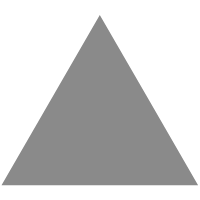
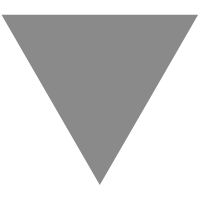
New features for unit testing your Entity Framework Core 5 code
source link: https://www.thereformedprogrammer.net/new-features-for-unit-testing-your-entity-framework-core-5-code/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
New features for unit testing your Entity Framework Core 5 code
This article is about unit testing applications that use Entity Framework Core (EF Core), with the focus on the new approaches you can use in EF Core 5. I start out with an overview of the different ways of unit testing in EF Core and then highlight some improvements brought in EF Core 5, plus some improvements I added to the EfCore.TestSupport version 5 library that is designed to help unit testing of EF Core 5.
NOTE: I am using xUnit in my unit tests. xUnit is well supported in NET and widely used (EF Core uses xUnit for its testing).
TL;DR – summary
- There are three main ways to unit test your EF Core code:
- Use the same type of database as your production system. Best choice
- Use in-memory SQLite databases. Fastest choice, but has limitations
- Use some form of Repository pattern and mock your repository. Good, but more work.
- If you are using a database you need create a unique, empty test database with the correct schema. I describe three ways to do this.
- There is an EF Core feature called Identity Resolution that can cause code with errors to still pass. I show how to get around this with a new feature in EF Core 5.
- When using the SQLite in-memory database in a unit test you should dispose the database at the end to ensure the memory is released.
- There are two new ways in EF Core 5 to inspect the SQL created by your EF Core code.
- Version 5 of the EfCore.TestSupport library supports EF Core 5, but it has breaking changes. If you are already using EfCore.TestSupport read this document before upgrading.
NOTE: In this article I use the term entity class (or entity instance) to refer to a class that is mapped to the database by EF Core.
Setting the scene – why, and how, I unit test my EF Core code
I have used unit testing since I came back to being a developer (I had a long stint as a tech manager) and I consider unit testing one of the most useful tools to produce correct code. And when it comes to database code, which I write a lot of, I started with a repository pattern which is easy to unit test, but I soon moved away from the repository patten to using Query Objects. At that point had to work out how to unit test my code that uses the database. This was critical to me as I wanted my tests to cover as much of my code as possible.
In EF6.x I used a library called Effort which mocks the database, but when EF Core came out, I had to find another way. I tried EF Core’s In-Memory database provider, but the EF Core’s SQLite database provider using an in-memory database was MUCH better. The SQLite in-memory database (I cover this later) very easy to use for unit tests, but has some limitations so sometimes I have to use a real database.
The reason why I unit test my EF Core code is to make sure they work as I expected. Typical things that I am trying to catch.
- Bad LINQ code: EF Core throws an exception if can’t translate my LINQ query into database code. That will make the unit test fail.
- Database write didn’t work. Sometimes my EF Core create, update or delete didn’t work the way I expected. Maybe I left out an Include or forgot to call SaveChanges. By testing the database
While some people don’t like using unit tests on a database my approach has caught many, many errors which would be been hard to catch in the application. Also, a unit test gives me immediate feedback when I’m writing code and continues to check that code as I extend and refactor that code.
The three ways to unit test your EF Core code
Before I start on the new features here is a quick look at the three ways you can unit test your EF Core code. The figure (which comes from chapter 17 on my book “Entity Framework Core in Action, 2nd edition”) compares three ways to unit test your EF Core code, with the pros and cons of each.
As the figure says, using the same type of database in your unit test as what your application uses is the safest approach – the unit test database accesses will respond just the same as your production system. In this case, why would I also list using an SQLite in-memory database in unit tests? While there are some limitations/differences from other databases, it does have many positives:
- The database schema is always up to date.
- The database is empty, which is a good starting point for a unit test.
- Running your unit tests in parallel works because each database is held locally in each test.
- Your unit tests will run successfully in the Test part of a DevOps pipeline without any other settings.
- Your unit tests are faster.
NOTE: Item 3, “running your unit tests in parallel”, is an xUnit feature which makes running unit tests much quicker. It does mean you need separate databases for each unit test class if you are using a non in-memory database. The library EfCore.TestSupport has features to obtain unique database names for SQL Server databases.
The last option, mocking the database, really relies on you using some form pf repository pattern. I use this for really complex business logic where I build a specific repository pattern for the business logic, which allows me to intercept and mock the database access – see this article for this approach.
The new features in EF Core 5 that help with unit testing
I am going to cover four features that have changes in the EfCore.TestSupport library, either because of new features in EF Core 5, or improvements that have been added to the library.
- Creating a unique, empty test database with the correct schema
- How to make sure your EF Core accesses match the real-world usage
- Improved SQLite in-memory options to dispose the database
- How to check the SQL created by your EF Core code
Creating a unique, empty test database with the correct schema
To use a database in a xUnit unit test it needs to be:
- Unique to the test class: that’s needed to allow for parallel running of your unit tests
- Its schema must match the current Model of your application’s DbContext: if the database schema is different to what EF Core things it is, then your unit tests aren’t working on the correct database.
- The database’s data should be a known state, otherwise your unit tests won’t know what to expect when reading the database. An empty database is the best choice.
Here are three ways to make sure database fulfils these three requirements.
- Use an SQLite in-memory which is created every time.
- Use a unique database name, plus calling EnsureDeleted, and then EnsureCreated.
- Use unique database name, plus call the EnsureClean method (only works on SQL Server).
1. Use an SQLite in-memory which is created every time.
The SQLite database has a in-memory mode, which is applied by setting the connection string to “Filename=:memory:”. The database is then hidden in the connection string, which makes it unique to its unit test and its database hasn’t been created yet. This is quick and easy, but you production database uses another type of database then it might not work for you.
The EF Core documentation on unit testing shows one way to set up a in-memory SQLite database, but I use the EfCore.TestSupport library’s static method called SqliteInMemory.CreateOptions<TContext> that will setup the options for creating an SQLite in-memory database, as shown in the code below.
[Fact]
public
void
TestSqliteInMemoryOk()
{
//SETUP
var
options = SqliteInMemory.CreateOptions<BookContext>();
using
var
context =
new
BookContext(options);
context.Database.EnsureCreated();
//Rest of unit test is left out
}
The database isn’t created at the start, so you need to call EF Core’s EnsureCreated method at the start. This means you get a database that matches the current Model of your application’s DbContext.
2. Unique database name, plus Calling EnsureDeleted, then EnsureCreated
If you are use a normal (non in-memory) database, then you need to make sure the database has a unique name for each test class (xUnit runs test classes in parallel, but methods in a test class are run serially). To get a unique database name the EfCore.TestSupport has methods that take a base SQL Server connection string from an appsetting.json file and adds the class name to the end of the current name (see code after next paragraph, and this docs).
That solves the unique database name, and we solve the “matching schema” and “empty database” by calling the EnsureDeleted method, then the EnsureCreated method. These two methods will delete the existing database and create a new database whose schema will match the EF Core’s current Model of the database. The EnsureDeleted / EnsureCreated approach works for all databases but is shown with SQL Server here.
[Fact]
public
void
TestEnsureDeletedEnsureCreatedOk()
{
//SETUP
var
options =
this
.CreateUniqueClassOptions<BookContext>();
using
var
context =
new
BookContext(options);
context.Database.EnsureDeleted();
context.Database.EnsureCreated();
//Rest of unit test is left out
}
The EnsureDeleted / EnsureCreated approach used to be very slow (~10 seconds) for a SQL Server database, but since the new SqlClient came out in NET 5 this is much quicker (~ 1.5 seconds), which makes a big difference to how long a unit test would take to run when using this EnsureDeleted + EnsureCreated version.
3. Unique database name, plus call the EnsureClean method (only works on SQL Server).
While asking some questions on the EFCore GitHub Arthur Vickers described a method that could wipe the schema of an SQL Server database. This clever method removed the current schema of the database by deleting all the SQL indexes, constraints, tables, sequences, UDFs and so on in the database. It then, by default, calls EnsuredCreated method to return a database with the correct schema and empty of data.
The EnsureClean method is deep inside EF Core’s unit tests, but I extracted that code and build the other parts needed to make it useful and it is available in version 5 of the EfCore.TestSupport library. The following listing shows how you use this method in your unit test.
[Fact]
public void TestSqlDatabaseEnsureCleanOk()
{
//SETUP
var options = this.CreateUniqueClassOptions<BookContext>();
using var context = new BookContext(options);
context.Database.EnsureClean();
//Rest of unit test is left out
}
EnsureClean approach is a faster, maybe twice as fast as the EnsureDeleted + EnsureCreated version, which could make a big difference to how long your unit tests take to run. It also better in situations where your database server won’t allow you to delete or create new databases but does allow you to read/write a database, for instance if your test databases were on SQL Server where you don’t have admin privileges.
How to make sure your EF Core accesses match the real-world usage
Each unit test is a single method that has to a) setup the database ready for testing, b) runs the code you are testing, and the final part, c) checks that the results of the code you are testing are correct. And the middle part, run the code, must reproduce the situation in which the code you are testing is normally used. But because all three parts are all in one method it can be difficult to create the same state that the test code is normally used in.
The issue of “reproducing the same state the test code is normally used in” is common to unit testing, but when testing EF Core code this is made more complicated by the EF Core feature called Identity Resolution. Identity Resolution is critical in your normal code as it makes sure you only have one entity instance of a class type that has a specific primary key (see this example). The problem is that Identity Resolution can make your unit test pass even when there is a bug in your code.
Here is a unit test that passes because of Identity Resolution. The test of the Price at the end of the unit test should fail, because SaveChanges wasn’t called (see line 15). The reason it passed is because the entity instance in the variable called verifyBook was read from the database, but because the tracked entity instances inside the DbContext was found, and that was returned instead of reading from the database.
[Fact]
public
void
ExampleIdentityResolutionBad()
{
//SETUP
var
options = SqliteInMemory
.CreateOptions<EfCoreContext>();
using
var
context =
new
EfCoreContext(options);
context.Database.EnsureCreated();
context.SeedDatabaseFourBooks();
//ATTEMPT
var
book = context.Books.First();
book.Price = 123;
// Should call context.SaveChanges()
//VERIFY
var
verifyBook = context.Books.First();
//!!! THIS IS WRONG !!! THIS IS WRONG
verifyBook.Price.ShouldEqual(123);
}
In the past we fixed this with multiple instances of the DbContext, as shown in the following code
public
void
UsingThreeInstancesOfTheDbcontext()
{
//SETUP
var
options = SqliteInMemory
.CreateOptions<EfCoreContext>();
options.StopNextDispose();
using
(
var
context =
new
EfCoreContext(options))
{
//SETUP instance
}
options.StopNextDispose();
using
(
var
context =
new
EfCoreContext(options))
{
//ATTEMPT instance
}
using
(
var
context =
new
EfCoreContext(options))
{
//VERIFY instance
}
}
But there is a better way to do this with EF Core 5’s ChangeTracker.Clear method. This method quickly removes all entity instances the DbContext is currently tracking. This means you can use one instance of the DbContext, but each stage, SETUP, ATTEMPT and VERIFY, are all isolated which stops Identity Resolution from giving you data from another satge. In the code below there are two potential errors that would slip through if you didn’t add calls to the ChangeTracker.Clear method (or used multiple DbContexts).
- Line 15: If the Include was left out the unit test would still pass (because the Reviews collection was set up in the SETUP stage).
- Line 19: If the SaveChanges was left out the unit test would still pass (because the VERIFY read of the database would have bee given the book entity from the ATTEMPT stage)
public
void
UsingChangeTrackerClear()
{
//SETUP
var
options = SqliteInMemory
.CreateOptions<EfCoreContext>();
using
var
context =
new
EfCoreContext(options);
context.Database.EnsureCreated();
var
setupBooks = context.SeedDatabaseFourBooks();
context.ChangeTracker.Clear();
//ATTEMPT
var
book = context.Books
.Include(b => b.Reviews)
.Single(b => b.BookId = setupBooks.Last().BookId);
book.Reviews.Add(
new
Review { NumStars = 5 });
context.SaveChanges();
//VERIFY
context.ChangeTracker.Clear();
context.Books.Include(b => b.Reviews)
.Single(b => b.BookId = setupBooks.Last().BookId)
.Reviews.Count.ShouldEqual(3);
}
This is much better than the three separate DbContext instances because
- You don’t have to create the three DbContext’s scopes (saves typing. Shorter unit test)
- You can use using var context = …, so no indents (nicer to write. Easier to read)
- You can still refer to previous parts, say to get its primary key (see use of setupBooks on line 16 and 25)
- It works better with the improved SQLite in-memory disposable options (see next section)
Improved SQLite in-memory options to dispose the database
You have already seen the SqliteInMemory.CreateOptions<TContext> method earlier but in version 5 of the EfCore.TestSupport library I have updated it to dispose the SQLite connection when the DbContext is disposed. The SQLite connection holds the in-memory database so disposing it makes sure that the memory used to hold the database is released.
NOTE: In previous versions of the EfCore.TestSupport library I didn’t do that, and I haven’t had any memory problems. But the EF Core docs say you should dispose the connection, so I updated the SqliteInMemory options methods to implement the IDisposable interface.
It turns out the disposing of the DbContext instance will dispose the options instance, which in turn disposes the SQLite connection. See the comments at the end of the code.
[Fact]
public
void
TestSqliteInMemoryOk()
{
//SETUP
var
options = SqliteInMemory.CreateOptions<BookContext>();
using
var
context =
new
BookContext(options);
//Rest of unit test is left out
}
// context is disposed at end of the using var scope,
// which disposes the options that was used to create it,
// which in turn disposes the SQLite connection
NOTE: If you use multiple instances of the DbContext based on the same options instance, then you need to use one of these approaches to delay the dispose of the options until the end of the unit test.
How to check the SQL created by your EF Core code
If I’m interested in the performance of some part of the code I am working on, it often easier to look at the SQL commands in a unit test than in the actual application. EF Core 5 provides two ways to do this:
- The AsQueryString method to use on database queries
- Capturing EF Core’s logging output using the LogTo method
1. The AsQueryString method to use on database queries
The AsQueryString method will turn an IQueryable variable built using EF Core’s DbContext into a string containing the database commands for that query. Typically, I output this string the xUnit’s window so I can check it (see line 24). In the code below also contains a test so you can see the created SQL code – I don’t normally do that, but I put it in so you could see the type of output you get.
public
class
TestToQueryString
{
private
readonly
ITestOutputHelper _output;
public
TestToQueryString(ITestOutputHelper output)
{
_output = output;
}
[Fact]
public
void
TestToQueryStringOnLinqQuery()
{
//SETUP
var
options = SqliteInMemory.CreateOptions<BookDb
using
var
context =
new
BookDbContext(options);
context.Database.EnsureCreated();
context.SeedDatabaseFourBooks();
//ATTEMPT
var
query = context.Books.Select(x => x.BookId);
var
bookIds = query.ToArray();
//VERIFY
_output.WriteLine(query.ToQueryString());
query.ToQueryString().ShouldEqual(
"SELECT \"b\".\"BookId\"\r\n"
+
"FROM \"Books\" AS \"b\"\r\n"
+
"WHERE NOT (\"b\".\"SoftDeleted\")"
);
bookIds.ShouldEqual(
new
[]{1,2,3,4});
}
}
2. Capturing EF Core’s logging output using the LogTo method
EF Core 5 makes it much easier to capture the logging that EF Core outputs (before you needed to create a ILoggerProvider class and register that with EF Core). But now you can add the LogTo method to your options and it will return a string output for every log. The code below shows how to do this, with the logs output to the xUnit’s window.
[Fact]
public
void
TestLogToDemoToConsole()
{
//SETUP
var
connectionString =
this
.GetUniqueDatabaseConnectionString();
var
builder =
new
DbContextOptionsBuilder<BookDbContext>()
.UseSqlServer(connectionString)
.EnableSensitiveDataLogging()
.LogTo(_output.WriteLine);
// Rest of unit test is left out
The LogTo has lots of different ways to filter and format the output (see the EF Core docs here). I created versions of the EfCore.TestSupport’s SQLite in-memory and SQL Server option builders to use LogTo, and I decided to use a class, called LogToOptions, to manage all the filters/formats for LogTo (the LogTo requires calls to different methods). This allowed me to define better defaults (defaults to: a) Information, not Debug, log level, b) does not include datetime in output) for logging output and make it easier for the filters/formats to be changed.
I also added a feature that I use a lot, that is the ability to turn on or off the log output. The code below shows the SQLite in-memory option builder with the LogTo output, plus using the ShowLog feature. This only starts output logging once the database has been created and seeded – see highlighted line 17 .
[Fact]
public
void
TestEfCoreLoggingCheckSqlOutputShowLog()
{
//SETUP
var
logToOptions =
new
LogToOptions
{
ShowLog =
false
};
var
options = SqliteInMemory
.CreateOptionsWithLogTo<BookContext>(
_output.WriteLine);
using
var
context =
new
BookContext(options);
context.Database.EnsureCreated();
context.SeedDatabaseFourBooks();
//ATTEMPT
logToOptions.ShowLog =
true
;
var
book = context.Books.Single(x => x.Reviews.Count() > 1);
//Rest of unit test left out
}
Information to existing user of the EfCore.TestSupport library
It’s no longer possible to detect the EF Core version via the netstandard so now it is done via the first number in the library’s version. For instance EfCore.TestSupport, version 5.?.? works with EF Core 5.?.?.* At the same time the library was getting hard to keep up to date, especially with EfSchemaCompare in it, so I took the opportunity to clean up the library.
BUT that clean up includes BREAKING CHANGES, mainly around SQLite in-memory option builder. If you use SqliteInMemory.CreateOptions you MUST read this document to decided whether you want to upgrade or not.
NOTE: You may not be aware, but your NuGet packages in your test projects override the same NuGet packages installed EfCore.TestSupport library. So, as long as you add the newest versions of the EF Core NuGet libraries, then the EfCore.TestSupport library will use those. The only part that won’t run is the EfSchemaCompare, but has now got its own library so you can use that directly.
Conclusion
I just read a great article on the stackoverfow blog which said
“Which of these bugs would be easier for you to find, understand, repro, and fix: a bug in the code you know you wrote earlier today or a bug in the code someone on your team probably wrote last year? It’s not even close! You will never, ever again debug or understand this code as well as you do right now, with your original intent fresh in your brain, your understanding of the problem and its solution space rich and fresh.“
I totally agree and that’s why I love unit tests – I get feedback as I am developing (I also like that unit tests will tell me if I broke some old code too). So, I couldn’t work without unit tests, but at the same time I know that unit tests can take a lot of development time. How do I solve that dilemma?
My answer to the extra development time isn’t to write less unit tests, but to build a library and develop patterns that makes me really quick at unit testing. I also try to make my unit tests fast to run – that’s why I worry about how long it takes to set up the database, and why I like xUnit’s parallel running of unit tests.
The changes to the EfCore.TestSupport library and my unit test patterns due to EF Core 5 are fairly small, but each one reduces the number of lines I have to write for each unit test or makes the unit test easier to read. I think the ChangeTracker.Clear method is the best improvement because it does both – it’s easier to write and easier to read my unit tests.
Happy coding.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK