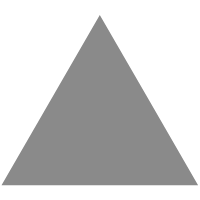
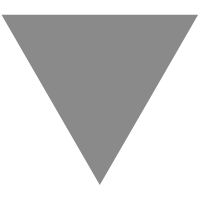
Test that a component does not render when it has passed a certain prop value wi...
source link: https://www.codesd.com/item/test-that-a-component-does-not-render-when-it-has-passed-a-certain-prop-value-with-the-enzyme.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Test that a component does not render when it has passed a certain prop value with the enzyme
I am wanting to test if my LoadingIndicator component loads into the DOM when the status property is set to FETCHING and doesn't load when it is READY. Right now I have this:
import React from 'react';
import { assert } from 'chai';
import { shallow, mount } from 'enzyme';
import LoadingIndicator from '../../src/components/LoadingIndicator';
import { STATUSES } from '../../src/reducers/status';
describe('LoadingIndicator', () => {
it('should render when the status is FETCHING', () => {
const wrapper = shallow(<LoadingIndicator status={STATUSES.FETCHING} />);
assert.ok(wrapper);
});
it('should not render when the status is READY', () => {
const wrapper = mount(<LoadingIndicator status={STATUSES.READY} />);
assert.isNotOkay(wrapper);
});
});
The component itself looks like this:
import React, { PropTypes } from 'react';
import CircularProgress from 'material-ui/CircularProgress';
import { STATUSES } from '../reducers/status';
import '../styles/LoadingIndicator.scss';
export default class LoadingIndicator extends React.Component {
render() {
return (
this.props.status === STATUSES.READY ? null :
<div className="loader-div">
<CircularProgress className="loader" />
</div>
);
}
}
LoadingIndicator.propTypes = {
status: PropTypes.string.isRequired,
};
This doesn't seem to be the correct way to check if it renders or not though, I get
1) LoadingIndicator should not render when the status is READY:
AssertionError: expected { Object (component, root, ...) } to be falsy
Any idea how I can properly test if this component loads depending on the property it is given?
Thanks.
Hi so I ended up figuring this out myself. I should have thought about the fact that since I'm doing a shallow render, I am able to just look if the div renders or not, so I end up with:
import React from 'react';
import { expect } from 'chai';
import { shallow } from 'enzyme';
import LoadingIndicator from '../../src/components/LoadingIndicator';
import { STATUSES } from '../../src/reducers/status';
describe('LoadingIndicator', () => {
it('should render when the status is FETCHING', () => {
const wrapper = shallow(<LoadingIndicator status={STATUSES.FETCHING} />);
expect(wrapper.find('div')).to.have.length(1);
});
it('should not render when the status is READY', () => {
const wrapper = shallow(<LoadingIndicator status={STATUSES.READY} />);
expect(wrapper.find('div')).to.have.length(0);
});
});
and that works (tested for both positive/negative on both).
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK