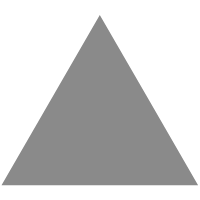
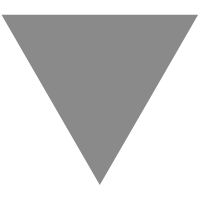
Mastering String Methods in Python
source link: https://towardsdatascience.com/mastering-string-methods-in-python-456174ede911
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
1. Introduction to Strings
A string is a Python data type that’s used to represent a piece of text. It’s written between quotes, either double quotes or single quotes and can be as short as zero characters, or empty string, or as long as you wish.
Strings can be concatenated to build longer strings using the plus sign and also they can be multiplied by a number, which results in the continuous repetition of the string as many times as the number indicates. Also, if we want to find out the length of the string, we simply have to use the len() function as shown in the example below:

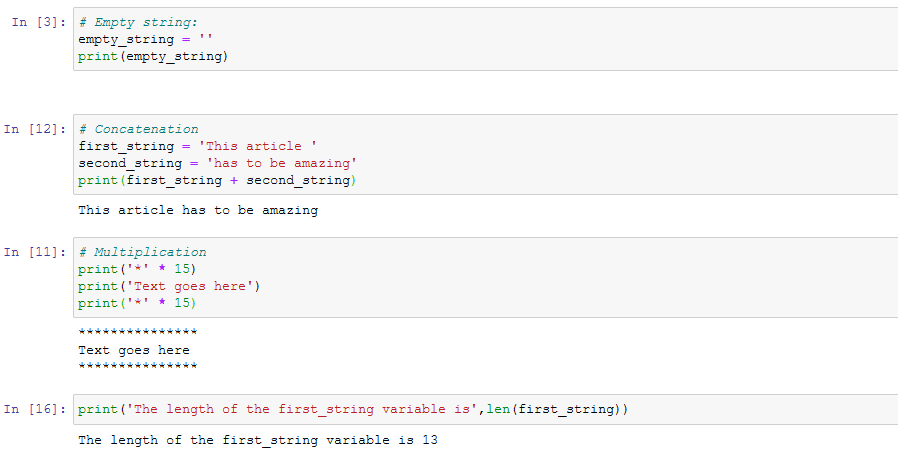
There are tons of things we could do with strings in our scripts. For example, we can check if files are named a certain way by looking at the filename and seeing if they match our criteria, or we can create a list of emails by checking out the users of our system and concatenating our domain:
Email-generator functionIn addition, we can access just a specific character or a slice of characters of a string. We might want to do this, for example, if we have a text that’s too long to display and we want to show just a portion of it. Or if we want to make an acronym by taking the first letter of each word in a phrase. We can do that through an operation called string indexing. This operation lets us access the character in a given position or index using square brackets and the number of the position we want, as the example below shows:

What if you want to print the last character of a string but you don’t know how long it is? You can do that using negative indexes. In the example above, we don’t know the length of the string, but we know that the word ‘text’ plus the exclamation sign take five indices, so we call negative five to access them.
Remember that Python starts counting indexes from 0 not 1. Just like it does with the range function, it considers the range of values between the first and one less than last number.
2. Modifying strings:
Apart from trying to access certain characters inside a string, we might want to change them in case they’re incorrect and we want to fix it, or in case we want to communicate a different thing.
Slicing won’t be useful for this, as strings are immutable data types, in terms of Python, which means that they can’t be modified. What we can do is create a new string based on the old one:

We’re not changing the underlying string that was assigned to it before. We’re assigning a whole new string with different content. In this case, it was pretty easy to find the index to change as there are few characters in the string. How are we supposed to know which character to change if the string is larger?

To achieve our goal, we’ll use the index method which is a function associated with a specific class and serves for a certain function when attached to a variable following a dot.
The index method in particular, returns the index of the given substring, inside the string.The substring that we pass, can be as long or as short as we want. And what happens if the string doesn’t have the substring we’re looking for? The index method can’t return a number because the substring isn’t there, so we get a value error instead:

In order to avoid this Traceback Error, we can use the keyword in to check if a substring is contained in a string. This keyword is also used for for loops, as I explain in this previous article of Loops in Python.
In the case of Loops, it was used for iteration, whereas in this case it’s a conditional that can be either true or false. It’ll be true if the substring is part of the string, and false if it’s not.

3. Transforming Strings
There are a bunch of fun methods for transforming our string text. Among those that are more important to understand to make real-world applications we can find the lower(), upper(), strip(), count() and join() methods.
The strip() method is useful when dealing with user input as it gets rid of surrounding spaces in the string. This means it doesn’t just remove spaces, it also removes tabs and new line characters, which are all characters we don’t usually want in user-provided strings.
There are two more versions of this method, lstrip and rstrip, which eliminate white space characters to the left or to the right of the string respectively, instead of both sides.

Other methods give you information about the string itself. The method count returns how many times a given substring appears within a string. The method endswith returns whether the string ends with a certain substring, whereas the method startswith returns whether the string started with a substring:

Another form of concatenation is with the application of the join method. To use the join method, we have to call it on the string that’ll be used for joining. In this case, we’re using a string with a space in it. The method receives a list of strings and returns one string with each of the strings joined by the initial string. Let’s check its functionality with one simple example:

Lastly, an important application of strings is the split method, which returns a list of all the words in the initial string and it automatically splits by any white space. It can optionally take a parameter and split the strings by another character, like a comma or a dot:

4. Formatting strings
String objects have a built-in functionality to make format changes, which also include an additional way of concatenating strings. Let’s take a look at it:

In the example above, I generated a new string based on the input values of two already-created variables by using the curly brackets placeholder to show where the variables should be written. Then, I passed the variables as a parameter to the format method.
On the other hand, we might want to format the numerical output of a float variable. For example, in the case a product with taxes:

In this case between the curly brackets we’re writing a formatting expression. These expressions are needed when we want to tell Python to format our values in a way that’s different from the default. The expression starts with a colon to separate it from the field name that we saw before. After the colon, we write “.2f”. This means we’re going to format a float number and that there should be two digits after the decimal dot. So no matter what the price is, our function always prints two decimals.
You can also specify text alignment using the greater than operator: >. For example, the expression {:>3.2f} would align the text three spaces to the right, as well as specify a float number with two decimal places.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK