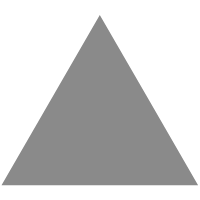
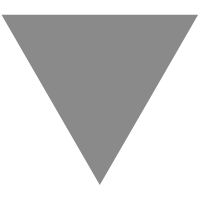
Github GitHub - azjezz/psl: PHP Standard Library - a modern, consistent, central...
source link: https://github.com/azjezz/psl
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
Psl - PHP Standard Library
Psl is a standard library for PHP, inspired by hhvm/hsl.
The goal of Psl is to provide a consistent, centralized, well-typed set of APIs for PHP programmers.
Example
<?php declare(strict_types=1); use Psl\{Str, Vec}; /** * @psalm-param iterable<?int> $codes */ function foo(iterable $codes): string { $codes = Vec\filter_nulls($codes); $chars = Vec\map($codes, fn(int $code): string => Str\chr($code)); return Str\join($chars, ', '); } foo([95, 96, null, 98]); // 'a, b, d'
Installation
Supported installation method is via composer:
$ composer require azjezz/psl
Psalm Integration
PSL comes with a Psalm plugin, that improves return type for PSL functions that psalm cannot infer from source code.
To enable the Psalm plugin, add the Psl\Integration\Psalm\Plugin
class to your psalm configuration file plugins list as follows:
<psalm> ... <plugins> ... <pluginClass class="Psl\Integration\Psalm\Plugin" /> </plugins> </psalm>
Documentation
You can read through the API documentation in docs/README.md
.
Principles
- All functions should be typed as strictly as possible
- The library should be internally consistent
- References may not be used
- Arguments should be as general as possible. For example, for
array
functions, preferiterable
inputs where practical, falling back toarray
when needed. - Return types should be as specific as possible
- All files should contain
declare(strict_types=1);
Consistency Rules
This is not exhaustive list.
- Functions argument order should be consistent within the library
- All iterable-related functions take the iterable as the first argument ( e.g.
Iter\map
andIter\filter
) $haystack
,$needle
, and$pattern
are in the same order for all functions that take them
- All iterable-related functions take the iterable as the first argument ( e.g.
- Functions should be consistently named.
- If an operation can conceivably operate on either keys or values, the default is to operate on the values - the version that operates on keys should have
_key
suffix (e.g.Iter\last
,Iter\last_key
,Iter\contains
,Iter\contains_key
) - Find-like operations that can fail should return
?T
; a second function should be added with anx
suffix that uses an invariant to returnT
(e.g.Arr\last
,Arr\lastx
) - Iterable functions that do an operation based on a user-supplied keying function for each element should be suffixed with
_by
(e.g.Arr\sort_by
,Iter\group_by
,Math\max_by
)
Sponsors
Thanks to our sponsors and supporters:
JetBrains
License
The MIT License (MIT). Please see LICENSE
for more information.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK