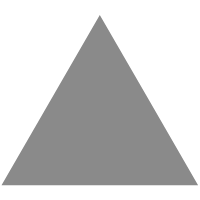
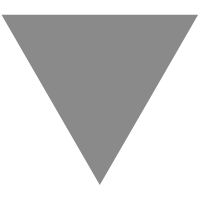
How to Interact with Business Processes Using Camel Routes
source link: https://blog.kie.org/2021/04/how-to-interact-with-business-processes-using-camel-routes.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
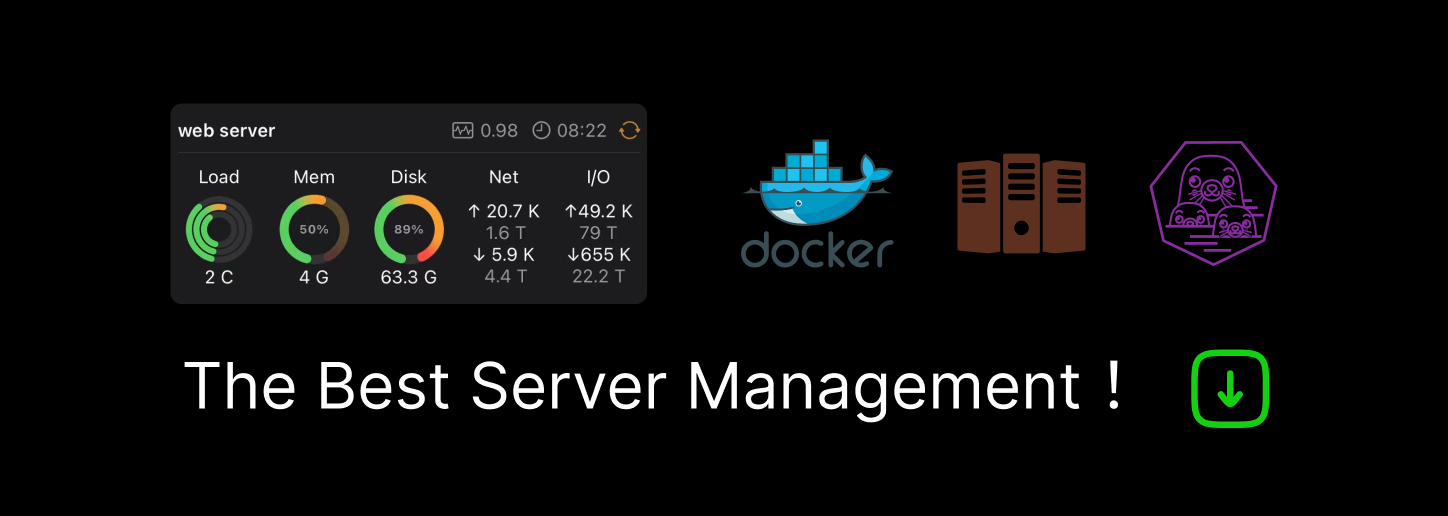
How to Interact with Business Processes Using Camel Routes
The JBPM KIE server has a rich set of REST APIs that allows control over business processes and other business assets. Interaction with business processes is straightforward and easily accessible. Usually these APIs are used by the Business Central component and custom applications. In this post I want to give a simple example of how to interact with business processes, deployed in a KIE server, using Apache Camel. Utilizing business processes in a camel route, as you will see, is pretty easy!
There is an available component in Camel called simply the JBPM component. Both consumers and producers are supported. The consumer side has already been covered by Maciej in this excellent post. My example will focus on the producer side.
What interactions are available?
The JBPM component uses exchange headers to hold the operations/commands used to interact with the KIE server. The query parameters in the URL holds the security and deployment information. The following is an example of an JBPM URL.
jbpm:http://localhost:8080/kie-server/services/rest/server?userName=myuser&password=mypass&deploymentId=mydeployment
That URL above will interact with the KIE server running on localhost port 8080, the container deployed as mydeployment, and using the user myuser and password mypass. Of course all these values can be parameterized.
The following table shows some of the interactions available as of version Apache Camel 3.8. The header key for the operation header is JBPMConstants.OPERATION. All supported header keys are listed in the JBPMConstants class.
OperationSupport HeadersDescriptionstartProcessPROCESS_IDPARAMETERSStart a process in the deployed container (in URL) and the process definition ID and any parameters.signalEventPROCESS_INSTANCE_ID
EVENT_TYPE
EVENTSignal a process instance with the signal (EVENT_TYPE) and payload (EVENT). If process instance ID is missing then the signal scope will be defaultgetProcessInstancePROCESS_INSTANCE_IDRetrieve the process instance using the ID. The resulting exchange body will be populated by a
org.kie.server.api.model.instance.ProcessInstance
object.completeWorkItemPROCESS_INSTANCE_IDWORK_ITEM_ID
PARAMETERSComplete the work item using the parameters.
You can find the complete list in the org.apache.camel.component.jbpm.JBPMProducer.Operation
enum.
Simple Example
In this example we’ll be using the following versions:
Install and start the JBPM KIE server.
> docker pull jboss/jbpm-server-full
> docker run -p 8080:8080 -p 8001:8001 -d --name jbpm-server-full jboss/jbpm-server-full:latest
Log into Business Central (http://localhost:8080/business-central/) using wbadmin/wbadmin as the credentials. In the default MySpace, create a project call test-camel and a business process in it call test-camel-process. Add a process variable call data of type String. Add a script task to print out data. Save and deploy the project.
To quickly stand up a Camel environment, we’ll be using Spring Boot. The following command will help you create the spring boot project:
mvn archetype:generate \
-DarchetypeGroupId=org.apache.camel.archetypes \
-DarchetypeArtifactId=camel-archetype-spring-boot \
-DarchetypeVersion=3.8.0
In the resulting project, add the following dependency into the pom.xml file.
<dependency>
<groupId>org.apache.camel.springboot</groupId>
<artifactId>camel-jbpm-starter</artifactId>
</dependency>
Add the following to the application.properties file.
server.port = 8280
jbpm.server.url = http://localhost:8080/kie-server/services/rest/server
jbpm.server.user = wbadmin
jbpm.server.pass = wbadmin
jbpm.deployment.id = test-camel_1.0.0-SNAPSHOT
jbpm.process.id = test-camel.test-camel-process
jbpm.process.data = data
Modify the MySpringBootRouter.java file to add some routes. The following code snippet will start a process.
@Override public void configure() { from("timer:startprocess?delay=1000&period=5000") .to("direct:startprocess"); from("direct:startprocess") .setHeader(JBPMConstants.OPERATION, constant("CamelJBPMOperationstartProcess")) .setHeader(JBPMConstants.PROCESS_ID, simple("{{jbpm.process.id}}")) .process((Exchange exchange) -> { String dataName = exchange.getContext().resolvePropertyPlaceholders("{{jbpm.process.data}}"); Map<String, Object> params = new HashMap<>(); params.put(dataName, "hello world"); exchange.getIn().setHeader(JBPMConstants.PARAMETERS, params); }) .to("jbpm:{{jbpm.server.url}}?userName={{jbpm.server.user}}&password={{jbpm.server.pass}}&deploymentId={{jbpm.deployment.id}}"); }
The above example will send "hello world" as the data to start the process in the KIE server. By default without specifying JBPMConstants.OPERATION
, the operation will be to start the process. The reason the operation is set to CamelJBPMOperationstartProcess is the operation is an aggregation of the value of JBPMConstants.OPERATION
and the enum value defined in org.apache.camel.component.jbpm.JBPMProducer.Operation
. Unfortunately we cannot use the operation enum directly because it’s not exposed publicly.
Recommend
-
10
Article Leader election in Kubernetes using Apache Camel ...
-
10
What is Apache Camel? As described by the creator itself, Claus Ibsen on Apache Camel in Action, 2nd edition: At the core of the...
-
6
Implementing the Filter EIP using Camel-k and KogitoApache Camel is an Open Source integration framework that supports the implement...
-
9
Integrate Apache ActiveMQ brokers using Camel K Skip to main content ...
-
13
Exception Handling in Apache Camel using Spring Reading Time: 3 minutes Apache Camel is a rule-based routing and mediation engine that provides a Java object-based implementation of the Enterpr...
-
2
Build reactive apps on Kubernetes using Camel K Skip to main content ...
-
5
A recent addition to the Kogito Serverless Workflow is the ability to call Camel Routes from your workflow!
-
3
Using System.Text.Json for Camel Case Serialization Posted by Code Maze | May 25, 2023 |
-
5
Camel Routes support in Serverless Workflow Editors Camel Routes support in Serverless Workflow EditorsThe...
-
6
How to leverage AI to generate Apache Camel routes Skip to main content ...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK