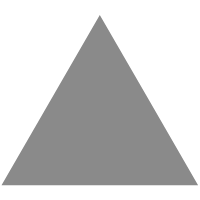
8
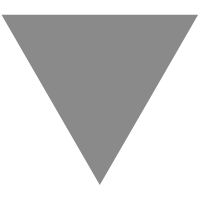
Jim~ - 博客园
source link: https://www.cnblogs.com/jimmypony/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Jim~ - 博客园

代理模式----为另一个对象提供一个替身活占位符以控制对这个对象的访问
head first书上是用JAVA RMI来做的例子,我这边用销售房子 房子--房产商--中介--买家为例说明什么是代理模式
Code
#region 接口
/// <summary>
/// 购房者
/// </summary>
public interface ICustomer
{
//找中介
void FindProxy(IProxy proxy);
//看房
void LookHouse();
//买房
void BuyHouse(string id,int money);
}
/// <summary>
/// 房产中介
/// </summary>
public interface IProxy
{
//代理房产商
void SetLandAgent(ILandAgent landAgent);
//提供房产信息
void ShowHouse();
void SellHouse(string id,int money);
}
/// <summary>
/// 房产商
/// </summary>
public interface ILandAgent
{
//添加可售房源
void AddHouse(IHouse house);
//显示房产信息
void ShowInfo();
void SoldHouse(string id,int money);
}
/// <summary>
/// 房子
/// </summary>
public interface IHouse
{
//房子信息
void GetInfo();
string Property { get; set; }
}
#endregion
/*
* 在这里房子的代理是房产商
* 房产商的代理是中介
* 买家直接找中介买房子,来改变房子的拥有权
*
*/
#region 类
public class House : IHouse
{
private string info;
private string property;
public House(string info,string id)
{
this.info = info;
Property = id;
}
#region IHouse Members
public void GetInfo()
{
Console.WriteLine(string.Format("房产信息:{0},房产ID:{1}", info,Property));
}
public string Property
{
get{ return property; }
set{ property = value; }
}
#endregion
}
public class LandAgent : ILandAgent
{
private List<IHouse> houses;
public LandAgent()
{
houses = new List<IHouse>();
}
#region ILandAgent Members
public void AddHouse(IHouse house)
{
houses.Add(house);
}
public void ShowInfo()
{
foreach (IHouse house in houses)
{
house.GetInfo();
}
}
public void SoldHouse(string id, int money)
{
IHouse soldHouse=new House("","");
foreach (IHouse house in houses)
{
if (house.Property == id)
{
soldHouse = house;
break;
}
}
if (soldHouse.Property != "")
{
houses.Remove(soldHouse);
Console.WriteLine(string.Format("我卖出了房子{0}通过中介获得了购房款{1}", id, money));
soldHouse.Property = "已售出";
soldHouse.GetInfo();
}
else
Console.WriteLine("没有找到这房子 @_@");
}
#endregion
}
public class Proxy : IProxy
{
ILandAgent landAgent;
#region IProxy Members
public void SetLandAgent(ILandAgent landAgent)
{
if (landAgent != null)
this.landAgent = landAgent;
}
public void ShowHouse()
{
if (landAgent != null)
landAgent.ShowInfo();
else
Console.WriteLine("我还没获得代理房产商的代理权呢");
}
public void SellHouse(string id, int money)
{
Console.WriteLine(string.Format("我卖出了房子:{0},获得了5%的中介费:{1}", id, money / 20));
landAgent.SoldHouse(id, money / 20 * 19);
}
#endregion
}
public class Customer : ICustomer
{
private IProxy proxy;
#region ICustomer Members
public void FindProxy(IProxy proxy)
{
if (proxy != null)
this.proxy = proxy;
}
public void LookHouse()
{
proxy.ShowHouse();
}
public void BuyHouse(string id,int money)
{
proxy.SellHouse(id, money);
}
#endregion
}
#endregion
class Program
{
static void Main(string[] args)
{
ICustomer customer = new Customer();
IProxy zhongyuandichan = new Proxy();
ILandAgent wanke = new LandAgent();
IHouse jinsewanli = new House("万科金色万里1楼101室,230平米超大私人空间,售价200万", "001");
IHouse huayuanxiaocheng = new House("万科花园小城13楼602室,104平米二室,售价120万", "002");
IHouse wankejinse = new House("万科金色万里6楼603室,98平米一室户,售价98万", "003");
IHouse wankeyangguang = new House("万科阳光10楼1008室,156平米三室两厅,售价132万", "004");
//房子通过房产商来改变拥有属性
wanke.AddHouse(jinsewanli);
wanke.AddHouse(huayuanxiaocheng);
wanke.AddHouse(wankejinse);
wanke.AddHouse(wankeyangguang);
//房子卖不出去了,房产商只好通过销售外包的方式销售房子
zhongyuandichan.SetLandAgent(wanke);
//顾客找到了中介
customer.FindProxy(zhongyuandichan);
//顾客寻求房产信息
customer.LookHouse();
//顾客看中了房子,通过中介对房子的拥有权进行了修改
customer.BuyHouse("003", 980000);
//顾客再次看房
customer.LookHouse();
Console.ReadKey();
}
}

Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK