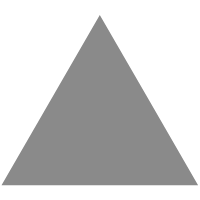
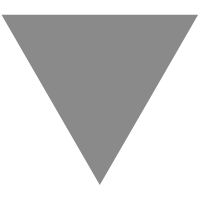
8 Extension Methods to Simplify Coding in 2020
source link: https://www.danylkoweb.com/Blog/8-extension-methods-to-simplify-coding-in-2020-RA
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
8 Extension Methods to Simplify Coding in 2020
How could I not continue the tradition of writing more C# Extension Methods for the C# Advent Calendar this year?
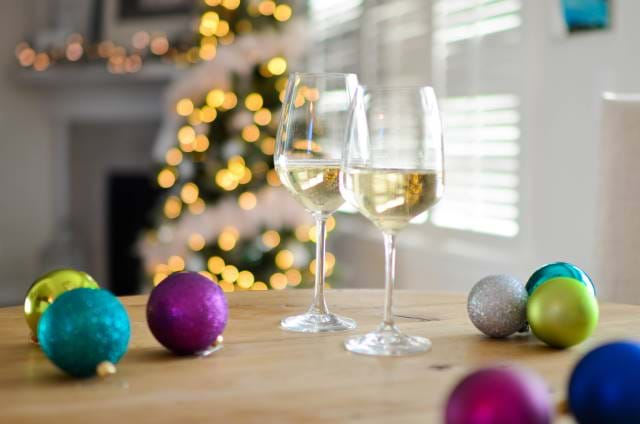
Notice: I usually post on Monday, Wednesday, and/or Friday. However, I selected a date on the Advent Calendar where it fell on a Thursday.
This post was written for the Fourth Annual C# Advent Calendar (#csadvent). A TON of thanks to Matt Groves (@mgroves) for putting this together again! Awesome job, Matt!
With every C# Advent Calendar, I discussed what Extension Methods were, how to use them, how to identify them, and examples of my favorite extensions every year.
Starting with 2014, here are the posts in case you really want more extension methods:
I'm always on the lookout for awesome extension methods. If you have any extensions you'd like to share, post them in the comments below.
Let's dig into this year's list.
GetFlagSum(<array of Enum items>)
With last week's post describing how to add granular authorizations using Identity Claims, this extension was necessary for when you wanted to grant a user all of the permissions and returning a simple integer to store in your UserClaims table. <T> is the Enumerated type.
public static int GetFlagSum(this T[] list)
{
return list.Cast<int>().Sum();
}
Based on the example from the link above, we have enumeration which contains flags.
[Flags]
public enum PermissionEnum
{
Create = 1,
Retrieve = 2,
Update = 4,
Delete = 8,
Upload = 16,
SendEmail = 32
}
If we have an array of enumerations, we can perform a LINQ Sum() to get the total of all the flags.
var list = new[]
{
PermissionEnum.Create,
PermissionEnum.View,
PermissionEnum.Update,
PermissionEnum.Delete
};
var total = list.GetFlagSum<PermissionEnum>(); // total = 15
This simplifies your flag arithmetic.
ToEnum<T>() (for string and int)
This makes things a little easier to convert either a string or an integer into an Enum. <T> is the enumerated type.
// 1 - string
public static T ToEnum<T>(this string value)
{
return (T) System.Enum.Parse(typeof(T), value, true);
}
// 2 - int
public static T ToEnum<T>(this int value)
{
var type = typeof(T);
if (!type.IsEnum)
{
throw new ArgumentException($"{type} is not an enum.");
}
if (!type.IsEnumDefined(value))
{
throw new ArgumentException($"{value} is not a valid ordinal of type {type}.");
}
return (T) System.Enum.ToObject(type, value);
}
Usage:
var updateEnum = 4.ToEnum<PermissionEnum>(); // returns PermissionEnum.Update
var retrieveEnum = "Retrieve".ToEnum<PermissionEnum>(); // returns PermissionEnum.Retrieve
LoadFile()
Ever look for a quick way to load a file?
public static MemoryStream LoadFile(this string file)
{
var ms = new MemoryStream();
using (var fs = File.OpenRead(file))
{
fs.CopyTo(ms);
}
return ms;
}
Usage:
var stream = "C:\\TextFile.txt".LoadFile();
ReadUrl()
How about a quick way to download a file from a URL?
public static MemoryStream ReadUrl(this string url)
{
byte[] result = null;
using (var webClient = new WebClient())
{
result = webClient.DownloadData(url);
}
return new MemoryStream(result);
}
Usage:
var webStream = "https://www.cnn.com/".ReadUrl();
ToCurrency()
Similar to ToInt()
, this creates a quick and simple currency string from a double. Sometimes it's just the little things that make your coding easier.
Enter your cultureName and it returns a formatted currency string.
public static string ToCurrency(this double value, string cultureName)
{
CultureInfo currentCulture = new CultureInfo(cultureName);
return (string.Format(currentCulture, "{0:C}", value));
}
Usage:
var price = 14.99;
var formattedPrice = price.ToCurrency("en-us"); // returns $14.99
Repeat()
The Repeat() extension method takes a string and repeats it X times and returns the result.
public static string Repeat(this string instr, int times)
{
var result = String.Empty;
for (var i = 0; i < times; i++)
result += instr;
return result;
}
Usage:
var batman = "NaN".Repeat(5); // Returns NaNNaNNaNNaNNaN
ToQueryString()
Finally, if you have a NameValueCollection, the ToQueryString() extension method takes said collection and creates a query string for a URL.
public static string ToQueryString(this NameValueCollection nvc)
{
return string.Join("&",
nvc.AllKeys.Select(
key => $"{HttpUtility.UrlEncode(key)}={HttpUtility.UrlEncode(nvc[key])}"));
}
Usage:
var parameters = new NameValueCollection
{
{"pageIndex", "3"},
{"pageSize", "20"}
};
var queryString = parameters.ToQueryString(); // Returns "pageIndex=3&pageSize=20"
Conclusion
Every year, I continue to build on my extension method collection, but lately, it seems I'm noticing more extension methods appearing in the .NET framework. It's great to see these useful and recommended methods appearing in .NET for each type.
We just need to get more creative with our extension methods.
Do you love extension methods? Do you dislike them? Why? Post your comments below and let's discuss.
Did you like this content? Show your support by buying me a coffee.

Jonathan Danylko is a web architect and entrepreneur who's been programming for over 25 years. He's developed websites for small, medium, and Fortune 500 companies since 1996.
He currently works at Insight Enterprises as a Principal Software Engineer.
When asked what he likes to do in his spare time, he replies, "I like to write and I like to code. I also like to write about code."
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK