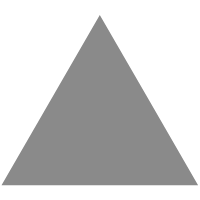
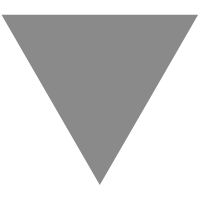
Errors and Error Handling in JavaScript
source link: https://blog.bitsrc.io/errors-and-error-handling-in-javascript-52d448b8183d
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Errors and Error Handling in JavaScript
Why handle them in the first place?
What Are Errors?
Things do not always work the way you want. When something goes wrong, we get notified via an error. This error can either be thrown by the JavaScript engine or a developer.
Any developer should be quite familiar with the concept of errors. Front end developers would definitely be familiar with the alarming red text notifying errors on their browser consoles when running their web applications.
The terms error and exception are used often in the programming world. But more often than not, they are used interchangeably. Although subjective, I consider errors to be thrown by the JavaScript engine while exceptions are thrown by developers. This might not be the case in languages such as Java where errors belong to the unchecked type and occur at runtime while exceptions can occur either in runtime or compile time.
You can read more about errors and exceptions in Java over here.
Types of Errors in JavaScript
Being a dynamically typed language, JavaScript does not warn of type errors before runtime. Although errors are commonly categorized under the Error
object in JavaScript, they can be further subdivided. These sub-divisions inherit from the Error
object.
EvalError
Represents an error which is thrown during the use of the global function eval()
InternalError
Represents an error that is thrown by the JavaScript engine due to an internal error. A perfect example of this would be “too many recursions” or situations where something is too large.
RangeError
Represents an error that is thrown when a numerical value is out of its range.
ReferenceError
Represents an error that is thrown when a reference to a variable cannot be de-referenced. In other words, the referenced variable cannot be found or is inaccessible.
SyntaxError
Represents an error that is thrown when the JavaScript engine could not interpret the given code due to invalid syntax. This error is quite common amongst newbies.
Type Error
Represents an error that is thrown when an operation could not be performed typically due to an invalid data type being used in the operation.
URIError
Represents an error which is thrown when the methods encodeURI()
or decodeURI()
receive invalid parameters.
You can read more about these 7 types of errors in this awesome article below.
Tip: Share your reusable components between projects using Bit (Github).
Bit makes it simple to share, document, and reuse independent components between projects. Use it to maximize code reuse, keep a consistent design, collaborate as a team, speed delivery, and build apps that scale.
Bit supports Node, React Native, React, Vue, Angular, and more.
Example: React components shared on Bit.devWhat is Error Handling?
Generally, programs contain two main types of problems — programmer mistakes and genuine problems. A programmer mistake is where you do something stupid as a programmer in your code such as forgetting to use the correct variable. Genuine problems are which you do not have control over, such as an instance where your database connection fails due to a poor internet connection.
The first category of problems should be handled by finding and fixing them. But the latter can only be fixed by preventive measures or rather proactive measures. This means that you write your code in such a way that it checks for any worst-case possibilities and handles them appropriately. This can be a common action defined to be performed whenever an error occurs of any nature or specific actions to be performed for specific error types.
This concept of proactive measures is called Error Handling.
Why Handle Errors in the First Place?
In order to understand why error handling is essential, we should imagine a simple scenario of what would happen if you don’t handle errors appropriately.
Let’s imagine a scenario where a web application has been deployed with no error handling implemented. As mentioned in the section above, there can be many instances beyond the control of a developer where errors can occur. Let’s take an example when a user tries to upload a file of incorrect format. If this situation was not proactively handled,
- The file upload process will fail
- The user will have no clue as to why the failure happened(unless they are geeks and open the browser console to have a look)
- The user will most likely refrain from visiting your website in the future due to the above issue.
- You as a developer will not have a clue that your program failed at this particular instance
If appropriate error handling measures are taken, the above chain of issues can be stopped from occurring. By the term “appropriate” I refer to a suitable remedy when an error occurs. One of the common issues existing in web applications is that programmers do handle errors but not “appropriately”. I have seen numerous websites that show a general error message whenever an error occurs. Although this should be the final remedy in your bag, most inexperienced developers choose this approach first. The ideal solution would be to handle each error, with a suitable remedy rather than putting a band-aid on a bullet wound. In an actual scenario, you might not be able to think of all the possible scenarios in a specific user journey.
But sometimes, notifying your user on what the specific error is, might not be a good idea as it might sound too complex for an average user. In situations like these, you can provide an error code for the customer to pass on to the customer care team, who can refer to the error code and guide the user accordingly.
Moreover, error handling is essential for the concept of error logging as you cannot log errors if you do not handle the errors in the first place. Error logging is a practice that I personally believe, should be an area of expertise of every developer.
Ways of Error Handling
There are several ways to handle errors in JavaScript. Some concern asynchronous tasks while the other concern synchronous.
try-catch
The try-catch approach runs a block of code statements and “catches” any errors thrown by the code block. If an error occurs in the middle of the code statement, the code execution flow will stop and move onto the statements in the catch block. This enables you to handle these errors appropriately. The try-catch block can be used to handle both synchronous and asynchronous code.
try {
console.log(unknownVariable);
} catch (error) {
console.log(error);
//ReferenceError: unknownVariable is not defined
}
Promise catch
The introduction of Promises revamped JavaScript on the whole. When an error is thrown by a Promise, we can use the catch
method to handle that error. This is similar to the try-catch approach shown earlier, except there is no try block in this approach.
Callback error
In classic Node APIs, a callback is used to handle responses and errors. The usual pattern of the callback would be callback(err,res)
where only “err” or “res” is a non-null value.
As you can see above, the err
parameter will receive an error if the asynchronous function fails and this error should be handled appropriately.
Error event
This is another event handling pattern commonly found in NodeJS where an error
event will be emitted in case of an error.
Since port 80 is the default port for HTTP, the above code snippet will fail to create a server and hence an error will be thrown.
Onerror
HTML elements have several events such as onclick
, onchange
, etc. The onerror
event will be triggered when DOM element throws an error. Furthermore, an onerror
event will be fired by the window
object whenever a JavaScript runtime error occurs.
window.onerror = function(message, source, lineno, colno, error) {
//handle error
};element.error = function(event) {
//handle element error
}
Error boundaries — React
Error boundaries in React allow JavaScript errors to be handled in the component tree without showing a broken UI to the user. These React components can be created by simply defining a new lifecycle method called componentDidCatch(error, info)
You can learn more about error boundaries over here.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK