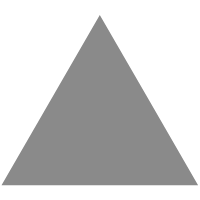
8
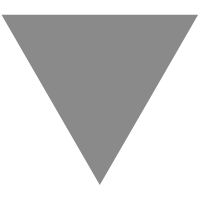
LeetCode题解:pow(x, n)
source link: https://blog.csdn.net/jbj6568839z/article/details/111485868
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
pow(x, n)
实现 pow(x, n) ,即计算 x 的 n 次幂函数。
示例 1:
输入: 2.00000, 10
输出: 1024.00000
示例 2:
输入: 2.00000, -2
输出: 0.25000
解释: 2-2 = 1/22 = 1/4 = 0.25
解题思路
- 要判断 n 的正负,以确定我们的底是 x 还是 1/x
- 经过分析
x^9 = x^4 * x^4 * x = (x^2 * x^2) * (x^2 * x^2) * x
- 判断 n 的奇偶性,已确定是否需要单独考虑
- 如果是奇数,那么需要多乘一次 x 本身,因为 Math.floor 向下取整,9 / 2=> 4, 4 + 4 = 8,少了 1 个
- 如果是偶数,那么不需要考虑,直接降半即可
/**
* @param {number} x
* @param {number} n
* @return {number}
*/
var myPow = function(x, n) {
// 分析n
if (n === 0) return 1;
if (n === 1) return x;
if (n < 0) {
x = 1 / x;
n = -n;
}
let res = 1;
// x^7 = x^1 * x^2 * x^4
// x^9 = x^4 * x^4 * x = (x^2 * x^2) * (x^2 * x^2) * x
while (n > 0) {
// if(m是奇数,m的个位是1),就多乘1次x,因为我们是做向下取整
// &表示 2进制数字的相与
// 如果是奇数,拿x^9举例,那么第一次res = 1 * x,最后一次是1,res = x * x ^ 8
// 如果偶数,拿x^8举例,那么第一次res = 1 * x ^ 8
if ((n & 1) === 1) res *= x;
n = Math.floor(n / 2);
x *= x; // x = x^2 底就要平方,每降一半,自己就要平方一次
}
return res;
};
LeetCode 结果
执行用时:76 ms, 在所有 JavaScript 提交中击败了95.38%的用户
内存消耗:39.6 MB, 在所有 JavaScript 提交中击败了5.03%的用户
三、写在最后
本文是附加题,可能仅仅借助了二分思想中的一部分,加油!
如果对你有所帮助不妨给本项目的github 点个 star,这是对我最大的鼓励
关于我
- 花名:余光
- WX:j565017805
- 沉迷 JS,水平有限,虚心学习中
其他沉淀
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK