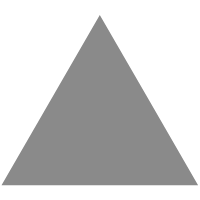
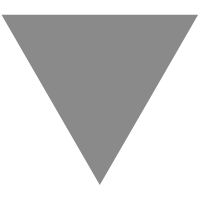
Creating a strictly typed collection of objects in PHP
source link: https://odan.github.io/2019/08/30/creating-a-strictly-typed-collection-of-objects-in-php.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Daniel's Dev Blog
Developer, Trainer, Open Source Contributor
Blog About me DonateCreating a strictly typed collection of objects in PHP
30 Aug 2019
Table of contents
Intro
Generics are still a long way off and whether they’ll ever come like that is questionable.
Did you know that we actually do not need generics, because PHP 7.4+ has everything to implement type-safe and future-proof collection classes.
We only need the features everyone should know since PHP 7: Classes, arrays and type declarations.
Type declarations were also known as “type hints” in PHP 5. With type declarations, methods can require that parameters be of a certain type at the time of the invocation. If the specified value is of the wrong type, an error occurred.
Creating a data object
First, we create a data object to store our data in-memory:
<?php
final class User
{
/**
* @var int
*/
public int $id;
/**
* @var string
*/
public string $username;
}
Note: Typed class properties have been added in PHP 7.4 and provide a major improvement to PHP’s type system.
Creating a collection class
Now we create a collection class to collect and retrieve our “array” of user objects.
Example:
final class UserList
{
/**
* @var User[] The users
*/
private $users = [];
/**
* Add user to list.
*
* @param User $user The user
*
* @return void
*/
public function addUser(User $user): void
{
$this->users[] = $user;
}
/**
* Get all users.
*
* @return User[] The users
*/
public function all(): array
{
return $this->users;
}
}
Usage
Let’s just create a new (and empty) collection object:
$users = new UserList();
Then we add some new User objects to the collection:
$user = new User();
$user->id = 1;
$user->username = 'admin';
$users->addUser($user);
$user = new User();
$user->id = 2;
$user->username = 'operator';
$users->addUser($user);
Now we can iterate over the collection with the method $users->all()
:
foreach ($users->all() as $user) {
echo sprintf("ID: %s, Username: %s\n", $user->id, $user->username);
}
© 2020 Daniel Opitz | Twitter
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK