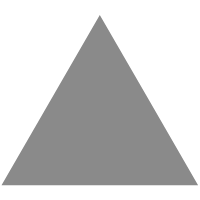
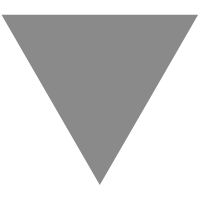
万能Python的秘诀:操纵数据的内置工具
source link: http://developer.51cto.com/art/202010/630434.htm
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Python可谓是如今最流行的编程语言,甚至孩子们也可以从它开始学习趣味编程。Python类似英语的简单语法使它成为一种通用语言,已在全世界各个领域被广泛使用。
Python的万能之处正在于其内置的数据结构,它使编码变得简单,不受数据类型限制,并可以根据需要操纵数据。
首先,让我们试着理解什么是数据结构?数据结构是能够存储、组织和管理数据的结构/容器,以便能够有效地访问和使用数据。数据结构就是收集数据类型。Python中有四种内置数据结构。它们是:
· 列表
· 字典
· 元组
· 集合
开发人员最常用的数据结构是列表和字典。接下来,让我们详细看看每一个数据结构。
列表
Python列表是按顺序排列的任意类型的项的集合。一个列表可以有重复的项,因为每个项都是使用索引访问的,而且可以通过使用负索引逆向访问该列项。列表是可变的,这意味着即使在创建了项之后,也可以添加、删除或更改项;一个列表中还可以包含另一个列表。
图源:unsplash
创建列表:
列表可以通过将元素括在[ ]方括号中来创建,每个项之间用逗号分隔。以购物清单为例,创建列表的语法是:
#Creating a list fruits = ['Apple', 'Banana', "Orange"]
print(type(fruits)) #returns type
print(fruits) #prints the elements of the listOutput:
['Apple', 'Banana', 'Orange']
访问列表:
可以使用索引访问列表中的项。列表中的每个项都有一个与之关联的索引,具体取决于该项在列表中的位置。访问列表中的项的语法:
#Access elements in the fruits listfruits = ['Apple', 'Banana',"Orange"]
print(fruits[0]) #index 0 is the first element
print(fruits[1])
print(fruits[2])Output:
Apple
Banana
Orange
但是,索引不必总是为正。如果想逆向访问列表,也就是按照相反的顺序,可以使用负索引,如下所示:
#Access elements in the fruits list using negative indexesfruits = ['Apple','Banana', "Orange"]
print(fruits[-1]) #index -1 is the last element
print(fruits[-2])
print(fruits[-3])Output:
Orange
Banana
Apple
如果必须返回列表中两个位置之间的元素,则使用切片。必须指定起始索引和结束索引来从列表中获取元素的范围。语法是List_name[起始:结束:步长]。在这里,步长是增量值,默认为1。
#Accessing range of elements using slicingfruits = ['Apple', 'Banana',"Orange"]
fruits #all elements
['Apple', 'Guava', 'Banana', 'Kiwi'] #output
fruits[::1] #start to end with step 1
['Apple', 'Guava', 'Banana', 'Kiwi'] #outputfruits[::2] #start to endwith step 2 basically index 0 & 2
['Apple', 'Banana'] #output
fruits[::3] #start to end with step 2 basically index 0 & 3
['Apple', 'Kiwi'] #output
fruits[::-1] #start to end with step 2 - reverse order
['Kiwi', 'Banana', 'Guava', 'Apple'] #output
向列表中添加元素:
可以使用append()、extend()和insert()函数向列表添加项。
#Adding elementsfruits = ['Apple', 'Banana', "Orange"]#Appendnew elements
fruits.append('Kiwi')
print(fruits)
Output:
['Apple', 'Banana', 'Orange', 'Kiwi']#Insertelements in to the listfruits.insert(1,'Guava') #inserts Guava as secondelement is the list since the index is specified as 1
print(fruits)
Output:
['Apple', 'Guava', 'Banana','Orange', 'Kiwi']
从列表中删除项:
与添加元素类似,从列表中删除元素也非常容易,可以使用del()、remove()和pop()方法实现。要清除整个列表,可以使用clear()函数。
· del()函数删除给定索引处的元素。
· pop()函数从列表中删除给定索引处的元素,也可以将删除的元素赋值给变量。如果未指定索引值,则删除列表中的最后一个元素。
· remove()函数根据元素的值来删除元素。
· clear()函数清空列表。
#Deleting elements from the listfruits = ['Apple', 'Guava', 'Banana','Orange', 'Kiwi']
#del() function
del fruits[3] #delete element at index 4
print(fruits)
Output:
['Apple', 'Guava', 'Banana', 'Kiwi']#pop()function
del_fruit = fruits.pop(2)
print(del_fruit)
print(fruits)
Output:
'Banana'
['Apple', 'Guava', 'Orange', 'Kiwi']
#Remove function
fruits.remove('Apple')
print(fruits)
Output:
['Guava', 'Banana', 'Orange', 'Kiwi']
#Clear() function
fruits.clear()
print(fruits)
Output :
[] # clears the list
其他函数:
在处理列表时,还可以使用其他几个函数:
· len()函数返回列表的长度。
· index()函数查找第一次遇到的传入值的索引值。
· count()函数查找传递给它的值的个数。
· sorted()和sort()函数用于对列表的值进行排序。sorted()具有返回类型,而sort()修改原始列表。
#Other functions for listnum_list = [1, 2, 3, 10, 20, 10]
print(len(num_list)) #find length of list
print(num_list.index(10)) #find index of element that occurs first
print(num_list.count(10)) #find count of the element
print(sorted(num_list)) #print sorted list but not change original
num_list.sort(reverse=True) #sort original list
print(num_list)Output:
6
3
2
[1, 2, 3, 10, 10, 20]
[20, 10, 10, 3, 2, 1]
字典
字典是另一种无序的数据结构,即元素的存储顺序与它们被插入的顺序不同。这是因为索引值不能访问字典中的元素。在字典中,数据以键值对的形式存储,元素值是通过键访问的。
图源:unsplash
创建字典:
字典由冒号分隔的{}大括号或使用dict()函数编写键和值被创建。
#Creating Dictionariesnew_dict = {} #empty dictionary
print(new_dict)
new_dict = {1: 'Python', 2: 'Java'} #dictionary with elements
print(new_dict)Output:
{}
{1: 'Python', 2: 'Java'}
改变并增加键值对:
要更改字典的值,将使用键来访问键,然后相应地更改值。要添加值,只需添加另一个键-值对,如下所示:
#Changing and Adding key, value pairslang_dict = {'First': 'Python','Second': 'Java'}
print(lang_dict)
lang_dict['Second'] = 'C++' #changing element
print(lang_dict)
lang_dict['Third'] = 'Ruby' #adding key-value pair
print(lang_dict)Output:
{'First': 'Python', 'Second': 'Java'}
{'First': 'Python', 'Second': 'C++'}
{'First': 'Python', 'Second': 'C++','Third': 'Ruby'}
访问字典中的元素:
字典中的元素只能使用键访问,可以使用get()函数或只是通过键来获取值。
#Accessing Elementslang_dict = {'First': 'Python', 'Second': 'Java'}
print(lang_dict['First']) #access elements using keys
print(lang_dict.get('Second'))Output:
Python
Java
删除字典中的键值对:
这些是字典中用于删除元素的函数。
· pop()-删除值并返回已删除的值
· popitem()-获取键值对并返回键和值的元组
· clear()-清除整个字典
#Deleting key, value pairs in a dictionarylang_dict = {'First': 'Python','Second': 'Java', 'Third': 'Ruby'}
a = lang_dict.pop('Third') #pop element
print('Value:', a)
print('Dictionary:', lang_dict)
b = lang_dict.popitem() #pop the key-value pair
print('Key, value pair:', b)
print('Dictionary', lang_dict)
lang_dict.clear() #empty dictionary
print(lang_dict)Output:
Value: Ruby #pop element
Dictionary: {'First': 'Python','Second': 'Java'}
Key, value pair: ('Second', 'Java') #popthe key-value pair
Dictionary {'First': 'Python'}
{} #empty dictionary
其他函数:
这是其他一些可以与字典一起使用的函数,用于获取键值和键-值对等。
#Other functions for dictionarylang_dict = {'First': 'Python','Second': 'Java', 'Third': 'Ruby'}
print(lang_dict.keys()) #get keys
print(lang_dict.values()) #get values
print(lang_dict.items()) #get key-value pairs
print(lang_dict.get('First'))Output:
dict_keys(['First', 'Second','Third'])
dict_values(['Python', 'Java','Ruby'])
dict_items([('First', 'Python'),('Second', 'Java'), ('Third', 'Ruby')])
Python
元组
图源:unsplash
元组与列表基本相同,不同的是,一旦数据进入元组,无论如何都不能更改。因此,一旦生成元组,就不能添加、删除或编辑任何值。
创建元组:
使用()圆括号或tuple()函数创建元组。
#Creating Tuplesmy_tuple = (1, 2, 3) #create tupleprint(my_tuple)Output: (1, 2, 3)#Creating Tuplesmy_tuple = (1, 2, 3) #create tuple
print(my_tuple)Output:
(1, 2, 3)
访问元组中的元素:
访问元组元素与列表类似。
#access elementsmy_tuple2 = (1, 2, 3,'new') for x in my_tuple2:
print(x) # prints all the elementsin my_tuple2print(my_tuple2)
print(my_tuple2[0]) #1st element
print(my_tuple2[:]) #all elements
print(my_tuple2[3][1]) #this returns the 2nd character of the element atindex 3
print(my_tuple2[-1]) #last elementOutput:
1
2
3
new
(1, 2, 3, 'new')
1
(1, 2, 3, 'new')
e
new
在另一元组中追加元素:
要追加值,可以使用'+'操作符。
#Appending elementsmy_tuple = (1, 2, 3)
my_tuple = my_tuple + (4, 5, 6) #add elements
print(my_tuple)Output:
(1, 2, 3, 4, 5, 6)
元组赋值:
元组打包和解包操作很有用,执行这些操作可以在一行中将另一个元组的元素赋值给当前元组。元组打包就是通过添加单个值来创建元组,元组拆包则是将元组中的值分配给变量。
#tuple packing
planets = ('Earth','Mars','Jupiter')
#tuple unpackinga,b,c = planets
print(a)
print(b)
print(c)Output:
Earth
Mars
Jupiter
集合
图源:unsplash
集合是唯一的无序元素的集合。这意味着,即使数据重复一次以上,集合也只保留一次。
创建集合:
使用{ }花括号创建集合,并赋值。
#Creating setsnew_set = {1, 2, 3, 4, 4, 4, 5} #create set
print(new_set)Output:
{1, 2, 3, 4, 5}
向集合中添加元素:
使用add()函数赋值并添加元素。
#Adding elements to a Setnew_set = {1, 2, 3}
new_set.add(4) #add element to set
print(new_set)Output:
{1, 2, 3, 4}
集合操作:
可以对一个集合执行的不同操作如下所示。
· union()函数合并了两个集合中的数据。
· intersection()函数只查找在这两个集合中同时出现的数据。
· difference()函数删除两个集合中同时存在的数据,并只输出在传递的集合中存在的数据。
· symmetric_difference()函数执行与difference()函数相同的操作,但是输出在两个集合中保留的数据。
· clear()函数清空该集合。
#Operations on set
my_set = {1, 2, 3, 4}
my_set_2 = {3, 4, 5, 6}
print(my_set.union(my_set_2))
print(my_set.intersection(my_set_2))
print(my_set.difference(my_set_2))
print(my_set.symmetric_difference(my_set_2))
my_set.clear()
print(my_set)Output:
{1, 2, 3, 4, 5, 6}
{3, 4}
{1, 2}
{1, 2, 5, 6}
set()
Python为我们有效管理、组织和访问数据提供了多种选项,学习其基本内置数据结构是Python学习之旅非常关键的一环。
【责任编辑:赵宁宁 TEL:(010)68476606】
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK