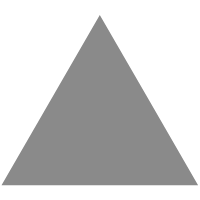
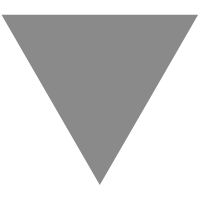
Generate combinations of substrings from a string
source link: https://www.codesd.com/item/generate-combinations-of-substrings-from-a-string.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Generate combinations of substrings from a string
I'm trying to is generate all possible syllable combinations for a given word. The process for identifying what's a syllable isn't relevant here, but it's the generating of all combinations that's giving me a problem. I think this is probably possible to do recursively in a few lines I think (though any other way is fine), but I'm having trouble getting it working. Can anyone help ?
// how to test a syllable, just for the purpose of this example
bool IsSyllable(string possibleSyllable)
{
return Regex.IsMatch(possibleSyllable, "^(mis|und|un|der|er|stand)$");
}
List<string> BreakIntoSyllables(string word)
{
// the code here is what I'm trying to write
// if 'word' is "misunderstand" , I'd like this to return
// => {"mis","und","er","stand"},{ "mis","un","der","stand"}
// and for any other combinations to be not included
}
Try starting with this:
var word = "misunderstand";
Func<string, bool> isSyllable =
t => Regex.IsMatch(t, "^(mis|und|un|der|er|stand)$");
var query =
from i in Enumerable.Range(0, word.Length)
from l in Enumerable.Range(1, word.Length - i)
let part = word.Substring(i, l)
where isSyllable(part)
select part;
This returns:

Does that help to begin with at least?
EDIT: I thought a bit further about this problem an came up with this couple of queries:
Func<string, IEnumerable<string[]>> splitter = null;
splitter =
t =>
from n in Enumerable.Range(1, t.Length - 1)
let s = t.Substring(0, n)
let e = t.Substring(n)
from g in (new [] { new [] { e } }).Concat(splitter(e))
select (new [] { s }).Concat(g).ToArray();
var query =
from split in (new [] { new [] { word } }).Concat(splitter(word))
where split.All(part => isSyllable(part))
select split;
Now query
return this:

Let me know if that's nailed it now.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK