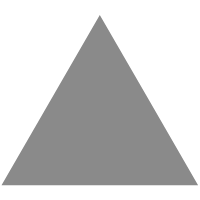
12
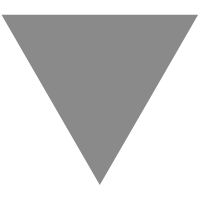
使用 Java 客户端添加 ElasticSearch 文档
source link: https://mp.weixin.qq.com/s?__biz=MzI1NDY0MTkzNQ%3D%3D&%3Bmid=2247492127&%3Bidx=1&%3Bsn=8b71c47931a7befa911e19261a105165
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
松哥原创的 Spring Boot 视频教程已经杀青,感兴趣的小伙伴戳这里--> Spring Boot+Vue+微人事视频教程
今天我们来继续看 ElasticSearch 的 Java 客户端操作~我们来看下如何利用 Java 客户端添加 Es 文档。
以下是视频笔记:
注意,笔记只是视频内容的一个简要记录,因此笔记内容比较简单,完整的内容可以查看视频。
29.1 添加文档
public class DocTest01 {
public static void main(String[] args) throws IOException {
RestHighLevelClient client = new RestHighLevelClient(RestClient.builder(
new HttpHost("localhost", 9200, "http"),
new HttpHost("localhost", 9201, "http"),
new HttpHost("localhost", 9202, "http")
));
//构建一个 IndexRequest 请求,参数就是索引名称
IndexRequest request = new IndexRequest("book");
//给请求配置一个 id,这个就是文档 id。如果指定了 id,相当于 put book/_doc/id ,也可以不指定 id,相当于 post book/_doc
// request.id("1");
//构建索引文本,有三种方式:JSON 字符串、Map 对象、XContentBuilder
request.source("{\"name\": \"三国演义\",\"author\": \"罗贯中\"}", XContentType.JSON);
//执行请求,有同步和异步两种方式
//同步
IndexResponse indexResponse = client.index(request, RequestOptions.DEFAULT);
//获取文档id
String id = indexResponse.getId();
System.out.println("id = " + id);
//获取索引名称
String index = indexResponse.getIndex();
System.out.println("index = " + index);
//判断文档是否添加成功
if (indexResponse.getResult() == DocWriteResponse.Result.CREATED) {
System.out.println("文档添加成功");
}
//判断文档是否更新成功(如果 id 已经存在)
if (indexResponse.getResult() == DocWriteResponse.Result.UPDATED) {
System.out.println("文档更新成功");
}
ReplicationResponse.ShardInfo shardInfo = indexResponse.getShardInfo();
//判断分片操作是否都成功
if (shardInfo.getTotal() != shardInfo.getSuccessful()) {
System.out.println("有存在问题的分片");
}
//有存在失败的分片
if (shardInfo.getFailed() > 0) {
//打印错误信息
for (ReplicationResponse.ShardInfo.Failure failure : shardInfo.getFailures()) {
System.out.println("failure.reason() = " + failure.reason());
}
}
//异步
// client.indexAsync(request, RequestOptions.DEFAULT, new ActionListener<IndexResponse>() {
// @Override
// public void onResponse(IndexResponse indexResponse) {
//
// }
//
// @Override
// public void onFailure(Exception e) {
//
// }
// });
client.close();
}
}
演示分片存在问题的情况。由于我只有三个节点,但是在创建索引时,设置需要三个副本,此时的节点就不够用:
PUT book
{
"settings": {
"number_of_replicas": 3,
"number_of_shards": 3
}
}
创建完成后,再次执行上面的添加代码,此时就会打印出 有存在问题的分片
。
构建索引信息,有三种方式:
//构建索引文本,有三种方式:JSON 字符串、Map 对象、XContentBuilder
//request.source("{\"name\": \"三国演义\",\"author\": \"罗贯中\"}", XContentType.JSON);
//Map<String, String> map = new HashMap<>();
//map.put("name", "水浒传");
//map.put("author", "施耐庵");
//request.source(map).id("99");
XContentBuilder jsonBuilder = XContentFactory.jsonBuilder();
jsonBuilder.startObject();
jsonBuilder.field("name", "西游记");
jsonBuilder.field("author", "吴承恩");
jsonBuilder.endObject();
request.source(jsonBuilder);
默认情况下,如果 request 中包含有 id 属性,则相当于 PUT book/_doc/1
这样的请求,如果 request 中不包含 id 属性,则相当于 POST book/_doc
,此时 id 会自动生成。对于前者,如果 id 已经存在,则会执行一个更新操作。也就是 es 的具体操作,会自动调整。
当然,也可以直接指定操作。例如,指定为添加文档的操作:
//构建一个 IndexRequest 请求,参数就是索引名称
IndexRequest request = new IndexRequest("book");
XContentBuilder jsonBuilder = XContentFactory.jsonBuilder();
jsonBuilder.startObject();
jsonBuilder.field("name", "西游记");
jsonBuilder.field("author", "吴承恩");
jsonBuilder.endObject();
request.source(jsonBuilder).id("99");
//这是一个添加操作,不要自动调整为更新操作
request.opType(DocWriteRequest.OpType.CREATE);
//执行请求,有同步和异步两种方式
//同步
IndexResponse indexResponse = client.index(request, RequestOptions.DEFAULT);
ElasticSearch 基础知识:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK