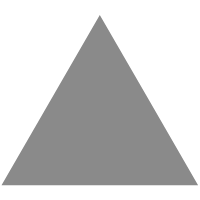
8
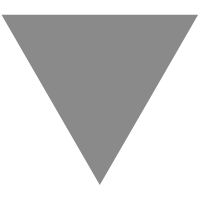
JavaScript前后端JSON使用方法详解
source link: https://www.chenwenguan.com/how-to-use-json-in-javascript/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
汇总整理下JSON在JavaScript前后端中的使用方法,包括字符串和JSON对象的互转,JSON数组的遍历,JSON对象key值的获取,JSON内容格式化输出到文件,读取JSON内容文件转化为JSON对象等。
一、JavaScript后端JSON操作方法
1、JavaScript JSON字符串转JSON对象
var testStr = '{"name":"will","age":18,"hobby":"football"}' var jsonObj = JSON.parse(testStr)
2、JS JSON对象转字符串
var testObj = { "name": 'will', "age": '18', "hobby": 'football' } var jsonStr = JSON.stringify(testObj)
3、JavaScript JSON数组的遍历
一种是for循环遍历:
for (var l = 0; l < jsonArray.length; l++) { var jsonItem = jsonArray[l] }
一种是键值遍历:
var testJSArray = [ {"number": 'one'}, {"number": "two"}, {"number": "three"}, {"number": "four"}, {"number": "five"}, {"number": "six"}, ] for(index in testJSArray){ console.log("index:" + index + "; name:" + testJSArray[index].number) } 输出内容如下: index:0; number:one index:1; number:two index:2; number:three index:3; number:four index:4; number:five index:5; number:six
4、JS JSON数组合并
数组合并连接用concat方法,前端和后端都是用concat。
var testJSArray01 = [ {"name": 'one'}, {"name": "two"}, {"name": "three"}, ] var testJSArray02 = [ {"name": "four"}, {"name": "five"}, {"name": "six"}, ] var testJSONMerge = testJSArray01.concat(testJSArray02)
5、JavaScript获取JSON对象key值
var testObj = { "name": 'will', "age": '18', "hobby": 'football' } for (var key in testObj){ console.log("key:" + key + ", value:" + testObj[key]) } 输出内容如下: key:name, value:will key:age, value:18 key:hobby, value:football
6. JS格式化输出JSON内容到文件
var writeStream = fs.createWriteStream(filePath);
return new Promise(function(resolve, reject) {
writeStream.write("写入你需要的字符串内容");
// 换行,如果是字符串中包含换行的字符,写入到.txt文件的时候并不能换行,需要输出换行符才行。
writeStream.write("\n");
// 格式化输出JSON字符串内容, JSONObj是要输出的JSON数据对象
writeStream.write(JSON.stringify(JSONObj, null, "\t") + "\n");
writeStream.end();
writeStream.on('finish', () => {
resolve(filePath);
});
});
7、JavaScript读取JSON文件内容
不管内容是保存成.json还是.txt,只要是合法的JSON字符串内容都可以。
var filePath = 'xxx/xxx/test.json' var fileContent = fs.readFileSync(filePath).toString(); var fileJson = JSON.parse(fileContent);
二、JavaScript前端JSON操作方法
1、字符串转JSON,angular.fromJson() 等价于JSON.parse()
var processInfo = angular.fromJson('{"process":[]}');
2、JSON转字符串,angular.toJson() 等价于JSON.stringify()
var out = angular.toJson(jsonObj, true);
3、JSON数组遍历
angular.forEach(jsonArray, function(jsonItem) { });
4、判断JSON对象是否含有某个Key值
比如判断jsonObj这个对象是否含有“samples”这个key值
jsonObj.hasOwnProperty("samples")
扩展阅读:
AngularJS集成HighCharts动态绘制CPU和内存变化曲线
转载请注明出处:陈文管的博客– JavaScript前后端JSON使用方法详解
扫码或搜索: 文呓
微信公众号 扫一扫关注
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK