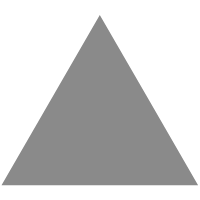
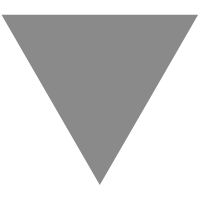
API with NestJS #21. An introduction to CQRS
source link: https://wanago.io/2020/12/07/api-nestjs-introduction-cqrs/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.

So far, in our application, we’ve been following a pattern of controllers using services to access and modify the data. While it is a very valid approach, there are other possibilities to look into.
NestJS suggests command-query responsibility segregation (CQRS). In this article, we look into this concept and implement it into our application.
Instead of keeping our logic in services, with CQRS, we use commands to update data and queries to read it. Therefore, we have a separation between performing actions and extracting data. While this might not be beneficial for simple CRUD applications, CQRS might make it easier to incorporate a complex business logic.
Doing the above forces us to avoid mixing domain logic and infrastructural operations. Therefore, it works well with Domain-Driven Design.
Domain-Driven Design is a very broad topic and it will be covered separately
Implementing CQRS with NestJS
The very first thing to do is to install a new package. It includes all of the utilities we need in this article.
Let’s explore CQRS by creating a new module in our application that we’ve been working on in this series. This time, we add a comments module.
comment.entity.tscreateComment.dto.tsIf you want to know more on creating entities with relationships, check out API with NestJS #7. Creating relationships with Postgres and TypeORM
We tackle the topic of validating DTO classes in API with NestJS #4. Error handling and data validation
Executing commands
With CQRS, we perform actions by executing commands. We first need to define them.
createComment.command.tsTo execute the above command, we need to use a command bus. Although the official documentation suggests that we can create services, we can execute commands straight in our controllers. In fact, this is what the creator of NestJS does during his talk at JS Kongress.
comments.controller.tsAbove, we use the fact that the user that creates the comment is authenticated. We tackle this issue in API with NestJS #3. Authenticating users with bcrypt, Passport, JWT, and cookies
Once we execute a certain command, it gets picked up by a matching command handler.
createComment.handler.tsIn this handler we use a repository provided by TypeORM. If you want to explore this concept more, check out API with NestJS #2. Setting up a PostgreSQL database with TypeORM
The CreateCommentHandler invokes the execute method as soon as the CreateCommentCommand is executed. It does so, thanks to the fact that we’ve used the @CommandHandler(CreateCommentCommand) decorator.
We need to put all of the above in a module. Please notice that we also import the CqrsModule here.
comments.module.tsDoing all of that gives us a fully functional controller that can add comments through executing commands. Once we execute the commands, the command handler reacts to it and performs the logic that creates a comment.
Querying data
Another important aspect of CQRS is querying data. The official documentation does not provide an example, but a Github repository can be used as such.
Let’s start by defining our query. Just as with commands, queries can also carry some additional data.
getComments.query.tsTo execute a query, we need an instance of the QueryBus. It acts in a very similar way to the CommandBus.
comments.controller.tsWhen we execute the query, the query handler picks it up.
getComments.handler.tsAbove, we’ve also created the GetCommentsDto that can transform the postId from a string to a number. If you want to know more about serialization, look into API with NestJS #5. Serializing the response with interceptors
As soon as we execute the GetCommentsQuery, the GetCommentsHandler calls the execute method to get our data.
Summary
This article introduced the concept of CQRS and implemented a straightforward example within our NestJS application. There are still more topics to cover when it comes to CQRS, such as events and sagas. Other patterns also work very well with CQRS, such as Event Sourcing. All of the above deserve separate articles, though.
Knowing the basics of CQRS, we know have yet another tool to consider when designing our architecture.
Series Navigation<< API with NestJS #20. Communicating with microservices using the gRPC frameworkRecommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK