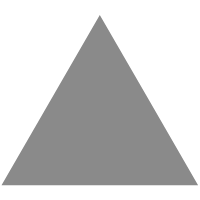
9
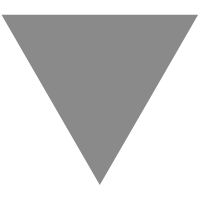
Array won’t change
source link: https://yourbasic.org/golang/gotcha-function-doesnt-change-array/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Array won’t change
yourbasic.org/golang
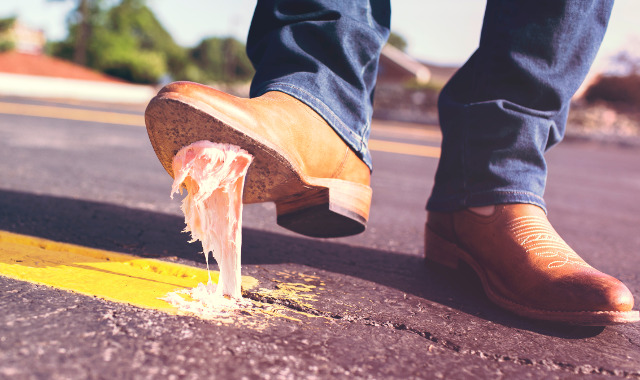
Why does the array value stick?
func Foo(a [2]int) {
a[0] = 8
}
func main() {
a := [2]int{1, 2}
Foo(a) // Try to change a[0].
fmt.Println(a) // Output: [1 2]
}
Answer
- Arrays in Go are values.
- When you pass an array to a function, the array is copied.
If you want Foo
to update the elements of a
, use a slice instead.
func Foo(a []int) {
if len(a) > 0 {
a[0] = 8
}
}
func main() {
a := []int{1, 2}
Foo(a) // Change a[0].
fmt.Println(a) // Output: [8 2]
}
A slice does not store any data, it just describes a section of an underlying array.
When you change an element of a slice, you modify the corresponding element of its underlying array, and other slices that share the same underlying array will see the change.
See Slices and arrays in 6 easy steps for all about slices in Go.
Share:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK