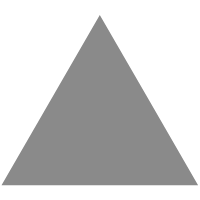
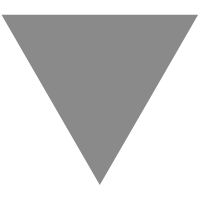
测试驱动技术(TDD)系列之:从excel中读取数据
source link: http://developer.51cto.com/art/202102/644556.htm
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
测试框架中参数化的一般形式
在Junit4和TestNG框架中实现参数化的形式几乎是一致的。
Junit4定义参数化数据,代码如下:
public static Collection prepareData(){ Object [][]object= {{1,2,3},{0,2,2},{0,3,3}}; return Arrays.asList(object); }
TestNG定义参数化数据,代码如下:
@DataProvider public Object[][] dp1() { return new Object[][] { new Object[] { 1, 1,0 }, new Object[] { 2, 1,1 }, new Object[] { 2, 1,2 }, }; }
从excel中读取测试驱动数据的实现思路
在前面的文章中,我详细地讲解了,java中数组的应用,以及如何利用api来操控excel文件。接下来我就把在测试框架中读取excel进行接口测试参数化的核心代码以及实现思路讲解给大家。
1.首先我们看到测试数据的参数化返回值是Object [][] object,那么我们就需要写一个读取excel的方法,该方法的返回值是Object [][],方法可以定义为:Object[][] readExcel(String p_file,int p_sheetindex)
2.excel中读取cell的名称是通过行和列确认的,而且不同类型的cell在读取值时用到的api是不同的,我们写一个读取Cell值得方法,代码实现如下:
public static Object getCellVaule(Sheet p_sheet,int p_rowIndex,int p_cellIndex) { Object value=null; Row row = p_sheet.getRow(p_rowIndex); Cell cell = row.getCell(p_cellIndex); if(cell.getCellType()==CellType.NUMERIC) //判断是数字类型 { value= cell.getNumericCellValue(); } else if(cell.getCellType()==CellType.STRING) //判断是String类型 { value=cell.getStringCellValue(); } else if(cell.getCellType()==CellType.BOOLEAN) // 判断是BOOLEAN类型 { value=cell.getStringCellValue(); } else { value=cell.toString(); // 其他类型统一转化成String类型 } return value; }
3.结合getCellVaule方法,我们编写一个读取完整excel值的方法,返回值为Object[][] ,代码如下:
public static Object[][] readExcel(String p_file,int p_sheetindex){ Workbook workbook=null; Object [][] data=null; try { workbook = new XSSFWorkbook(new FileInputStream(p_file)); Sheet sheet = workbook.getSheetAt(p_sheetindex); // 获取第一个工作表.0代表第一个sheet页 int iRowNum=sheet.getPhysicalNumberOfRows(); //获取工作表中的行数 int iColumnNum= sheet.getRow(0).getPhysicalNumberOfCells(); //获取工作表中的列数 data=new Object[iRowNum][iColumnNum];//通过行列数,创建一个二维数组来保存cell中的数据 for(int i=0;i<iRowNum;i++) { for(int j=0;j<iColumnNum;j++) { data[i][j]= getCellVaule(sheet,i,j); //通过循环以及之前编写好的读取excel单元格值的方法,把cell中的数据保存到二维数组中 } } workbook.close(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } return data; }
4.我们可以把这两个方法作为工具方法,写入到工具类ExcelTool中,方便在测试框架中应用
测试用例中参数化的应用
在测试用例中(以junit4为例),使用该工具方法实现参数化的代码实现如下:
@RunWith(Parameterized.class) public class ParaDemo { private Object input1; private Object input2; @Parameters public static Collection prepareData(){ System.out.println("prepareData"); Object [][] object=ExcelTool.readExcel("D:\\TestData2.xlsx",0); return Arrays.asList(object); } public ParaDemo(Object input1,Object input2,Object expected){ System.out.println("ParaDemo"); this.input1 = input1; this.input2 = input2; } @Test public void testEqual(){ Assert.assertEquals(this.input1,this.input2); } }
excel里保存的测试数据如下所示

运行测试用例,发现参数化成功执行,如下图所示

Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK