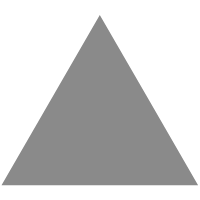
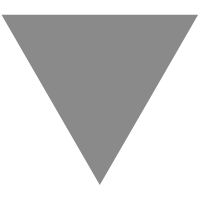
5个简单的步骤掌握Tensorflow的Tensor
source link: https://panchuang.net/2020/10/19/5%e4%b8%aa%e7%ae%80%e5%8d%95%e7%9a%84%e6%ad%a5%e9%aa%a4%e6%8e%8c%e6%8f%a1tensorflow%e7%9a%84tensor/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
作者|Orhan G. Yalçın
编译|VK
来源|Towards Datas Science
如果你正在读这篇文章,我相信我们有着相似的兴趣,现在/将来也会从事类似的行业。
在这篇文章中,我们将深入研究Tensorflow Tensor的细节。我们将在以下五个简单步骤中介绍与Tensorflow的Tensor中相关的所有主题:
-
第一步:张量的定义→什么是张量?
-
第二步:创建张量→创建张量对象的函数
-
第三步:张量对象的特征
-
第四步:张量操作→索引、基本张量操作、形状操作、广播
-
第五步:特殊张量
张量的定义:什么是张量
张量是TensorFlow的均匀型多维数组。它们非常类似于NumPy数组,并且它们是不可变的,这意味着一旦创建它们就不能被更改。只能使用编辑创建新副本。
让我们看看张量如何与代码示例一起工作。但是首先,要使用TensorFlow对象,我们需要导入TensorFlow库。我们经常将NumPy与TensorFlow一起使用,因此我们还可以使用以下行导入NumPy:
import tensorflow as tf
import numpy as np
张量的创建:创建张量对象
有几种方法可以创建tf.Tensor对象。让我们从几个例子开始。可以使用多个TensorFlow函数创建张量对象,如下例所示:
# 你可以用`tf.constant`函数创建tf.Tensor对象:
x = tf.constant([[1, 2, 3, 4 ,5]])
# 你可以用`tf.ones`函数创建tf.Tensor对象:
y = tf.ones((1,5))
# 你可以用`tf.zeros`函数创建tf.Tensor对象:
z = tf.zeros((1,5))
# 你可以用`tf.range`函数创建tf.Tensor对象:
q = tf.range(start=1, limit=6, delta=1)
print(x)
print(y)
print(z)
print(q)
输出:
tf.Tensor([[1 2 3 4 5]], shape=(1, 5), dtype=int32)
tf.Tensor([[1. 1. 1. 1. 1.]], shape=(1, 5), dtype=float32)
tf.Tensor([[0. 0. 0. 0. 0.]], shape=(1, 5), dtype=float32)
tf.Tensor([1 2 3 4 5], shape=(5,), dtype=int32)
如你所见,我们使用三个不同的函数创建了形状(1,5)的张量对象,使用tf.range()函数创建了形状(5,)的第四个张量对象。注意,tf.ones的和tf.zeros接受形状作为必需的参数,因为它们的元素值是预先确定的。
张量对象的特征
tf.Tensor创建对象,它们有几个特征。首先,他们有维度数量。其次,它们有一个形状,一个由维度的长度组成的列表。所有张量都有一个大小,即张量中元素的总数。最后,它们的元素都被记录在一个统一的数据类型(datatype)中。让我们仔细看看这些特征。
张量根据其维数进行分类:
-
Rank-0(标量)张量:包含单个值且没有轴的张量(0维);
-
Rank-1张量:包含单轴(一维)值列表的张量;
-
Rank-2张量:包含2个轴(2维)的张量;以及
-
Rank-N张量:包含N轴的张量(三维)。
例如,我们可以通过向tf.constant传递一个三层嵌套的list对象来创建一个Rank-3张量。对于这个例子,我们可以将数字分割成一个3层嵌套的列表,每个层有3个元素:
three_level_nested_list = [[[0, 1, 2],
[3, 4, 5]],
[[6, 7, 8],
[9, 10, 11]] ]
rank_3_tensor = tf.constant(three_level_nested_list)
print(rank_3_tensor)
Output:
tf.Tensor( [[[ 0 1 2]
[ 3 4 5]]
[[ 6 7 8]
[ 9 10 11]]],
shape=(2, 2, 3), dtype=int32)
我们可以查看“rank_3_tensor”对象当前具有“.ndim”属性的维度数。
tensor_ndim = rank_3_tensor.ndim
print("The number of dimensions in our Tensor object is", tensor_ndim)
Output:
The number of dimensions in our Tensor object is 3
形状特征是每个张量都具有的另一个属性。它以列表的形式显示每个维度的大小。我们可以查看使用.shape属性创建的rank_3_tensor对象的形状,如下所示:
tensor_shape = rank_3_tensor.shape
print("The shape of our Tensor object is", tensor_shape)
Output:
The shape of our Tensor object is (2, 2, 3)
如你所见,我们的张量在第一层有两个元素,第二层有两个元素,第三层有三个元素。
大小是张量的另一个特征,它意味着张量有多少个元素。我们不能用张量对象的属性来测量大小。相反,我们需要使用tf.size函数。最后,我们将使用实例函数.NumPy()将输出转换为NumPy,以获得更具可读性的结果:
tensor_size = tf.size(rank_3_tensor).numpy()
print("The size of our Tensor object is", tensor_size)
Output:
The size of our Tensor object is 12
张量通常包含数字数据类型,如浮点和整数,但也可能包含许多其他数据类型,如复数和字符串。
但是,每个张量对象必须将其所有元素存储在一个统一的数据类型中。因此,我们还可以使用.dtype属性查看为特定张量对象选择的数据类型,如下所示:
tensor_dtype = rank_3_tensor.dtype
print("The data type selected for this Tensor object is", tensor_dtype)
Output:
The data type selected for this Tensor object is <dtype: 'int32'>
索引是项目在序列中位置的数字表示。这个序列可以引用很多东西:一个列表、一个字符串或任意的值序列。
TensorFlow还遵循标准的Python索引规则,这类似于列表索引或NumPy数组索引。
关于索引的一些规则:
-
索引从零(0)开始。
-
负索引(“-n”)值表示从末尾向后计数。
-
冒号(“:”)用于切片:开始:停止:步骤。
-
逗号(“,”)用于达到更深层次。
让我们用以下几行创建rank_1_tensor:
single_level_nested_list = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
rank_1_tensor = tf.constant(single_level_nested_list)
print(rank_1_tensor)
Output:
tf.Tensor([ 0 1 2 3 4 5 6 7 8 9 10 11],
shape=(12,), dtype=int32)
测试一下我们的规则1,2,3:
# 规则1,索引从0开始
print("First element is:",
rank_1_tensor[0].numpy())
# 规则2,负索引
print("Last element is:",
rank_1_tensor[-1].numpy())
# 规则3,切片
print("Elements in between the 1st and the last are:",
rank_1_tensor[1:-1].numpy())
Output:
First element is: 0
Last element is: 11
Elements in between the 1st and the last are: [ 1 2 3 4 5 6 7 8 9 10]
现在,让我们用以下代码创建rank_2_tensor:
two_level_nested_list = [ [0, 1, 2, 3, 4, 5], [6, 7, 8, 9, 10, 11] ]
rank_2_tensor = tf.constant(two_level_nested_list)
print(rank_2_tensor)
Output:
tf.Tensor( [[ 0 1 2 3 4 5]
[ 6 7 8 9 10 11]], shape=(2, 6), dtype=int32)
并用几个例子来测试第4条规则:
print("The 1st element of the first level is:",
rank_2_tensor[0].numpy())
print("The 2nd element of the first level is:",
rank_2_tensor[1].numpy())
# 规则4, 逗号代表进入更深层
print("The 1st element of the second level is:",
rank_2_tensor[0, 0].numpy())
print("The 3rd element of the second level is:",
rank_2_tensor[0, 2].numpy())
Output:
The first element of the first level is: [0 1 2 3 4 5]
The second element of the first level is: [ 6 7 8 9 10 11]
The first element of the second level is: 0
The third element of the second level is: 2
现在,我们已经介绍了索引的基本知识,让我们看看我们可以对张量进行的基本操作。
张量基本运算
你可以轻松地对张量进行基本的数学运算,例如:
-
求最大值或最小值
-
找到Max元素的索引
-
计算Softmax值
让我们看看这些运算。我们将创建两个张量对象并应用这些操作。
a = tf.constant([[2, 4],
[6, 8]], dtype=tf.float32)
b = tf.constant([[1, 3],
[5, 7]], dtype=tf.float32)
我们可以从加法开始。
# 我们可以使用' tf.add() '函数并将张量作为参数传递。
add_tensors = tf.add(a,b)
print(add_tensors)
Output:
tf.Tensor( [[ 3. 7.]
[11. 15.]], shape=(2, 2), dtype=float32)
# 我们可以使用' tf.multiply() '函数并将张量作为参数传递。
multiply_tensors = tf.multiply(a,b)
print(multiply_tensors)
Output:
tf.Tensor( [[ 2. 12.]
[30. 56.]], shape=(2, 2), dtype=float32)
矩阵乘法:
# 我们可以使用' tf.matmul() '函数并将张量作为参数传递。
matmul_tensors = tf.matmul(a,b)
print(matmul_tensors)
Output:
tf.Tensor( [[ 2. 12.]
[30. 56.]], shape=(2, 2), dtype=float32)
注意:Matmul操作是深度学习算法的核心。因此,尽管你不会直接使用matmul,但了解这些操作是至关重要的。
我们上面列出的其他操作示例:
# 使用' tf.reduce_max() '和' tf.reduce_min() '函数可以找到最大值或最小值
print("The Max value of the tensor object b is:",
tf.reduce_max(b).numpy())
# 使用' tf.argmax() '函数可以找到最大元素的索引
print("The index position of the max element of the tensor object b is:",
tf.argmax(b).numpy())
# 使用 tf.nn.softmax'函数计算softmax
print("The softmax computation result of the tensor object b is:",
tf.nn.softmax(b).numpy())
Output:
The Max value of the tensor object b is: 1.0
The index position of the Max of the tensor object b is: [1 1]
The softmax computation result of the tensor object b is: [[0.11920291 0.880797 ] [0.11920291 0.880797 ]]
就像在NumPy数组和pandas数据帧中一样,你也可以重塑张量对象。
这个变形操作非常快,因为底层数据不需要复制。对于重塑操作,我们可以使用tf.reshape函数
# 我们的初始张量
a = tf.constant([[1, 2, 3, 4, 5, 6]])
print('The shape of the initial Tensor object is:', a.shape)
b = tf.reshape(a, [6, 1])
print('The shape of the first reshaped Tensor object is:', b.shape)
c = tf.reshape(a, [3, 2])
print('The shape of the second reshaped Tensor object is:', c.shape)
# 如果我们以shape参数传递-1,那么张量就变平坦化。
print('The shape of the flattened Tensor object is:', tf.reshape(a, [-1]))
Output:
The shape of our initial Tensor object is: (1, 6)
The shape of our initial Tensor object is: (6, 1)
The shape of our initial Tensor object is: (3, 2)
The shape of our flattened Tensor object is: tf.Tensor([1 2 3 4 5 6], shape=(6,), dtype=int32)
如你所见,我们可以很容易地重塑我们的张量对象。但要注意的是,在进行重塑操作时,开发人员必须是合理的。否则,张量可能会混淆,甚至会产生错误。所以,小心点😀.
当我们尝试使用多个张量对象进行组合操作时,较小的张量可以自动伸展以适应较大的张量,就像NumPy数组一样。例如,当你尝试将标量张量与秩2张量相乘时,标量将被拉伸以乘以每个秩2张量元素。参见以下示例:
m = tf.constant([5])
n = tf.constant([[1,2],[3,4]])
print(tf.multiply(m, n))
Output:
tf.Tensor( [[ 5 10]
[15 20]], shape=(2, 2), dtype=int32)
多亏了广播,在对张量进行数学运算时,你不必担心大小匹配。
张量的特殊类型
我们倾向于生成矩形的张量,并将数值存储为元素。但是,TensorFlow还支持不规则或特殊的张量类型,这些类型包括:
-
参差不齐的张量
-
字符串张量
让我们仔细看看每一个都是什么。
参差不齐的张量
参差不齐张量是沿着尺寸轴具有不同数量元素的张量
可以构建不规则张量,如下所示
ragged_list = [[1, 2, 3],[4, 5],[6]]
ragged_tensor = tf.ragged.constant(ragged_list)
print(ragged_tensor)
Output:
<tf.RaggedTensor [[1, 2, 3],
[4, 5],
[6]]>
字符串张量
字符串张量是存储字符串对象的张量。我们可以建立一个字符串张量,就像你创建一个普通的张量对象。但是,我们将字符串对象作为元素而不是数字对象传递,如下所示:
string_tensor = tf.constant(["With this",
"code, I am",
"creating a String Tensor"])
print(string_tensor)
Output:
tf.Tensor([b'With this'
b'code, I am'
b'creating a String Tensor'],
shape=(3,), dtype=string)
最后,稀疏张量是稀疏数据的矩形张量。当数据中有空值时,稀疏张量就是对象。创建稀疏张量有点耗时,应该更主流一些。这里有一个例子:
sparse_tensor = tf.sparse.SparseTensor(indices=[[0, 0], [2, 2], [4, 4]],
values=[25, 50, 100],
dense_shape=[5, 5])
# 我们可以把稀疏张量转换成密集张量
print(tf.sparse.to_dense(sparse_tensor))
Output:
tf.Tensor( [[ 25 0 0 0 0]
[ 0 0 0 0 0]
[ 0 0 50 0 0]
[ 0 0 0 0 0]
[ 0 0 0 0 100]], shape=(5, 5), dtype=int32)
我们已经成功地介绍了TensorFlow的张量对象的基础知识。
这应该会给你很大的信心,因为你现在对TensorFlow框架的基本知识了解得更多了。
查看本教程系列的第1部分:https://link.medium.com/yJp16uPoqab
原文链接:https://towardsdatascience.com/mastering-tensorflow-tensors-in-5-easy-steps-35f21998bb86
欢迎关注磐创AI博客站:
http://panchuang.net/
sklearn机器学习中文官方文档:
http://sklearn123.com/
欢迎关注磐创博客资源汇总站:
http://docs.panchuang.net/
原创文章,作者:磐石,如若转载,请注明出处:https://panchuang.net/2020/10/19/5%e4%b8%aa%e7%ae%80%e5%8d%95%e7%9a%84%e6%ad%a5%e9%aa%a4%e6%8e%8c%e6%8f%a1tensorflow%e7%9a%84tensor/
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK