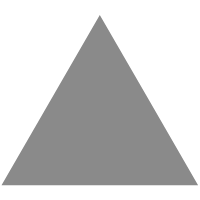
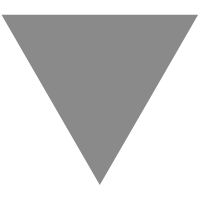
如何在 Asp.Net Core 中发送 Email
source link: https://mp.weixin.qq.com/s?__biz=MjM5MjQwMDUzMw%3D%3D&%3Bmid=2247484161&%3Bidx=1&%3Bsn=7328bd1052fb5bfd6038abcc8a2fb634
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
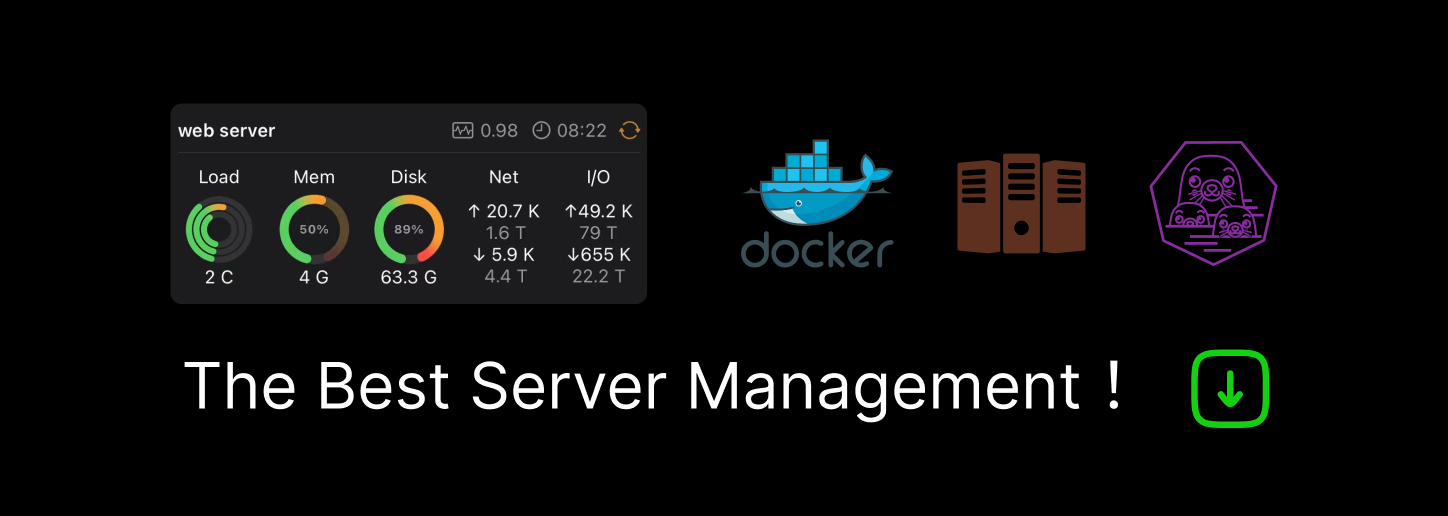
在项目开发中常常会需要做发送 Email 的功能,在 ASP.NET Core 中你可以用 MailKit
来实现 Email 的发送, MailKit
是一个开源的客户端库,可用在 Windows,Linux 或者 Mac 上,本篇文章就来讨论在 ASP.NET Core 中去实现。
安装 MailKit
要想使用 MailKit,你可以使用 Visual Studio 2019 中的 NuGet package manager
可视化界面进行安装,或者通过 NuGet package manager console
命令行输入如下命令:
Install-Package NETCore.MailKit
安装完成之后,在代码中引入以下命令空间即可。
using MailKit.Net.Smtp;
using MimeKit;
配置 Email 的基础信息
下面的代码片段展示了在 appsettings.json
文件中配置 email 的详细信息。
"NotificationMetadata": {
"Sender": "[email protected]",
"SmtpServer": "smtp.gmail.com",
"Reciever": "[email protected]",
"Port": 465,
"Username": "[email protected]",
"Password": "specify your password here"
}
为了能够实现 configuration 中的NotificationMetadata节点映射,我定义了一个 NotificationMetadata
类,代码如下:
public class NotificationMetadata
{
public string Sender { get; set; }
public string Reciever { get; set; }
public string SmtpServer { get; set; }
public int Port { get; set; }
public string UserName { get; set; }
public string Password { get; set; }
}
接下来在 Startup.ConfigureServices
方法中将 NotificationMetadata 节点映射到 NotificationMetadata 类。
public void ConfigureServices(IServiceCollection services)
{
var notificationMetadata =
Configuration.GetSection("NotificationMetadata").
Get<NotificationMetadata>();
services.AddSingleton(notificationMetadata);
services.AddControllers();
}
生成 EmailMessage 消息类
使用如下代码创建一个 EmailMessage 类。
public class EmailMessage
{
public MailboxAddress Sender { get; set; }
public MailboxAddress Reciever { get; set; }
public string Subject { get; set; }
public string Content { get; set; }
}
生成 MimeMessage 类
下面的代码展示了如何从自定义的 EmailMessage 类中构造出一个 MimeMessage。
private MimeMessage CreateMimeMessageFromEmailMessage(EmailMessage message)
{
var mimeMessage = new MimeMessage();
mimeMessage.From.Add(message.Sender);
mimeMessage.To.Add(message.Reciever);
mimeMessage.Subject = message.Subject;
mimeMessage.Body = new TextPart(MimeKit.Text.TextFormat.Text)
{ Text = message.Content };
return mimeMessage;
}
用 MailKit 同步发送 Email
为了最终能够实现 email 发送,需要使用 MailKit.Net.Smtp
命名空间下的 SmtpClient 类,下面的代码展示了具体实现步骤。
using (SmtpClient smtpClient = new SmtpClient())
{
smtpClient.Connect(_notificationMetadata.SmtpServer,
_notificationMetadata.Port, true);
smtpClient.Authenticate(_notificationMetadata.UserName,
_notificationMetadata.Password);
smtpClient.Send(mimeMessage);
smtpClient.Disconnect(true);
}
为了方便起见,我就把完整的发送 Email 代码放在 DefaultController.Get
方法下。
public string Get()
{
EmailMessage message = new EmailMessage();
message.Sender = new MailboxAddress("Self", _notificationMetadata.Sender);
message.Reciever = new MailboxAddress("Self", _notificationMetadata.Reciever);
message.Subject = "Welcome";
message.Content = "Hello World!";
var mimeMessage = CreateEmailMessage(message);
using (SmtpClient smtpClient = new SmtpClient())
{
smtpClient.Connect(_notificationMetadata.SmtpServer,
_notificationMetadata.Port, true);
smtpClient.Authenticate(_notificationMetadata.UserName,
_notificationMetadata.Password);
smtpClient.Send(mimeMessage);
smtpClient.Disconnect(true);
}
return "Email sent successfully";
}
用 MailKit 异步发送 Email
上面我们用同步的方式发送 Email,这一节来看看如何使用异步的方式发送 Email。
using (SmtpClient smtpClient = new SmtpClient())
{
await smtpClient.ConnectAsync(_notificationMetadata.SmtpServer,
_notificationMetadata.Port, true);
await smtpClient.AuthenticateAsync(_notificationMetadata.UserName,
_notificationMetadata.Password);
await smtpClient.SendAsync(mimeMessage);
await smtpClient.DisconnectAsync(true);
}
最后值得注意的是,MailKit 除了简单的字符串,还支持模板的方式甚至可以带上 附件
发送,更多的 MailKit 特性我会在后面的文章中和大家去讨论。
译文链接:https://www.infoworld.com/article/3534690/how-to-send-emails-in-aspnet-core.html
喜欢就来个三连,让更多人因你而受益
Recommend
-
17
Angular Email Confirmation with ASP.NET Core Identity Posted by Marinko Spasojevic | Updated Date Dec 21, 2020 |
-
17
你可以遵循一些最佳实践来写出更干净的 Controller,一般我们称这种方法写出来的 Controller 为瘦Controller,瘦 Controller 的好处在于拥有更...
-
7
ASP.NET Core 是一个轻量级,模块化的框架,常用来在 Windows,Linux 和 MacOS 上构建高性能,现代化的web框架,不像过去的 Asp.NET,在 ASP.NE...
-
13
ASP.Net Core 是微软开源的跨平台、可扩展、轻量级的模块化框架,可用于构建高性能的web应用程序。中间件组件可以注入到 ASP.Net Core 请求管道中...
-
13
ASP.NET Core 是一个开源的、跨平台的、轻量级模块化框架,可用于构建高性能、可伸缩的web应用程序,你也许不知道 ASP.NET Core 中有一个藏得很深...
-
11
ASP.NET Core 中内置了对依赖注入的支持,可以使用 依赖注入 的方式在运行时实现组件注入,这样可以让代码更加灵活,测试和可维护,通常有三种方式可以实现依赖注入。 构造函数注入 属性注入 方法注入 构造函数 这种注...
-
12
本文转载自微信公众号「码农读书」,作者码农读书 。转载本文请联系码农读书公众号。 记录日志的一个作用就是方便对应用程序进行跟踪和...
-
13
利用IHttpClientFactory可以无缝创建HttpClient实例,避免手动管理它们的生命周期。 当使用ASP.Net Core开发应用程序时,可能经常需要通过HttpClient调用WebAPI的方法以检查终结点是否正常工作。要实现这一点,通常需要实例化HttpClient...
-
7
ASP.NET Core 是一个开源的,跨平台的,精简的模块化框架,可用于构建高性能,可扩展的web应用程序, ASP.NET Core 中的数据配置常用 k-v 的形式存储,值得注意的是,新的数据配置还支持 层级方式 ,在这篇文章中,我们将会...
-
5
在 ASP.NET Core 中处理 Web 应用程序时,我们可能经常希望构建轻量级服务,也就是没有模板或控制器类的服务。轻量级服务可以降低资源消耗,而且能够提高性能。我们可以在 Startup 或 Program 类中创建这些轻量级服务或 API。1. 使用 VS2022 创...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK