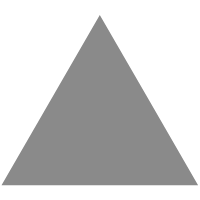
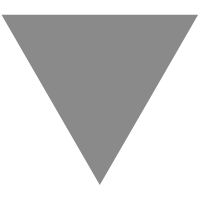
Setup JWT as OAuth Bearer Token in a ASP.NET Core Application
source link: https://www.roundthecode.com/dotnet/setup-jwt-as-oauth-bearer-token-in-asp-net-core-application
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Setup JWT as OAuth Bearer Token in a ASP.NET Core Application: OAuth Security - Part 2
23rd December 2020We are going to set up OAuth security, by creating a JSON Web Token as a Bearer token in an ASP.NET Core application.
In part 1, we had a look at how we can add Basic Authentication to an ASP.NET Core application.
We are going to use Basic authentication when generating a Bearer token. This is so we can offer some additional protection when generating a token.
In addition, we are going to decrypt the Bearer token to see what information is included.
As well, we are going to set up Bearer authentication and test it to check that it's working.
How to Generate a Bearer Token
To generate an OAuth token, we are going to create a new OAuth controller. Inside that controller, we are going to create a Token action.
Inside the Token action, we are going to write the functionality for creating the token.
When creating a token, we have to include these parameters:
- Subject - The identity of the user being authenticated
- Expires - The time (in UTC) when the token will expire
- Issuer - The name of the issuer of the ticket
- Audience - The name of the audience of which the ticket is intended for
- Signing Credentials - A security key password, which needs to be at least 15 characters
- Issued at - The time (in UTC) when the token was issued
Once the token has been generated, we output it as part of a JSON response.
In addition, we return the token type and the number of seconds when the token is due to expire.
We've also wrapped this Token action with a BasicAuthorization attribute. This is so the request has to pass in a username and password before it can be authenticated and generate a token.
// OAuthController.cs
[Route(
"oauth"
)]
public
class
OAuthController : Controller
{
[Route(
"token"
), BasicAuthorization]
public
IActionResult Token()
{
var
tokenHandler =
new
JwtSecurityTokenHandler();
var
authenticatedUser =
new
AuthenticatedUser(JwtBearerDefaults.AuthenticationScheme,
true
,
"roundthecode"
);
var
accessToken = tokenHandler.WriteToken(tokenHandler.CreateToken(
new
SecurityTokenDescriptor
{
Subject =
new
ClaimsIdentity(authenticatedUser),
Expires = DateTime.UtcNow.AddMinutes(1),
Issuer =
"me"
,
Audience =
"you"
,
SigningCredentials =
new
SigningCredentials(
new
SymmetricSecurityKey(Encoding.UTF8.GetBytes(
"roundthecode9999"
)), SecurityAlgorithms.HmacSha256Signature),
IssuedAt = DateTime.UtcNow
}));
return
Ok(
new
{ access_token = accessToken, token_type =
"bearer"
, expires_in = 60 });
}
}
What does the OAuth Bearer Token Contain?
At this point, it's a good idea to identify what the OAuth Bearer token contains.
When generating a Bearer token, it will look something like this:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1bmlxdWVfbmFtZSI6InJvdW5kdGhlY29kZSIsIm5iZiI6MTYwMzMwMzAyNCwiZXhwIjoxNjAzMzAzMDg0LCJpYXQiOjE2MDMzMDMwMjQsImlzcyI6Im1lIiwiYXVkIjoieW91In0.C3ve18YKc74F0NZzuSyS5F4JctEn2j3AsBoQpWItYms
So how do we decrypt this token? Well, we can go to the JWT website and paste the token into it's debugger.
Decrypt a Bearer Token on JWT.io
From the JWT debugger, we can see that the decoded token contains the following information:
{
"unique_name"
:
"roundthecode"
,
"nbf"
: 1603303024,
"exp"
: 1603303084,
"iat"
: 1603303024,
"iss"
:
"me"
,
"aud"
:
"you"
}
To give some information on what each means:
- unique_name - The name of the user that should be authenticated with this token
- nbf - Stands for "Not valid before". A timestamp to indicate the time when the token is valid from.
- exp - Stands for "Expires". A timestamp to indicate when the token expires.
- iat - Stands for "Issued at". A timestamp to indicate when the token was issued at.
- iss - Stands for "Issuer". Basically telling us who created the token.
- aud - Stands for "Audience". Who or what the token is intended for.
Set up OAuth Bearer Authentication in ASP.NET Core
Now that we have written the code to generate a token and know what is contained in a token, we can go ahead and set up OAuth Bearer authentication in ASP.NET Core.
In Startup, we need to call the AddJWTBearer method which is part of the AuthenticationBuilder. In there, we need to set up some validation parameters.
These will be checked against any Bearer token passed into the request. It will check against the issuer, the audience and the signing credentials.
In addition, we are going to be using the default Authorize attribute as using Bearer authentication. As a result, we need to set up Bearer authentication as the default authentication scheme.
As well, we need to set up a default authorisation policy, which we will set up as JWT Bearer.
// Startup.cs
public
class
Startup
{
public
Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public
IConfiguration Configuration {
get
; }
// This method gets called by the runtime. Use this method to add services to the container.
public
void
ConfigureServices(IServiceCollection services)
{
...
services
.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
...
.AddJwtBearer(options =>
{
options.SaveToken =
true
;
options.TokenValidationParameters =
new
TokenValidationParameters()
{
ValidIssuer =
"me"
,
ValidAudience =
"you"
,
IssuerSigningKey =
new
SymmetricSecurityKey(Encoding.UTF8.GetBytes(
"roundthecode9999"
)),
ClockSkew = TimeSpan.Zero
};
});
services.AddAuthorization(options =>
{
options.DefaultPolicy =
new
AuthorizationPolicyBuilder(JwtBearerDefaults.AuthenticationScheme).RequireAuthenticatedUser().Build();
...
});
}
...
}
How Do I Use It In ASP.NET Core?
Watch our video where we go ahead, set it up and show an example of how to use it in an ASP.NET Core application.
We will test the Bearer token and to make sure that it authenticates properly.
What About Extra Security Around Generating a Token?
Security is very important when generating a token. If someone is able to generate a token when they are not supposed to, then it will mean that there is a security vulnerability.
We will have a look at the OAuth guidelines and implement an additional security check when creating a token. In addition, we will do some more checks to ensure that the user trying to create a token is authenticated.
That's coming up in part 3, which is available on 29th December.
Want More ASP.NET Core Coding Tutorials?
Subscribe to my YouTube channel to get more ASP.NET Core coding tutorials.
You'll get videos where I share my screen and implement a how-to guide on a topic related to ASP.NET Core.
You can expect to see videos from the following technologies:
- Blazor
- Web APIs
- SQL Server
- Entity Framework
- SignalR
- and many more...
By subscribing, you can get access to all my ASP.NET Core coding tutorials completely free!
And so you never miss out on a new video, you have the option to be notified every time a new video is published.
So what are you waiting for?
To subscribe, click here to view my YouTube channel, and click on the red "Subscribe" button.
Sign up for the Round The Code Newsletter
Coming soon, we will sending out a newsletter with the latest updates.
Just simply fill out your email below.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK