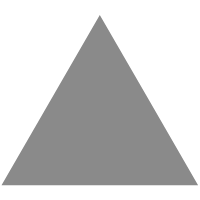
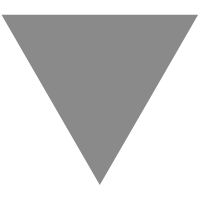
Singleton Pattern – Vorbrodt's C++ Blog
source link: https://vorbrodt.blog/2020/07/10/singleton-pattern/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Singleton Pattern
First things first: if you’re still reading this blog, thanks! I haven’t posted anything since last December; it has been a rough first half of the year: COVID-19, working from home, isolation, you know the deal, but I have not given up on blogging, just finding the time for it has been nearly impossible. I have a long list of topics I eventually want to cover but I can’t make any promises until this mad situation normalizes…
Alright then! A friend from work asked me about the Singleton Design Pattern and how to best implement it in C++. I have done it in the past but was not happy with that implementation; it insisted that the singleton class have a default constructor for example, so I started coding something that would allow non-default initialization. The first thing I came up with was a singleton template base class using the Curiously Recurring Template Pattern. It worked but it required you to declare its constructor private and declare the singleton base class a friend:
This approach allowed me to separate the singleton creation S::Create(17); from usage S::Instance()->foo(); but I was still bothered by the need for private constructor(s) and friendship, so I kept experimenting… I wanted a simpler solution, one that by the very nature of inheriting the singleton base class would automatically render the class non-instantiable by anyone other than the parent template:
The name of the singleton base class probably gave away the approach, but let me explain anyway: the abstract_singleton<AS> base injects a pure virtual method, preventing one from creating instances of class AS. The parent class later erases the abstraction by implementing the private pure virtual method before creating an instance of AS (it actually creates an instance of a private type derived from AS, the compiler takes care of the rest; this works because in C++ access and visibility of a member are two distinct concepts):
One can of course easily defeat the mechanism by which instance creation is restricted by implementing the void abstract_singleton() override {} in the class meant to be a singleton. I don’t think there is much that can be done about that, but if it’s not done on purpose the compiler will detect attempts of creating instances and will fail with cannot instantiate an abstract class error.
Here’s the example program (singleton.cpp):
And the complete listing (singleton.hpp):
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK