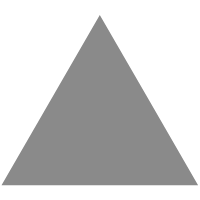
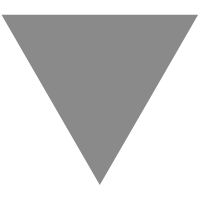
Github GitHub - hrsh7th/nvim-compe: Auto completion plugin for nvim.
source link: https://github.com/hrsh7th/nvim-compe
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
nvim-compe
Auto completion plugin for nvim.
Table Of Contents
Concept
- Lua source & Vim source
- Better matching algorithm
- Support LSP completion features (trigger character, isIncomplete)
Features
- VSCode compatible expansion handling
- rust-analyzer's Magic completion
- vscode-html-languageserver-bin's closing tag completion
- Other complex expansion are supported
- Flexible Custom Source API
- The source can support
documentation
/resolve
/confirm
- The source can support
- Better fuzzy matching algorithm
gu
can be matchedget_user
fmodify
can be matchedfnamemodify
- See matcher.lua for implementation details if you're interested
- Buffer source carefully crafted
- The buffer source will index buffer words by filetype specific regular expression if needed
Usage
The source
option is required but others can be omitted.
Prerequisite
You must set completeopt
to menu,menuone,noselect
which can be easily done as follows.
Using Vimscript
set completeopt=menu,menuone,noselect
or using Lua
vim.o.completeopt = "menu,menuone,noselect"
Available Options
-
compe.enabled (bool)
: Whether or not nvim-compe is enabled. default:true
. -
compe.autocomplete (bool)
: Whether or not nvim-compe opens the popup menu automatically. default:true
. -
compe.debug (bool)
: Whether or not nvim-compe should display debug info. default:false
-
compe.min_length (number)
: Minimal characters length to trigger completion. default:1
-
compe.preselect ("enable" | "disable" | "always")
Controls nvim-compe preselect behaviour. default:enable
enable
: Preselect completion item only if the source told nvim-compe to do so. Eg. completion fromgopls
disable
: Never preselect completion item regardless of sourcealways
: Always preselect completion item regardless of source
-
compe.throttle_time (number)
: Throttle nvim-compe completion menu. default:80
-
compe.source_timeout (number)
: Timeout for nvim-compe to get completion items. default:200
-
compe.incomplete_delay (number)
: Delay for LSP'sisIncomplete
. default:400
-
compe.allow_prefix_unmatch
: TODO??? -
compe.source.path (bool)
: Path completion. default:false
-
compe.source.buffer (bool)
: Buffer completion. default:false
-
compe.source.vsnip (bool)
: Vsnip completion, make sure you havevim-vsnip
installed. default:false
-
compe.source.nvim_lsp (bool)
: Nvim's builtin LSP completion. default:false
-
compe.source.nvim_lua (bool)
: Nvim's Lua "stdlib" completion. default:false
-
compe.source.spell (bool)
: Dictionary completion if you setspell
. default:false
-
compe.source.snippets_nvim (bool)
: snippets.nvim completion. default:false
-
compe.source.your_awesome_source (table | dict)
: Override source configuration using a customtable
(lua) ordictionary
(vimscript).
Example Configuration
Both Vimscript and Lua example are using the default value except the source field which enables all sources.
Vimscript Config
let g:compe = {} let g:compe.enabled = v:true let g:compe.autocomplete = v:true let g:compe.debug = v:false let g:compe.min_length = 1 let g:compe.preselect = 'enable' let g:compe.throttle_time = 80 let g:compe.source_timeout = 200 let g:compe.incomplete_delay = 400 let g:compe.allow_prefix_unmatch = v:false let g:compe.source = {} let g:compe.source.path = v:true let g:compe.source.buffer = v:true let g:compe.source.vsnip = v:true let g:compe.source.nvim_lsp = v:true let g:compe.source.nvim_lua = v:true let g:compe.source.spell = v:true let g:compe.source.snippets_nvim= v:true let g:compe.source.your_awesome_source = {}
require'compe'.setup { enabled = true; autocomplete = true; debug = false; min_length = 1; preselect = 'enable'; throttle_time = 80; source_timeout = 200; incomplete_delay = 400; allow_prefix_unmatch = false; source = { path = true; buffer = true; vsnip = true; nvim_lsp = true; nvim_lua = true; spell = true; snippets_nvim = true; your_awesome_source = {}; }; }
Mappings
If you don't use any autopair plugin.
inoremap <silent><expr> <C-Space> compe#complete() inoremap <silent><expr> <CR> compe#confirm('<CR>') inoremap <silent><expr> <C-e> compe#close('<C-e>')
If you use cohama/lexima.vim
inoremap <silent><expr> <C-Space> compe#complete() inoremap <silent><expr> <CR> compe#confirm(lexima#expand('<LT>CR>', 'i')) inoremap <silent><expr> <C-e> compe#close('<C-e>')
If you use Raimondi/delimitMate
inoremap <silent><expr> <C-Space> compe#complete() inoremap <silent><expr> <CR> compe#confirm({ 'keys': "\<Plug>delimitMateCR", 'mode': '' }) inoremap <silent><expr> <C-e> compe#close('<C-e>')
Source Configuration
The sources can be configured by let g:compe.source['source_name'] = { ...configuration... }
in Vimscript or passing the configuration inside sources['source_name']
field in Lua setup function.
- priority
- Specify source priority.
- filetypes
- Specify source filetypes.
- ignored_filetypes
- Specify filetypes that should not use this source.
- sort
- Specify source is sortable or not.
- dup
- Specify source candidates can have the same word another item.
- menu
- Specify item's menu (see
:help complete-items
)
- Specify item's menu (see
Built-in sources
Common
- buffer
- spell
Neovim-specific
- nvim_lsp
- nvim_lua
External-plugin
- vim_lsp
- vsnip
- ultisnips
- snippets.nvim
Development
Example source
You can see example on vim-dadbod-completion
The source
The source is defined as dict that has get_metadata
/determine
/complete
and documentation(optional)
.
- get_metadata
- This function should return the default source configuration. see
Source configuration
section.
- This function should return the default source configuration. see
- determine
- This function should return dict as
{ keyword_pattern_offset = 1-origin number; trigger_character_offset = 1-origin number}
. - If this function returns empty, nvim-compe will do nothing.
- This function should return dict as
- complete
- This function should callback the completed items as
args.callback({ items = items })
. - If you want to stop the completion process, you should call
args.abort()
.
- This function should callback the completed items as
- documentation
- You can provide documentation for selected items.
Public API
The compe is under development so I will apply breaking change sometimes.
The below APIs are mark as public.
Vim script
" Setup user configuration. call compe#setup({ ... }) " Register and unregister source. let l:id = compe#register_source('name', s:source) call compe#unregister_source(l:id) " Invoke completion. call compe#complete() " Confirm selected item. call compe#confirm('<C-y>') " optional fallback key. " Close completion menu. call compe#close('<C-e>') " optional fallback key. " Source helpers. call compe#helper#*()
-- Setup user configuration. require'compe'.setup({ ... }) -- Register and unregister source. local id = require'compe'.register_source(name, source) require'compe'.unregister_source(id) -- Source helpers. require'compe'.helper.*
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK