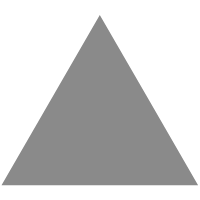
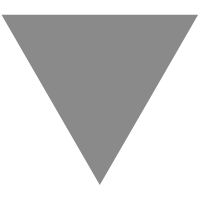
Java OOP: Object reference of subclass
source link: https://www.codesd.com/item/java-oop-object-reference-of-subclass.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Java OOP: Object reference of subclass
I have an ArrayClass
and mergeSortArray
extends it. And mergeSortArray
contains a mergeSort()
method. However, since I used super
to call a constructor from the superclass, I do not know how to refer to the mergeSortArray (the subclass object / array) and pass it as a parameter in the mergeSort
method. In fact, is this even feasible ? I know I can do this in a NON- OOP way. However, I am keen to know how to do this in an OOP way.
Please correct me if I have said incorrect, as I am new to Java and I want to learn more about it.
// ArrayClass Object
import java.util.*;
import java.io.*;
import java.math.*;
public class ArrayClass{
public int[] input_array;
public int nElems;
public ArrayClass(int max){
input_array = new int [max];
nElems = 0;
}
public void insert(int value){
input_array[nElems++] = value;
}
public void display(){
for(int j = 0; j < nElems; j++){
System.out.print(input_array[j] + " ");
}
System.out.println("");
}
}
import java.io.*;
import java.util.*;
import java.math.*;
class mergeSortArray extends ArrayClass{
public mergeSortArray(int max){
super(max);
}
public void methodOne(){
int[] output_array = new int[super.nElems];
mergeSort( // ************* // ,output_array,0, super.nElems -1);
}
................
}
I am not sure what I should put to replace ******
such that I can pass mergeSortArray
as a parameter into the mergeSort
method.
There isn't a mergeSortArray
. You inherit input_array
like (and no need for super.nElems
you inherit that too),
mergeSort( input_array, output_array, 0, nElems - 1);
Your sub-class will inherit everything that is protected
or greater visibility (not private
), however your ArrayClass
gives you both public fields
public int[] input_array;
public int nElems;
They should probably be protected
and have accessor methods (getters).
protected int[] input_array;
protected int nElems;
public int size() {
return nElems;
}
public int[] getInputArray() {
return input_array;
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK