader.readLine();
String secondLineOfResponse = reader.readLine();
String threeLineOfResponse = reader.readLine();
if(firstLineOfResponse.endsWith("OK")) {
System.out.println("开始传输\n");
byte[] b = new byte[1024];
FileOutputStream out= new FileOutputStream("D:/http/h"+"/"+filename);
int len = in.read(b);
while(len!=-1) {
out.write(b,0,len);
len=in.read(b);
}
System.out.println("传输完成\n");
in.close();
out.close();
}
else {
//output error message
System.out.println("该文件不存在\n");
StringBuffer result = new StringBuffer();
String line;
while((line=reader.readLine())!=null) {
result.append(line);
}
reader.close();
System.out.println(result);
}
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK