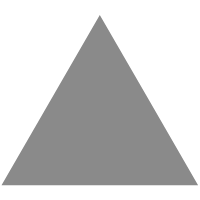
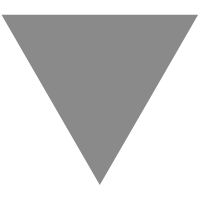
Generate random numbers, characters and slice elements
source link: https://yourbasic.org/golang/generate-number-random-range/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Generate random numbers, characters and slice elements
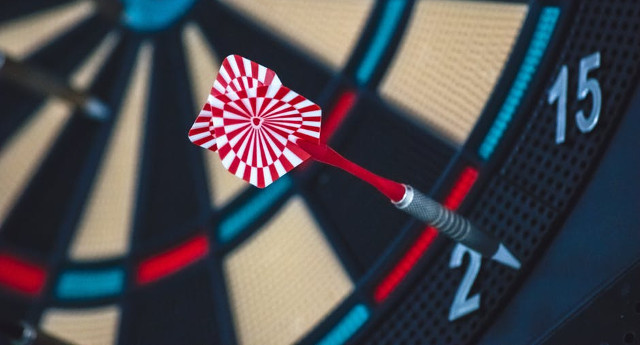
Go pseudo-random number basics
Use the rand.Seed
and
rand.Int63
functions in package
math/rand
to generate a non-negative pseudo-random number of type int64
:
rand.Seed(time.Now().UnixNano())
n := rand.Int63() // for example 4601851300195147788
Similarly, rand.Float64
generates a pseudo-random float x, where 0 ≤ x < 1:
x := rand.Float64() // for example 0.49893371771268225
Warning: Without an initial call to
rand.Seed
, you will get the same sequence of numbers each time you run the program.
See What’s a seed in a random number generator? for an explanation of pseuodo-random number generators.
Several random sources
The functions in the math/rand
package all use a single random source.
If needed, you can create a new random generator of type Rand
with its own source, and then use its methods to generate random numbers:
generator := rand.New(rand.NewSource(time.Now().UnixNano()))
n := generator.Int63()
x := generator.Float64()
Integers and characters in a given range
Number between a and b
Use rand.Intn(m)
,
which returns a pseudo-random number n, where 0 ≤ n < m.
n := a + rand.Intn(b-a+1) // a ≤ n ≤ b
Character between 'a' and 'z'
c := 'a' + rune(rand.Intn('z'-'a'+1)) // 'a' ≤ c ≤ 'z'
Random element from slice
To generate a character from an arbitrary set, choose a random index from a slice of characters:
chars := []rune("AB⌘")
c := chars[rand.Intn(len(chars))] // for example '⌘'
Further reading
Share:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK