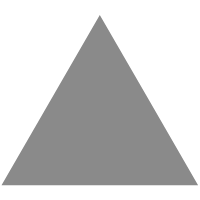
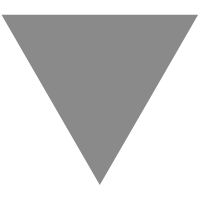
How to run a containerized Java application in the cloud on Microsoft Azure
source link: https://blog.oio.de/2020/05/11/how-to-run-a-containerized-java-application-in-the-cloud-on-microsoft-azure/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to run a containerized Java application in the cloud on Microsoft Azure
My intention for this blog post was to see how fast could I, with basically zero practical cloud experience, deploy a Java application in just that. For this purpose I decided to go for the Azure Cloud Services. Additionally, I made up my mind to also containerize my Java application making use of Docker. This promises me an easier deployment by including all the binaries and libraries needed.
This post is not meant for any Docker/Microsoft Azure expert. But for people like me, who already wrote some Java code and may have peeked a little bit into the Docker topic. Who have never touched “the cloud”, but are interested to see if this is possible without that much background or even if it can be done without spending any money in the first place. To see how you can achieve this in just a few hours of work…
To put it bluntly, it was much easier than I thought it would be. Just to let you know from the start, I did not check what’s the best way to achieve my goal, what the drawbacks are and how to make it quicker. I will just try to give you a summary of what worked well for me. I hope that you can follow along and also deploy your first code in the cloud.
- If you already have a suitable Java project, start with step 2.
- If you already have a dockerized Java application, you can start with step 3.
- If it is crashing for you in step 3, you can give it a try or you may go back to step 1.
- If you already have some Docker container running in Azure, you are in the wrong place here ;-)
Step 1: Let’s have a small and simple Java application
Because I wanted to start quickly with the actual deployment, I decided to go for a new SpringBoot project. However, it should also work with most of the “basic” Java projects you can find out there.
If you have a project yourself, just try it out. If you just don’t feel like making things on your own, here is what I chose to do.
I decided to build a tiny RESTful web service and added the following controller class to the project.
package
com.deployJavaOnAzure.playwithazure;
import
org.springframework.web.bind.annotation.GetMapping;
import
org.springframework.web.bind.annotation.RequestMapping;
import
org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping
(
"/azure/docker/hello"
)
public
class
sayHello {
@GetMapping
public
String hello() {
return
"I'm containerized Java code running in Azure"
;
}
}
To check if this works I also added the following line to the application.properties
file.
server.port=8085
You should now be able to build and run your application. To test this in a browser go to: localhost:8085/azure/docker/hello
Your browser should lie to you now, but hopefully not for long.
If you found this boring, you should have jumped directly to step 2. If this was new for you: congratulations, you run your first SpringBoot RESTful service. If you feel ready, continue to the next level.
Step 2: Put it in a Docker container
Now we want to deploy our application inside a Docker container. “Why one should do such a thing” and how to install Docker on your machine will not be covered here, so I jump right into what I did next. To build a new Docker image we create a Dockerfile
within the project repository.
Along with our application we have to put all needed dependencies and libraries as well. Fortunately, there are plenty of images out there you can build on. All we need is a version of the openjdk image provided by the public repository on DockerHub. I decided to go for the latest alpine release, because it leads to smaller images which will be beneficial especially in the cloud.
Adding our recently created application and opening the port 8085the Dockerfile
will look as follows:
FROM openjdk:8-alpine
ADD build
/libs/dockerizeMe
.jar dockerizeMe.jar
EXPOSE 8085
ENTRYPOINT [
"java"
,
"-jar"
,
"dockerizeMe.jar"
]
To get a better name for the build artifact I just add the following lines to the build.gradle
file.
bootJar {
archiveName =
'dockerizeMe.jar'
}
To build the new image, run the following command in the Powershell (inside your project’s repository):
IN:
docker build -t javaimage .
OUT:
... some lines skipped here ...
Something saying
"SUCCESSFULLY BUILD..."
should be fine.
To check if we didn’t mess things up, we try to create a container instance from the image we just created.
IN:
docker run -p 8085:8085 javaimage
OUT:
. ____ _ __ _ _
/\ / ___'_ __ _ _(_)_ __ __ _
( ( )___ | '_ | '_| | '_ / _` |
\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |___, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.2.6.RELEASE)
If you see this output, you did it right.
Again, we can simply test it: localhost:8085/azure/docker/hello
Congratulations! If you still see the message in your browser, now a bit less exaggerating than before, you have a running Java application, this time deployed inside a Docker container.
If you find it tedious to build a local Docker image as in the intermediate step when we only want to run the container in Azure, you should have a look at this alternative way.
Step 3: Finally, deploy your container to the cloud
Last but not least, we can finally take a look at the cloud provider, namely Microsoft Azure. When you visit the website it–hopefully still–tells you that you can have a free Azure account for at least some time. Please create yourself an account by following the instructions there.
Hopefully, you could successfully create an account for yourself. When you now head to the portal home you are greeted by quite an overloaded but simple to use GUI overview. I will show you how to use it to run your first Java code inside the cloud in no time.
Add all the following components to your account by selecting “Create a resource …”.
First you need to create a “Resource group”, where you’ll put all the components that belong together later on.
You only need to select a name and region. Which values you choose doesn’t matter too much for now. For simplicity just keep the default selection. Then add a “Container Registry”, where we can push our recently created Docker image.
It is also possible to skip this step and work without an own “Container Registry” on Azure. You can also use a local repository or some other repository to which you have access to. For example, you can use your DockerHub repository. But then you need to upload the image each time you want to redeploy it.
Here is my selection, which is sufficient for a small example like ours. Please make sure to enable “Admin user” if you want to use the docker login
option later.

To push our image to the right place we first have to tag it accordingly. In the Powershell do:
docker tag javaimage deployjavaregistry.azurecr.io
/javaimage
To actually push the image to this registry you need to first login with your account credentials. There are several ways to do this, and some of them seem quite troublesome. I decided to use Azure CLI, which you can either run in the Cloud Shell or install on your machine (that’s what I did). Now you simply log in with your account credentials. This generates a token, which is automatically reused in the subsequent steps. In the shell do:
az login
You can now log in to your “Container Registry” without further authentication. All further Docker commands are now also provided with the access token.
az acr login --name deployjavaregistry
If you don’t want to go for the Azure CLI option, you can also log in with Docker. Having enabled “Admin user” in the “Container Registry” you can get the credentials from “deployjavaregistry/Access keys”.
docker login deployjavaregistry.azurecr.io
Hopefully, you overcome the authentication step and we can push our image to the cloud. In case you have problems with authentication, this page may help you.
docker push deployjavaregistry.azurecr.io
/javaimage
This may take some time depending on your connection. Check in “deployjavaregistry/Repositories” if the image safely arrived. If so, we can finally deploy it by choosing the image and select “Run instance”.

Give it a name and select port 8085. After the process finished you should find a new “Container instance” in your “Resource group”. By selecting it, you should be able to get its public IP address.
Let’s see if our code stopped spreading fake news: {IPAddress}:8085/azure/docker/hello
Well done! You have deployed a containerized Java application in Azure!
Now, feel free to play around with your account and image. If you care about the cost, make sure you delete the services you don’t need anymore.
I hope you could follow along and enjoyed the little trip to the cloud. Stay tuned for further posts to come.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK