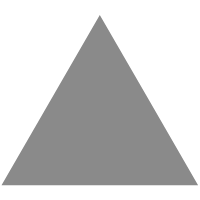
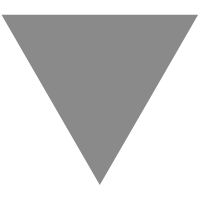
Android How to Integrate Emojis Keyboard in your App
source link: https://www.androidhive.info/2016/11/android-integrate-emojis-keyboard-app/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Emojis are amazing way to express our feeling and deliver our thoughts that people with deferent languages can understand it even children’s can understand it. Any popular social network application provide you a set of Emojis. Take a look at your last messages to see how much you are using them. It’s very important to have them in your applications. Android OS can render Emojis on Text fields.
This article explains how to integrate emojis keyboard into your app easily with many customization options using SuperNova-Emoji library.
1. Simple Integration
Below is the syntax of a simple integration for Emojis Keyboard. The EmojiIconActions constructer accept four parameters, Context, RootView, EmojiconEditText, and ImageView. 
Usually passing the parent layout as RootView is the best option to show the Emojis Keyboard above all views.
 The EmojiconEditText is a EditText with more custom attributes to enable emojis rendering. 
And the last parameter the ImageView will used to switch between the normal keyboard and the emojis keyboard.
To display emojis in TextView we will use EmojiconTextView which is also a TextView with more custom attributes to enable emojis rendering


EmojIconActions emojIcon=
new
EmojIconActions(
this
, rootView, emojiconEditText,
emojiImageView);

emojIcon.ShowEmojIcon();


If you want to use it in xml layout, we will use EmojiconEditText instead of the normal EditText.
<
hani.momanii.supernova_emoji_library.Helper.EmojiconEditText
android:id
=
"@+id/emojicon_edit_text"
android:layout_width
=
"match_parent"
android:layout_height
=
"wrap_content"
emojicon:emojiconSize
=
"28sp"
/>
And EmojiconTextView instead of TextView
<
hani.momanii.supernova_emoji_library.Helper.EmojiconTextView
android:id
=
"@+id/emojicon_text_view"
android:layout_width
=
"wrap_content"
android:layout_height
=
"wrap_content"
emojicon:emojiconSize
=
"28sp"
/>


2. Change the default Toggle Icon
For switching between normal keyboard and emojis keyboard, 
you can call setIconsIds() method which takes two parameters, keyboard Icon ID and smiley Icon ID.
emojIcon.setIconsIds(R.drawable.ic_action_keyboard,R.drawable.smiley);
3. Using device default Emojis
SuperNove-Emoji allow you to use device emojis ins simple way, you need to set the boolean value of setUseSystemEmoji() and setUseSystemDefault() methods to TRUE in every EmojiconTextView and EmojiconEditText you use to display the emojis.
emojIcon.setUseSystemEmoji(
true
);
textView.setUseSystemDefault(
true
);

emojiconEditText.setUseSystemDefault(
true
);


Xml attribute:
emojicon:emojiconUseSystemDefault="true"

4. Changing Emojis Size
In order to change Emojis size, 
you have to change the text size by setting the value of setEmojiconSize() method.
textView.setEmojiconSize(
30
);
XML code
emojicon:emojiconSize="28sp"
5. Detect When the Keyboard Opened or closed
SuperNova-Emoji let you detect when the user open the keyboard or close it to take some actions if needed like show some views when the keyboard open and hide it when the keyboard closed. Use the below code block to achieve this.
emojIcon.setKeyboardListener(
new
EmojIconActions.KeyboardListener() {
@Override
public
void
onKeyboardOpen() {
Log.i(
"Keyboard"
,
"open"
);
}
@Override
public
void
onKeyboardClose() {
Log.i(
"Keyboard"
,
"close"
);
}
});
6. Changing the Emoji Keyboard colors to match your app theme
You can set three colors to the emojis keyboard by adding three parameters to the constructer which they are pressed tabs icons color, tabs color, and background color. 
We will use the same above constructer with the colors value.

EmojIconActions emojIcon=
new
EmojIconActions(
this
, rootView, emojiconEditText, emojiImageView,
"#F44336"
,
"#e8e8e8"
,
"#f4f4f4"
);

emojIcon.ShowEmojIcon();

7. Creating Sample App
Now we’ll create a simple app integrating the emojis to get a good understanding of it in a real app.
1. In Android Studio, go to File ⇒ New Project and fill all the details required to create a new project.
2. Open build.gradle and add supernova emoji library. You need to add its maven repository too.
repositories {
maven { url "https://dl.bintray.com/hani-momanii/maven"}
}
dependencies {
.
.
.
// Supernova Emoji
compile 'hani.momanii.supernova_emoji_library:supernova-emoji-library:0.0.2'
}
3. Open the layout file your main activity activity_main.xml and add below code. Here you can see that I have added the emojiconEditText, emojiconTextView and the ImageView.
<?
xml
version
=
"1.0"
encoding
=
"utf-8"
?>
android:id
=
"@+id/root_view"
android:layout_width
=
"match_parent"
android:layout_height
=
"match_parent"
android:paddingBottom
=
"@dimen/activity_vertical_margin"
android:paddingLeft
=
"@dimen/activity_horizontal_margin"
android:paddingRight
=
"@dimen/activity_horizontal_margin"
android:paddingTop
=
"@dimen/activity_vertical_margin"
tools:context
=
"info.androidhive.emojis.MainActivity"
>
<
ImageView
android:id
=
"@+id/emoji_btn"
android:layout_width
=
"40dp"
android:layout_height
=
"40dp"
android:layout_alignParentBottom
=
"true"
android:layout_alignParentLeft
=
"true"
android:padding
=
"4dp"
android:src
=
"@drawable/ic_insert_emoticon_black_24dp"
/>
<
ImageView
android:id
=
"@+id/submit_btn"
android:layout_width
=
"40dp"
android:layout_height
=
"40dp"
android:layout_alignParentBottom
=
"true"
android:layout_alignParentRight
=
"true"
android:padding
=
"4dp"
android:src
=
"@android:drawable/ic_menu_send"
/>
<
hani.momanii.supernova_emoji_library.Helper.EmojiconEditText
android:id
=
"@+id/emojicon_edit_text"
android:layout_width
=
"match_parent"
android:layout_height
=
"wrap_content"
android:layout_alignParentBottom
=
"true"
android:layout_toLeftOf
=
"@id/submit_btn"
android:layout_toRightOf
=
"@id/emoji_btn"
emojicon:emojiconSize
=
"28sp"
/>
<
CheckBox
android:id
=
"@+id/use_system_default"
android:layout_width
=
"wrap_content"
android:layout_height
=
"wrap_content"
android:layout_below
=
"@+id/textView"
android:layout_centerHorizontal
=
"true"
android:checked
=
"false"
android:text
=
"Use System Default?"
/>
<
hani.momanii.supernova_emoji_library.Helper.EmojiconTextView
android:id
=
"@+id/textView"
android:layout_width
=
"wrap_content"
android:layout_height
=
"wrap_content"
android:layout_centerHorizontal
=
"true"
android:layout_centerVertical
=
"true"
android:layout_marginTop
=
"26dp"
android:text
=
"Hello Emojis!"
android:textAppearance
=
"@style/TextAppearance.AppCompat.Large"
android:textColor
=
"#000000"
emojicon:emojiconSize
=
"45sp"
emojicon:emojiconUseSystemDefault
=
"true"
/>
</
RelativeLayout
>
4. Now open MainActivity.java and make the changes as mentioned below. This activity show the different scenarios of implementing the SuperNova-Emoji as explained above.
package
info.androidhive.emojis;
import
android.os.Bundle;
import
android.support.v7.app.AppCompatActivity;
import
android.util.Log;
import
android.view.View;
import
android.widget.CheckBox;
import
android.widget.CompoundButton;
import
android.widget.ImageView;
import
hani.momanii.supernova_emoji_library.Actions.EmojIconActions;
import
hani.momanii.supernova_emoji_library.Helper.EmojiconEditText;
import
hani.momanii.supernova_emoji_library.Helper.EmojiconTextView;
public
class
MainActivity
extends
AppCompatActivity {
private
static
final
String TAG = MainActivity.
class
.getSimpleName();
CheckBox mCheckBox;
EmojiconEditText emojiconEditText;
EmojiconTextView textView;
ImageView emojiImageView;
ImageView submitButton;
View rootView;
EmojIconActions emojIcon;
@Override
protected
void
onCreate(Bundle savedInstanceState) {
super
.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
rootView = findViewById(R.id.root_view);
emojiImageView = (ImageView) findViewById(R.id.emoji_btn);
submitButton = (ImageView) findViewById(R.id.submit_btn);
mCheckBox = (CheckBox) findViewById(R.id.use_system_default);
emojiconEditText = (EmojiconEditText) findViewById(R.id.emojicon_edit_text);
textView = (EmojiconTextView) findViewById(R.id.textView);
emojIcon =
new
EmojIconActions(
this
, rootView, emojiconEditText, emojiImageView);
emojIcon.ShowEmojIcon();
emojIcon.setIconsIds(R.drawable.ic_action_keyboard, R.drawable.smiley);
emojIcon.setKeyboardListener(
new
EmojIconActions.KeyboardListener() {
@Override
public
void
onKeyboardOpen() {
Log.e(TAG,
"Keyboard opened!"
);
}
@Override
public
void
onKeyboardClose() {
Log.e(TAG,
"Keyboard closed"
);
}
});
mCheckBox.setOnCheckedChangeListener(
new
CompoundButton.OnCheckedChangeListener() {
@Override
public
void
onCheckedChanged(CompoundButton compoundButton,
boolean
b) {
emojIcon.setUseSystemEmoji(b);
textView.setUseSystemDefault(b);
}
});
submitButton.setOnClickListener(
new
View.OnClickListener() {
@Override
public
void
onClick(View v) {
String newText = emojiconEditText.getText().toString();
textView.setText(newText);
}
});
}
}
Run And test the app.
Hani is passionate about coding, Android Development, contributing to Opensource communities and actively competing in hackathons. focusing on making Android Developer life easier.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK